5: Loading and Saving Regions
Demonstrate importing / exporting of regions
@author: Guihong Wan and Boshen Yan
@date: Oct 13, 2023
[1]:
import anndata as ad
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import seaborn as sns
import spatialcells as spc
Read Anndata and saved region/shapes files
Load epithelial region file from omero csv format and tumor cell region from spatialcell outputs.
The workflow for generating and saving the tumor cell region can be found at the end of this notebook.
[2]:
# Load data
adata = ad.read("../../data/Z147_1_750.h5ad")
[3]:
# note that you may want to scale the coordinates to convert from pixels to microns
pixels2micron = 0.6
adata.obs.X_centroid = adata.obs.X_centroid*pixels2micron
adata.obs.Y_centroid = adata.obs.Y_centroid*pixels2micron
[4]:
adata.var_names
[4]:
Index(['HHLA2', 'CMA1', 'SOX10', 'S100B', 'KERATIN', 'CD1A', 'CD163', 'CD3D',
'C8A', 'MITF', 'FOXP3', 'PDL1', 'KI67', 'LAG3', 'TIM3', 'PCNA',
'pSTAT1', 'cPARP', 'SNAIL', 'aSMA', 'HLADPB1', 'S100A', 'CD11C', 'PD1',
'LDH', 'PANCK', 'CCNA2', 'CCND1', 'CD63', 'CD31'],
dtype='object')
[5]:
# The expression is scaled. So, the gate is 0.5
spc.prep.setGate(adata, "SOX10", 0.5)
SOX10_positive
False 586292
True 524293
Name: count, dtype: int64
[7]:
# Load regions
# note that you may want to scale the coordinates to convert from pixels to microns
epi = spc.utils.importRegion("../data/tutorial5_Z147_1.ome.tif-rois.csv", scale=pixels2micron)
tumor = spc.utils.importRegion("../data/tutorial5_boundary.csv") # see the end of this file for example
[8]:
# assign cells to regions
adata.obs["tumor_region"] = "non-Tumor"
spc.spatial.assignPointsToRegions(
adata, [tumor], ["Tumor"], assigncolumn="tumor_region", default="non-Tumor"
)
adata.obs["epi_region"] = "non-Epi"
spc.spatial.assignPointsToRegions(
adata, [epi], ["Epi"], assigncolumn="epi_region", default="non-Epi"
)
723114it [01:37, 7424.25it/s]
Assigned points to region: Tumor
531709it [00:16, 32188.14it/s]
Assigned points to region: Epi
[9]:
# plot
markersize = 1
fig, ax = plt.subplots(figsize=(10,8))
## all points
ax.scatter(
*zip(*adata.obs[["X_centroid", "Y_centroid"]].to_numpy()),
s=markersize, color="grey", alpha=0.3, label="Other cells"
)
## tumor
tumor_tmp = adata.obs[adata.obs["tumor_region"] == "Tumor"]
ax.scatter(
*zip(*tumor_tmp[["X_centroid", "Y_centroid"]].to_numpy()),
s=markersize, color="red", alpha=0.5, label="Tumor"
)
## epi
epi_tmp = adata.obs[adata.obs["epi_region"] == "Epi"]
ax.scatter(
*zip(*epi_tmp[["X_centroid", "Y_centroid"]].to_numpy()),
s=markersize, color="blue", alpha=0.5, label="Epi"
)
ax.legend(loc="lower right", markerscale=5)
ax.invert_yaxis()
plt.show()
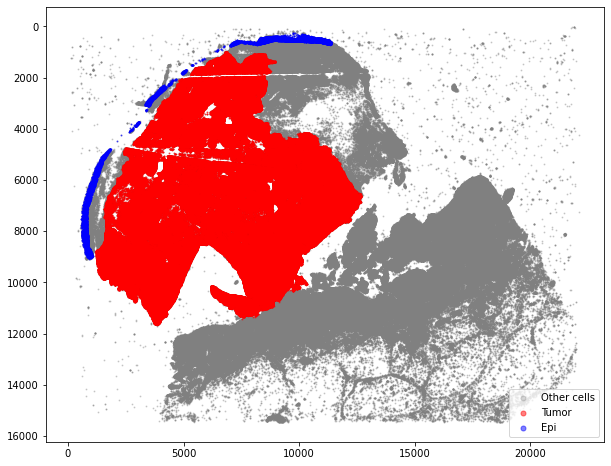
[10]:
print(adata.obs['epi_region'].value_counts())
print(adata.obs['tumor_region'].value_counts())
epi_region
non-Epi 1098026
Epi 12559
Name: count, dtype: int64
tumor_region
Tumor 586796
non-Tumor 523789
Name: count, dtype: int64
[11]:
# Calculate distance from tumor boundary
spc.msmt.getDistanceFromObject(
adata,
epi,
region_col="tumor_region",
region_subset=["Tumor"],
name="distance_from_epidermis",
binned=True,
binsize=1500,
)
586796it [00:10, 56913.73it/s]
[12]:
print(adata.obs['distance_from_epidermis_binned'].value_counts(sort=False))
distance_from_epidermis_binned
[0, 1500) 92232
[1500, 3000) 122678
[3000, 4500) 120291
[4500, 6000) 102554
[6000, 7500) 105681
[7500, 9000) 43095
[9000, 10500) 265
Name: count, dtype: int64
[13]:
adata
[13]:
AnnData object with n_obs × n_vars = 1110585 × 30
obs: 'X_centroid', 'Y_centroid', 'column_centroid', 'row_centroid', 'Area', 'MajorAxisLength', 'MinorAxisLength', 'Eccentricity', 'Solidity', 'Extent', 'Orientation', 'imageid', 'phenotype', 'kmeans', 'kmeans_renamed', 'pickseq_roi', 'pickseq_roi_minimal', 'dermis_roi', 'epidermis_roi', 'general_roi', 'Kmeans_r', 'der_epider_roi', 'phenotype_proliferation', 'phenograph', 'spatial_expression_phenograph', 'spatial_expression_kmeans', 'phenograph_raw', 'phenograph_raw_minimal', 'phenotype_1', 'phenotype_1_tumor_kmeans', 'phenotype_1_tumor_kmeans_renamed', 'phenotype_final', 'spatial_expression_consolidated', 'MC', 'spatial_count_kmeans', 'phenotype_2', 'phenotype_2_tumor_kmeans', 'phenotype_2_tumor_kmeans_renamed', 'phenotype_large_cohort', 'phenotype_large_cohort_RJP', 'spatial_lda_kmeans', 'phenotype_2_tumor_kmeans_old', 'tumor_expression_trimmed', 'tumor_expression_TC', 'IM_roi', 'TC_ROI_IM_large', 'TC_ROI_IM_small', 'TC_ROI_IM_elarge', 'manuscript_roi', 'SOX10_positive', 'tumor_region', 'epi_region', 'distance_from_epidermis', 'distance_from_epidermis_binned'
uns: 'all_markers', "dendrogram_['kmeans']", "dendrogram_['phenograph']", "dendrogram_['phenograph_raw']", "dendrogram_['phenograph_raw_minimal']", "dendrogram_['phenotype']", "dendrogram_['phenotype_2_tumor_kmeans']", "dendrogram_['phenotype_2_tumor_kmeans_scaled']", "dendrogram_['spatial_expression_consolidated']", "dendrogram_['spatial_expression_kmeans']", "dendrogram_['spatial_expression_phenograph']", 'interaction_all', 'rank_genes_groups', 'spatial_count_radius', 'spatial_distance', 'spatial_expression_radius', 'spatial_interaction', 'spatial_interaction_1', 'spatial_interaction_motif_analysis'
[14]:
adata.obs["pheno"] = "Other"
adata.obs.loc[adata.obs["phenotype_proliferation"] == "Proliferating Tumor", "pheno"] = "Proliferating Tumor"
adata.obs.loc[adata.obs["phenotype_proliferation"] == "Proliferating T cells", "pheno"] = "Proliferating T cells"
print(adata.obs['pheno'].value_counts())
pheno
Other 995691
Proliferating Tumor 100498
Proliferating T cells 14396
Name: count, dtype: int64
[15]:
metric_col = "distance_from_epidermis_binned"
pheno = "pheno"
markersize = 0.5
fig, [ax1, ax2] = plt.subplots(1, 2, figsize=(17, 7))
## Plot epidermis points and boundary
epi_tmp = adata.obs[adata.obs["epi_region"] == "Epi"]
ax1.scatter(
*zip(*epi_tmp[["X_centroid", "Y_centroid"]].to_numpy()),
s=markersize, color="red", alpha=0.5
)
spc.plt.plotBoundary(epi, ax=ax1, linewidth=1.0, color="k")
## Plot tumor points by distance from epidermis
sns.scatterplot(
data=adata.obs,
x="X_centroid",
y="Y_centroid",
hue=metric_col,
alpha=0.8,
s=markersize,
ax=ax1,
)
ax1.invert_yaxis()
ax1.legend(loc="upper right", title=metric_col)
## Plot region composition barplots
df = spc.msmt.getRegionComposition(
adata,
[metric_col, pheno],
regions=["Tumor"],
regioncol="tumor_region"
).sort_values(by=metric_col, ascending=False)
sns.barplot(data=df, y=metric_col, x="composition", hue=pheno, log=True, ax=ax2)
ax2.legend(bbox_to_anchor=(1.02, 1), loc="upper left", borderaxespad=0)
plt.tight_layout()
plt.show()
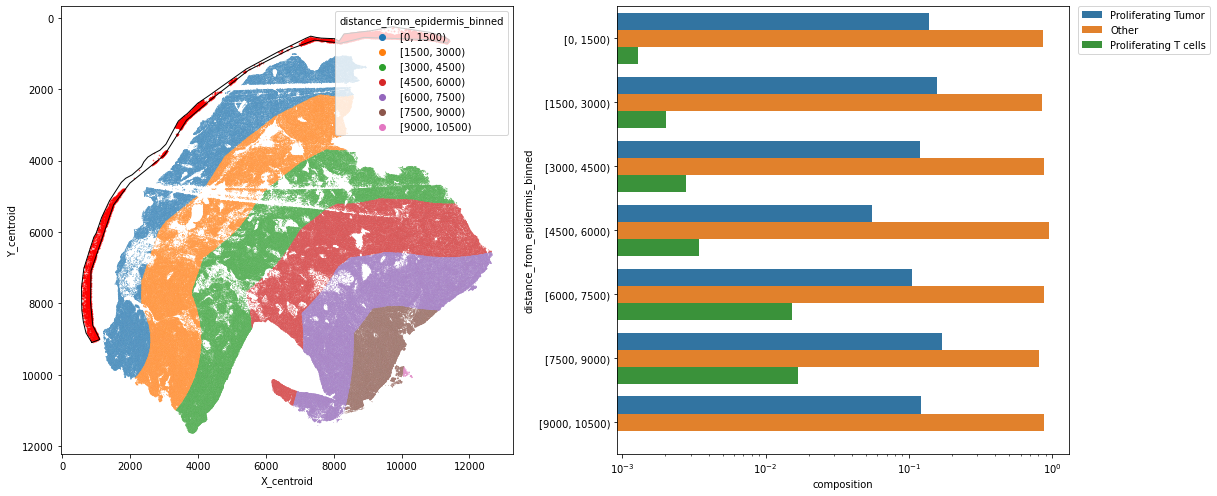
Generate and export tumor region
The following steps compute the tumor cell region based on SOX10+ cells for distance measurement with the epithelial cells. For detailed tutorial, please refer to tutorial 0.
[58]:
markers_of_interest = ["SOX10_positive"]
communitycolumn = "SOX10_community"
ret = spc.spa.getCommunities(
adata,
markers_of_interest,
eps=90, # smaller --> more cells are considered as outliers.
newcolumn=communitycolumn,
)
fig, ax = plt.subplots(figsize=(10, 8))
spc.plt.plotCommunities(
adata, ret, communitycolumn, plot_first_n_clusters=10, s=1, fontsize=10, ax=ax
)
ax.invert_yaxis()
plt.show()
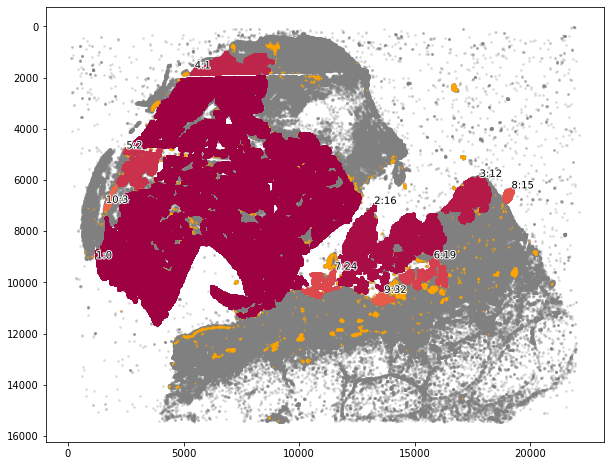
[60]:
clusters_idx_sorted = [idx for npoints, idx in ret[0]]
plot_first_n_clusters = 10
print(
"Indexes of the",
plot_first_n_clusters,
"largest clusters:\n",
clusters_idx_sorted[:plot_first_n_clusters],
)
print("Cluster size and index:\n", ret[0][:plot_first_n_clusters])
Indexes of the 10 largest clusters:
[0, 16, 12, 1, 2, 19, 24, 15, 32, 3]
Cluster size and index:
[(420503, 0), (53646, 16), (13179, 12), (9583, 1), (8328, 2), (6697, 19), (3407, 24), (1586, 15), (995, 32), (557, 3)]
[62]:
# Here we choose the three largest ones of interest in the larger tumor region:
communityIndexList = [0, 1, 2, 3]
boundary = spc.spa.getBoundary(adata, communitycolumn, communityIndexList, alpha=100)
# filter out small regions or holes based on the number of edges
# or the area of the region
boundary_pruned = spc.spa.pruneSmallComponents(
boundary, min_edges=50, holes_min_edges=50, holes_min_area=50000
)
markersize = 1
fig, ax = plt.subplots(figsize=(10, 8))
## all points
ax.scatter(
*zip(*adata.obs[["X_centroid", "Y_centroid"]].to_numpy()),
s=markersize, color="grey", alpha=0.2
)
# Points in selected commnities
xy = adata.obs[adata.obs[communitycolumn].isin(communityIndexList)][
["X_centroid", "Y_centroid"]
].to_numpy()
ax.scatter(xy[:, 0], xy[:, 1], s=markersize, color="r")
# Boundary of selected communities
spc.plt.plotBoundary(boundary_pruned, ax=ax)
ax.invert_yaxis()
plt.show()
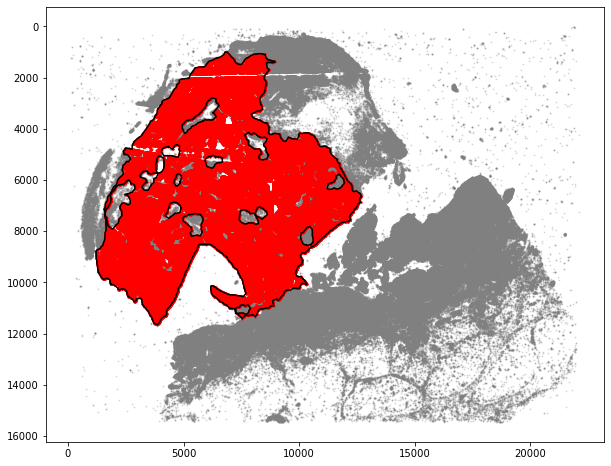
Save the boundary objects generated
Boundary objects can be saved as geojson type files or omero format csv files. Note that currently, saving to csv format ignores the holes inside boundary regions. To preserve information of the holes, save to a json/geojson file instead.
[5]:
spc.utils.exportRegion(boundary_pruned, "../data/tutorial5_boundary.json")
spc.utils.exportRegion(boundary_pruned, "../data/tutorial5_boundary.csv")
[5]:
'{"type":"MultiPolygon","coordinates":[[[[7377.602943319256,11265.546483982396],[7377.687364890276,11265.647648543443],[7398.785844577061,11287.78593094544],[7398.893507692692,11287.883587612476],[7427.174899640779,11310.012875187302],[7427.212336858052,11310.040784298144],[7441.717413094196,11320.336190500699],[7532.018077308936,11421.638825962767],[7532.088179621043,11421.709973009738],[7532.165029633437,11421.77377200699],[7532.2478606722225,11421.829586480644],[7532.335846395371,11421.87685961231],[7532.428109036503,11421.91511979405],[7532.523728161695,11421.943985333244],[7532.621749851944,11421.963168260447],[7532.721196219691,11421.972477202242],[7532.8210751644565,11421.971819290424],[7532.92039027027,11421.961201088478],[7533.018150746154,11421.940728526095],[7533.1133813104825,11421.9106058424],[7533.20513192063,11421.871133548408],[7533.292487250815,11421.822705429058],[7533.3745758236055,11421.765804614719],[7533.450578703992,11421.700998761367],[7533.519737669266,11421.628934387523],[7600.031736007459,11345.004524132573],[7615.072097968323,11335.355979529106],[7615.15694314926,11335.295070316444],[7622.572341275723,11329.361112471011],[7622.578434928411,11329.356196949564],[7628.688747206767,11324.387628007828],[7699.144568001149,11288.307103979532],[7761.074400818556,11287.858441262018],[7797.315869896483,11301.27094993832],[7797.407771284211,11301.30000844251],[7797.5020433566115,11301.320084251265],[7797.5978103014595,11301.330990855071],[7797.694182418771,11301.332626928723],[7797.790264386352,11301.324977272669],[7797.885163577576,11301.308112954215],[7797.977998354119,11301.282190647293],[7798.06790625661,11301.24745117692],[7826.194632629754,11288.790252181205],[7900.509879205953,11278.586359050249],[7906.003449847557,11278.666171721274],[7994.345290314919,11338.006988483643],[7994.424805935447,11338.055192600656],[7994.508455249572,11338.095802489246],[7994.595514994307,11338.128467021259],[7994.685232418818,11338.152903767037],[7994.776831792982,11338.168901437415],[7994.869521114647,11338.176321710605],[8098.9512463258525,11341.651366174812],[8099.049291023081,11341.64982941611],[8099.146713856008,11341.638697663935],[8099.2425780919875,11341.618077951467],[8099.335961984474,11341.588168539805],[8099.425967635738,11341.549257011658],[8099.511729630254,11341.501717506208],[8099.592423355789,11341.446007121705],[8099.667272932141,11341.382661520422],[8099.73555867133,11341.312289778185],[8099.79662399749,11341.235568528045],[8164.773649887649,11250.83495877375],[8226.827375837465,11246.439291544237],[8226.9237843298,11246.427736435156],[8227.018617686155,11246.406885716653],[8227.110981806467,11246.37693597176],[8227.200005870913,11246.338169570206],[8227.284850550113,11246.290952006195],[8227.364715918427,11246.235728452488],[8227.438848995744,11246.173019563266],[8227.506550846665,11246.10341656533],[8227.567183170158,11246.027575683955],[8227.620174317522,11245.946211955912],[8227.665024681975,11245.860092488028],[8227.701311408988,11245.770029224805],[8227.728692383018,11245.676871293306],[8265.036386955135,11091.422387285484],[8395.871123158324,11069.748545741944],[8395.932220557499,11069.736458530519],[8515.553099678378,11042.174467580291],[8515.632548833013,11042.152679241712],[8515.709934923441,11042.12442516451],[8515.784732738017,11042.08989710628],[8521.20100324153,11039.313516525026],[8648.72180421916,11019.563217792796],[8648.816568189386,11019.543806557063],[8648.909013323298,11019.515330192444],[8648.99827461121,11019.47805515245],[8649.0835168347,11019.432330219865],[8666.961343595884,11008.697945638593],[8692.731342621366,11000.22496951892],[8692.739533916632,11000.222237039123],[8773.01645597091,10973.057467104605],[8839.32509955968,10976.055736039276],[8839.42208830936,10976.05541329973],[8839.518589610801,10976.045696276255],[8839.613695683816,10976.026676376126],[8839.706511873019,10975.99853251806],[8839.796165063784,10975.961529449125],[8839.881811895564,10975.916015254294],[8839.962646695316,10975.862418082035],[8840.037909056406,10975.801242116751],[8893.21602588291,10928.11222729548],[8893.288884690102,10928.039800946492],[8893.353928299162,10927.960281698923],[8893.410470241022,10927.87450879747],[8893.457913773056,10927.783387487769],[8893.495758177089,10927.687879462468],[8893.52360404397,10927.588992711531],[8893.541157488928,10927.487770883929],[8893.548233253221,10927.385282272986],[8893.544756659363,10927.28260854164],[8887.223842090061,10853.304976496265],[8887.211779527932,10853.21364536436],[8887.191363269361,10853.123811865895],[8887.162766584712,10853.03623840709],[8887.12623217064,10852.95166821347],[8866.03815691071,10809.72331651796],[8861.230898907177,10783.97209863223],[8860.797442305537,10777.923260642407],[8860.78938234815,10777.849400786119],[8851.020085156206,10711.344429737297],[9035.95105674716,10663.773676039635],[9051.521597496267,10684.11458821552],[9055.270363108046,10690.361953404577],[9055.354723121573,10690.481692294798],[9069.426888070026,10707.634269614386],[9069.493268102646,10707.707481466376],[9069.566550279691,10707.773783853438],[9069.646018906604,10707.832529247631],[9144.628752719555,10757.727493276407],[9144.713432665128,10757.778002211786],[9144.802675084065,10757.819926386172],[9144.895612375334,10757.852858219207],[9144.991341016928,10757.876477552692],[9145.088930349759,10757.890554763111],[9145.187431625389,10757.894952993995],[9145.285887229611,10757.88962948642],[9145.38333999223,10757.874635994709],[9145.47884249252,10757.850118283273],[9145.571466269905,10757.81631470952],[9145.660310850311,10757.773553906583],[9145.744512500434,10757.722251588388],[9145.82325262482,10757.662906508158],[9145.895765724124,10757.596095609611],[9145.961346837186,10757.522468417996],[9146.019358394537,10757.442740725508],[9146.069236416768,10757.357687632479],[9146.11049599744,10757.268136011955],[9146.142736017275,10757.174956470979],[9146.165643043774,10757.079054886672],[9169.802201002516,10630.877290887962],[9185.928946292286,10609.496489150702],[9211.733073189687,10575.927736463174],[9211.737916388778,10575.921383558782],[9235.129840764574,10544.982274339782],[9239.929914947897,10540.171217310583],[9315.919819500501,10488.694587391872],[9316.001169443187,10488.633207907445],[9316.075850026622,10488.563867697314],[9316.143085673959,10488.487286877193],[9316.202178125905,10488.404260758372],[9316.252513692316,10488.315651588207],[9316.29356962554,10488.222379595463],[9316.324919549268,10488.125413433501],[9343.140361093945,10388.018860982327],[9361.0700318981,10367.027235037753],[9375.780423115713,10355.401546781943],[9375.85953489794,10355.33194877822],[9392.214647776122,10339.338497131843],[9400.903809847314,10331.076339390003],[9435.386126175452,10308.180526377622],[9528.494018555173,10298.217721086572],[9617.955984851413,10297.44492079345],[9677.972260825956,10305.388342046954],[9736.855394781202,10347.203862485861],[9736.937469582143,10347.25632833906],[9737.024259677006,10347.300559754758],[9737.114941519902,10347.336137023769],[9737.20865463638,10347.362722555565],[9737.304509788428,10347.380064081664],[9737.401597412378,10347.38799704939],[9737.498996249718,10347.386446183298],[9737.595782088856,10347.37542619946],[9737.691036534892,10347.355041665827],[9737.78385572423,10347.32548600999],[9737.87335890127,10347.287039683755],[9737.958696775853,10347.240067501956],[9738.039059582117,10347.185015180747],[9738.11368476232,10347.122405108226],[9738.181864202701,10347.052831387538],[9836.181406692629,10236.783297879656],[9892.683533228908,10253.607155631633],[9892.78007828,10253.630749425418],[9892.878488513863,10253.64464330418],[9892.97779187219,10253.648700029546],[9893.07700747474,10253.642879530747],[9893.175155308089,10253.627239300426],[9893.271265905825,10253.60193382674],[9893.364389924564,10253.56721306739],[9917.312270088478,10243.255578096081],[9982.455298985098,10234.319653270199],[10003.807059376139,10233.438008701389],[10003.90728309138,10233.428801220327],[10004.00607367512,10233.409566060633],[10004.102430421148,10233.380498066217],[10004.19537727698,10233.341891683387],[10004.283972730858,10233.29413797823],[10004.367319348863,10233.237720675286],[10004.444572865525,10233.17321125762],[10004.514950735875,10233.101263177941],[10004.577740062294,10233.022605239423],[10004.632304815854,10232.938034213219],[10004.678092279022,10232.848406767529],[10017.729195189217,10203.776310975256],[10020.921412326905,10198.702528095844],[10020.956978429072,10198.641289773546],[10103.74689236456,10043.707059636201],[10110.168810524601,10041.26902974884],[10126.859419884024,10034.96607606424],[10251.461353192813,10050.600330889487],[10297.740659135075,10084.083358719106],[10297.819759729924,10084.135075879192],[10297.903389251218,10084.179094874195],[10297.990800756137,10084.215022545433],[10298.081213522813,10084.242538002443],[10298.173820023409,10084.261395489028],[10298.267793136594,10084.271426578249],[10298.362293535049,10084.272541676744],[10298.456477181968,10084.264730824934],[10298.549502869631,10084.248063785979],[10298.640539732714,10084.222689422682],[10298.728774669216,10084.188834367917],[10327.268945400925,10071.66066363621],[10327.357479011831,10071.616453004444],[10327.441209469573,10071.563708424932],[10327.519316820175,10071.50294641375],[10327.591036175525,10071.434761999919],[10327.655665203736,10071.359822898417],[10327.712571006938,10071.278862971396],[10327.761196319107,10071.192675041628],[10327.801064963249,10071.102103128567],[10327.831786514493,10071.00803418305],[10327.85306012344,10070.91138940159],[10327.864677462314,10070.813115205288],[10327.866524765068,10070.714173971752],[10327.85858394147,10070.615534610735],[10327.840932754265,10070.518163075822],[10327.813744057645,10070.423012905052],[10327.777284104544,10070.331015883137],[10327.731909939268,10070.243072916695],[10327.678065901046,10070.160045211866],[10327.616279272708,10070.082745840717],[10321.322517679457,10062.961937246197],[10304.122330793962,10038.293358264167],[10304.10322489119,10038.266888142953],[10287.618426378218,10016.19643154163],[10237.31645998901,9931.938004304042],[10237.249393709351,9931.83951404274],[10227.78024162933,9919.573817293478],[10227.70959698959,9919.491885021478],[10227.630515044104,9919.418063559713],[10139.31666369721,9845.500641345445],[10083.591169847954,9779.563281697961],[10083.528076219496,9779.495290427029],[10083.45895421632,9779.433437637668],[10083.384398537795,9779.378255487703],[10059.714648336345,9763.503606011474],[10059.625967781345,9763.450559102956],[10059.532286396825,9763.406947022835],[10059.434604532778,9763.373235470295],[10059.333965257185,9763.349784424554],[10059.231443217901,9763.336844300926],[10059.12813316734,9763.33455327684],[10059.025138272467,9763.342935816345],[10058.92355833495,9763.361902408875],[10058.824478047234,9763.391250525076],[10035.503318139557,9771.63195339235],[10001.526504093827,9779.783261817407],[9995.97597940748,9780.047335527528],[9912.198461833626,9770.641288599625],[9894.212077804657,9758.573896425723],[9837.728270980862,9630.623264189315],[9857.248290578726,9585.736293902875],[9857.251793301964,9585.728140537078],[9859.8509230488,9579.603298764925],[9859.863263669824,9579.572835387353],[9872.441360012474,9546.994690021418],[9907.17507794486,9483.367645980139],[9907.223251249887,9483.266249281007],[9925.970350561589,9437.315531483562],[9925.974711026696,9437.304662072813],[9933.8807899115,9417.258121289498],[9946.129954329028,9399.033532281608],[9946.181655891392,9398.947593813495],[9946.22448934279,9398.856908824142],[9946.258023844157,9398.762389465961],[9946.28192208965,9398.664986459258],[9950.379559704437,9377.408417071845],[10083.700567000551,9309.276977139805],[10090.33217443268,9306.419736373678],[10090.420261466235,9306.376543769606],[10090.503692201153,9306.324927502808],[10090.581663622743,9306.265384376064],[10090.653425261798,9306.198487487593],[10090.718286417818,9306.12488071503],[10103.017302889912,9290.704002736458],[10103.067137743428,9290.635796328033],[10103.111038361505,9290.563627342259],[10103.14869148572,9290.488010750085],[10111.061133732619,9272.75822230881],[10111.08938927159,9272.68785413292],[10111.112231454781,9272.615547215357],[10134.137456981009,9188.78742325946],[10134.165092242622,9188.649414104002],[10140.474274452092,9139.31508842251],[10140.482004048237,9139.214648626994],[10140.479589285731,9139.113940791638],[10140.467054669343,9139.013986889402],[10140.444527399095,9138.91580124241],[10140.412236079455,9138.820380228708],[10140.370508399477,9138.728692171124],[10140.319767807452,9138.64166751082],[10126.277140114438,9117.126485769333],[10122.720014842671,9111.539410916683],[10122.673236952778,9111.472183106705],[10122.621114553767,9111.409008705732],[10109.39733244602,9096.656092013129],[10088.291886842973,9048.281925955294],[10088.227659024715,9048.158820899944],[10079.19733434939,9033.442002718126],[10079.131201813476,9033.347030171864],[10079.05453944329,9033.260334278955],[10074.25857812189,9028.431202359521],[10047.658275311149,8967.433180922726],[10107.141302481232,8849.874793036284],[10205.922587750218,8853.610180055617],[10232.260758883494,8862.48267352476],[10232.358803543639,8862.510229269297],[10232.459142469896,8862.527669863257],[10232.56073493945,8862.5348144115],[10232.662527227647,8862.53158881024],[10232.763463537296,8862.518026515656],[10232.86249694948,8862.494268196875],[10249.482496949478,8857.599808737416],[10249.57173499157,8857.56887943127],[10249.657657138901,8857.529669295678],[10249.739496961609,8857.482528087012],[10249.81652444437,8857.42787630722],[10249.888052498178,8857.366201452915],[10249.953443089207,8857.298053666891],[10270.010829452845,8834.31433130817],[10270.06638089107,8834.24463435596],[10286.322171500316,8811.872256356748],[10330.072956573302,8782.591818366363],[10359.47848671192,8763.348036441477],[10364.853469843083,8760.691738402695],[10379.329471681383,8755.275295076932],[10379.423236081353,8755.234635435154],[10379.51236078608,8755.184617876457],[10379.595914890777,8755.125764832701],[10379.673025675393,8755.05869102211],[10379.742887720158,8754.984097028571],[10379.804771318171,8754.902761984045],[10379.858030097159,8754.815535430567],[10379.902107770815,8754.723328446797],[10379.936543949198,8754.627104131838],[10379.960978947487,8754.527867545694],[10379.975157542876,8754.426655211446],[10381.65660194947,8735.381580246305],[10500.49611224331,8625.082941316678],[10528.338302004331,8616.826413129087],[10528.387559395218,8616.810407003803],[10600.098272882135,8591.436844042497],[10600.175075642774,8591.406036864824],[10600.24906829543,8591.36898509576],[10600.319744157194,8591.325942456415],[10633.19699075154,8569.388474342986],[10650.88156797555,8557.844829144824],[10669.064975643767,8549.377969233059],[10669.072333377293,8549.374506731974],[10706.203576953862,8531.716027800832],[10706.29220553952,8531.668266771763],[10706.375582541099,8531.611836126714],[10706.45286283747,8531.547307854722],[10759.636745311322,8482.389820399309],[10759.705123874828,8482.320128117484],[10759.766380213623,8482.244099813212],[10779.363642291508,8455.330481811228],[10787.729274996218,8446.035128004492],[10821.21730828002,8421.135214340358],[10821.292449776147,8421.073448080135],[10821.361234952728,8421.004673703914],[10831.0628023676,8410.311652588285],[10845.609649986241,8397.775305012585],[10853.31347138496,8392.715033631715],[10884.947975952444,8372.612943290796],[10885.038735858025,8372.54788257352],[10926.08812755621,8339.501531179847],[10926.160773755442,8339.436986501378],[10951.635938468371,8314.485095511389],[10951.673775754998,8314.445961584877],[10978.168976869125,8285.506456801084],[11015.726757535458,8254.218395942382],[11052.311018714641,8229.894503687374],[11052.315440083054,8229.891547052088],[11095.092619971336,8201.120877043893],[11128.00347814187,8182.287350057026],[11128.081581084845,8182.237721966838],[11128.154762134343,8182.18108672135],[11128.222394639792,8182.117929289137],[11128.283899462893,8182.048790488363],[11128.3387499368,8181.97426235576],[11152.592410279756,8145.607475701367],[11185.801991811644,8106.319928936647],[11245.908352270879,8077.417891283132],[11245.997380529352,8077.369379151946],[11246.081038999282,8077.312101598856],[11246.158467716967,8077.246647406044],[11246.22887075718,8077.1736894071155],[11246.29152441485,8077.093977570732],[11258.789419924164,8059.417476493504],[11294.746073189028,8016.594289071087],[11310.935141351834,8007.610400475821],[11326.278189977336,8000.904960214755],[11326.364335369975,8000.862267768852],[11326.445982734536,8000.811499851123],[11326.522377349427,8000.7531257436285],[11326.592813047766,8000.6876850375675],[11356.932600117616,7969.651508646329],[11423.243063620705,7949.677090286444],[11423.33072570825,7949.646172174227],[11423.415138193308,7949.607247842221],[11423.495571703788,7949.560653618892],[11423.571331248211,7949.506792104944],[11423.641762220834,7949.446128694627],[11474.079746236832,7901.744625196661],[11496.788802433544,7880.810258772209],[11502.308707864757,7876.710774067435],[11524.737801717545,7864.149872973268],[11524.764640785534,7864.134290415037],[11542.524121874987,7853.451401554833],[11542.556698429269,7853.430942272316],[11568.060352282884,7836.72118314738],[11568.06947711351,7836.71513299056],[11589.10507850924,7822.601237259854],[11589.174879961089,7822.549882129911],[11589.239973174364,7822.492676661316],[11589.299869325592,7822.430050443802],[11589.354118619109,7822.362473774717],[11612.874551245106,7790.312232819183],[11655.017379312705,7761.218793377482],[11685.261809080854,7754.415622366881],[11685.365019545408,7754.386512684813],[11685.464519417072,7754.346518321177],[11685.559164469847,7754.296099202098],[11685.647866307057,7754.23583513603],[11720.131830988612,7727.998615166834],[11744.963352818662,7715.662855689681],[11765.653009874715,7709.231076093412],[11765.735179347315,7709.201540073343],[11765.814459054178,7709.164948724795],[11786.693167593343,7698.398886621759],[11786.715983668875,7698.386745785777],[11817.937939465373,7681.251654042659],[11817.98221128248,7681.225859618136],[11826.709143100663,7675.837109618135],[11826.78641137831,7675.7842470320065],[11861.176303511442,7649.812396308785],[11967.012344289726,7648.257704624926],[11967.123222573886,7648.24989772734],[11967.232549493456,7648.229833674758],[11967.338974320732,7648.19776035738],[11972.769889499303,7646.225662143093],[11972.815240846361,7646.2079326770645],[12038.875943446044,7618.509880184724],[12094.438138609417,7629.936186667372],[12094.53131207368,7629.950807093406],[12094.625448517254,7629.9565847479025],[12094.719710591642,7629.953468238327],[12094.81325983086,7629.941485286193],[12094.905264109626,7629.920742480474],[12094.994905045158,7629.89142432949],[12095.081385276737,7629.8537916197],[12109.420760452644,7622.791843969827],[12109.464175378196,7622.7691245012875],[12190.238978009773,7577.944387659182],[12190.319460113566,7577.894604188327],[12190.394875851654,7577.837435757792],[12190.46454981856,7577.773394354492],[12190.527858030751,7577.703053518056],[12190.58423351488,7577.627043204345],[12209.481080474568,7549.454147886924],[12213.714419591117,7545.131860405749],[12213.780326593593,7545.0575150721725],[12213.838531022227,7544.976996713476],[12213.888458343341,7544.891100123286],[12213.929615726265,7544.800673183547],[12213.961596908053,7544.706608495107],[12213.984086203685,7544.609834566894],[12213.996861622192,7544.511306650637],[12213.999797057904,7544.411997311616],[12213.992863535252,7544.312886828499],[12213.976129494768,7544.214953517063],[12213.949760117524,7544.119164073262],[12213.914015694632,7544.026464031019],[12200.199691984586,7513.127372668433],[12175.810991248181,7438.771912060962],[12205.477990618952,7376.0105316919635],[12225.536193281103,7358.968530993913],[12225.60714746499,7358.902042832938],[12225.671308696197,7358.828977737501],[12225.728070319261,7358.7500265506305],[12225.776895643286,7358.665935769359],[12231.144707704998,7348.289918939063],[12231.162855859307,7348.252996568149],[12244.155913769691,7320.384760223589],[12256.899645354077,7304.423950913187],[12256.919098181877,7304.398776233967],[12265.004364592663,7293.58401471554],[12329.766267338426,7254.835485691662],[12329.851503671767,7254.778351661978],[12329.930436254785,7254.712783550771],[12330.002233964491,7254.639471759425],[12341.051056668373,7242.134014095305],[12341.11751060568,7242.050153532402],[12341.174624016638,7241.959672716298],[12341.221743025808,7241.863607536504],[12341.258328180857,7241.763057816345],[12368.023544384887,7153.818677923057],[12426.58435818209,7121.880700351143],[12426.65305005798,7121.839587057015],[12446.440907676077,7108.893096704386],[12446.516261620223,7108.838629941318],[12453.687134160855,7103.12966394474],[12453.75936775407,7103.066251854946],[12453.825179633679,7102.996197721426],[12453.883961777867,7102.920148759266],[12453.935171111034,7102.838807568487],[12453.978334521156,7102.752925642871],[12454.013053230761,7102.663296427075],[12458.377827570586,7089.557335101061],[12518.30871535091,7031.7195606157775],[12518.38511472661,7031.637042097321],[12518.451766067199,7031.546467435887],[12518.507826488456,7031.448982054089],[12521.887785378485,7024.722363349053],[12521.92813403673,7024.630943216905],[12521.959157197498,7024.535952594841],[12521.980545074211,7024.438340026419],[12521.99208409492,7024.339080237051],[12526.45960342096,6956.486127557275],[12539.659276881437,6927.665953996725],[12650.853984080572,6876.9805152538465],[12650.952159837048,6876.929010216715],[12651.044030869753,6876.866954102112],[12656.375688969885,6872.81772509012],[12656.449348320582,6872.755983145099],[12656.516740081128,6872.687454914926],[12656.577241702093,6872.612773447475],[12656.630294283555,6872.532628632794],[12656.67540773809,6872.447760830066],[12656.712165318086,6872.358954028339],[12656.740227465556,6872.26702860422],[12656.759334948898,6872.172833743424],[12656.769311257622,6872.077239596196],[12656.770064232902,6871.981129239062],[12656.76158691893,6871.885390517179],[12656.743957627163,6871.79090784262],[12656.717339212903,6871.698554024387],[12656.681977570877,6871.6091822056],[12656.638199363722,6871.523617982361],[12605.76835569242,6782.881743648506],[12634.402863188901,6696.663186559434],[12678.941855023517,6654.499477905207],[12679.013131189742,6654.42464997871],[12679.076304242066,6654.342865653097],[12679.130699523213,6654.254998344929],[12679.175736118212,6654.161986434043],[12679.210933058273,6654.064823242112],[12679.23591445731,6653.964546424462],[12679.250413526206,6653.8622268884155],[12679.254275422009,6653.7589573565265],[12679.247458901556,6653.655840696841],[12677.880069868957,6642.0922342349495],[12677.8612835344,6641.984388124517],[12677.830821534955,6641.879241671931],[12640.445498607442,6535.0906430582545],[12639.427770770597,6529.211253767544],[12639.407206300331,6529.118767652949],[12639.377981387868,6529.0286428321615],[12639.340358372941,6528.941688317742],[12639.294674981506,6528.858684663743],[12639.241341294128,6528.780376959007],[12639.180836064856,6528.70746813882],[12639.11370242365,6528.640612674953],[12639.040543000936,6528.580410700748],[12638.962014518034,6528.527402623971],[12638.878821892065,6528.482064275804],[12638.791711908192,6528.444802639502],[12599.519993252716,6513.805635709531],[12560.868991671134,6489.77114130848],[12474.361682970972,6422.447026633781],[12474.268417766476,6422.3825853264425],[12474.168458351225,6422.329113878327],[12474.063089311707,6422.287299455315],[12359.950588509664,6384.336610875502],[12279.41040293381,6272.346635622585],[12279.369228998521,6250.467348904302],[12279.369065208362,6250.4510347717105],[12278.831413386499,6220.908119791952],[12278.82536556378,6220.815013673418],[12278.81066650512,6220.72287647603],[12278.787444170606,6220.632510284078],[12278.755900718223,6220.544701764675],[12278.716310743985,6220.460215319561],[12278.669018891507,6220.379786430739],[12278.614436851754,6220.304115257862],[12275.59024255977,6216.49904874699],[12243.310820899307,6166.388525068807],[12225.394954641,6136.737458900528],[12222.719443152113,6130.75008602487],[12222.674861010866,6130.662216638726],[12222.62184783332,6130.579161239469],[12222.56091830301,6130.501726178444],[12222.492663960156,6130.430663241402],[12222.41774745862,6130.366662349713],[12222.336896132472,6130.3103448622005],[12201.059625531452,6116.99649572654],[12114.833408043156,6056.755535237068],[12099.925896512561,6040.203811260903],[12089.084559593557,6020.86786620828],[12089.019529382242,6020.76667470268],[12028.111554680927,5937.469133507613],[12011.911273278894,5907.6612542927705],[12011.857656748853,5907.573648165796],[12011.795336788142,5907.492003886913],[12005.069282527662,5899.560530170221],[11995.496441675974,5885.911688092298],[11971.421223270047,5832.653087203254],[11971.417623866624,5832.645215630671],[11968.2040887508,5825.697169489672],[11958.219612312705,5799.140617690021],[11958.167792484033,5799.025448480029],[11951.084210394481,5785.616057234012],[11951.08192907373,5785.611763941208],[11920.10921729308,5727.663586670584],[11916.192609115138,5700.74691681811],[11916.17390850625,5700.651335481111],[11916.14599862755,5700.558026620728],[11916.10914421832,5700.467875318598],[11916.063694861241,5700.381736705327],[11916.010081666402,5700.300427849141],[11915.948813182018,5700.224720005582],[11915.880470570588,5700.155331301759],[11878.2701739945,5665.517622345161],[11875.218276756492,5661.188251404675],[11817.04793772504,5571.420770176365],[11812.990540755534,5557.322298939633],[11812.958502494697,5557.228676092609],[11812.917368176692,5557.138678006382],[11812.867540575908,5557.053185914102],[11812.80950758865,5556.973036927631],[11793.043396150342,5532.338712049367],[11780.584437247775,5509.379733715306],[11780.538900513113,5509.304005595608],[11780.48685639418,5509.232593047681],[11780.428711269276,5509.166053685145],[11780.364919155438,5509.10490707012],[11767.869892376322,5498.145253465827],[11738.480871692056,5428.802281866459],[11745.703699461936,5374.359509500447],[11751.560618090678,5361.259533328795],[11751.595552033734,5361.170105963956],[11751.62174908289,5361.077740664331],[11751.638967763338,5360.983288819441],[11751.647049359583,5360.88762105182],[11751.645919378429,5360.791619191932],[11751.63558823563,5360.6961681497805],[11751.616151159878,5360.602147758133],[11751.587787315022,5360.510424662543],[11751.550758148602,5360.421844332934],[11751.505404981921,5360.337223270359],[11751.452145863866,5360.257341480798],[11751.391471717494,5360.1829352853465],[11751.323941814877,5360.114690533081],[11731.106086139127,5341.555851699724],[11731.036173478642,5341.497318636459],[11730.961219911838,5341.445396941427],[11730.881848595147,5341.4005182863875],[11730.798719413724,5341.363055787973],[11669.672293026826,5317.104775413339],[11627.626922294574,5195.249485639964],[11627.829110454244,5176.1814362424175],[11627.82510986747,5176.080849219342],[11627.811016637394,5175.981174073133],[11627.786973581342,5175.883420887193],[11627.753224345728,5175.788580268251],[11625.324057679063,5169.916318363489],[11625.279951929198,5169.823508693451],[11625.22655480913,5169.7357151880415],[11625.164430136489,5169.653864854672],[11625.094233882708,5169.578821947085],[11625.016707246668,5169.511378839743],[11624.932668828398,5169.452247661179],[11624.843005985525,5169.402052774672],[11519.232926005097,5117.215857473904],[11473.994701089761,5067.053201154051],[11473.92785740376,5066.985807851268],[11473.854925036892,5066.925055851456],[11473.776561105089,5066.87149252622],[11473.693471662327,5066.825600477675],[11443.467207269758,5051.957351697977],[11433.594092318526,5046.257309277768],[11339.47335497523,4919.012600586637],[11339.477308861982,4858.786069414442],[11342.083074899023,4817.353421806996],[11342.084912545022,4817.274272817005],[11341.567500670824,4785.693162179862],[11341.56067935926,4785.591803973978],[11341.54360981233,4785.4916608426165],[11341.516468188036,4785.393766264513],[11341.47953458838,4785.299130513299],[11341.433190168707,4785.208730231459],[11341.37791320418,4785.123498351367],[11341.314274153969,4785.044314467404],[11341.242929774098,4784.971995758534],[11341.164616339729,4784.907288555005],[11341.080142046769,4784.850860636205],[11340.990378671288,4784.803294339168],[11295.675375395462,4763.664917818759],[11295.60528673745,4763.635413694236],[11205.085778101093,4729.521540059269],[11196.438097833972,4724.207446866514],[11172.36521655542,4703.422318649057],[11172.291678704793,4703.3645974651],[11172.213071998804,4703.313995722779],[11140.6107362051,4685.000961993917],[11140.520142794549,4684.95445718429],[11140.425289544959,4684.9174068887905],[11140.327160071573,4684.890195313918],[11140.226771963618,4684.873104639989],[11140.125166232057,4684.866312094954],[11112.880519596423,4684.4354191622415],[11112.825104915148,4684.43607854361],[11095.319244045573,4685.129871795222],[11053.271342937769,4685.754787187248],[11039.164019289563,4685.2439931914105],[11019.751595954613,4683.850566283263],[11019.645981366775,4683.8485788012085],[11019.540746372159,4683.857743251912],[10965.140056716986,4691.507772808563],[10965.108603368057,4691.512707716802],[10923.639118884408,4698.697294557489],[10827.9637782313,4607.271692861744],[10809.980253022788,4563.559964470859],[10785.833787234453,4487.940476792559],[10782.856007929615,4352.631123132485],[10782.849578033894,4352.537801901356],[10782.834456323446,4352.445489769913],[10782.810775116193,4352.354994487376],[10782.778741627337,4352.26710790518],[10782.738636156175,4352.182599048121],[10782.69080963343,4352.102207385242],[10782.635680550537,4352.026636359327],[10782.573731297762,4351.956547231646],[10782.505503943192,4351.8925532957865],[10782.431595489536,4351.835214511211],[10662.932370282922,4267.775477940963],[10662.850255332914,4267.723489794922],[10662.763481508568,4267.679718391562],[10662.672868443527,4267.644577179467],[10662.57927203549,4267.618398089606],[10662.483576361712,4267.601428400036],[10655.940344671772,4266.766660659939],[10655.861516568219,4266.759760103331],[10612.853633401592,4264.704933608703],[10523.546367029152,4230.173655312132],[10517.603431373493,4226.4524428294035],[10517.543954696996,4226.417988257099],[10507.563393322887,4221.085730192583],[10507.532554510097,4221.069934678396],[10492.932793674769,4213.908521856835],[10492.899535461034,4213.892959068853],[10451.72790467127,4195.5408984667965],[10426.547343949831,4168.57002853934],[10426.478800307355,4168.503314740865],[10426.404196329757,4168.443454834774],[10426.32421456598,4168.390996477061],[10426.239586766129,4168.346419607239],[10426.151087186714,4168.310132057389],[10426.05952550701,4168.282465820918],[10425.965739421328,4168.263674015169],[10420.662581272822,4167.462685587011],[10420.561122904383,4167.452617857957],[10390.769626740701,4166.024335675519],[10390.76172731803,4166.023988253248],[10380.219035106442,4165.602067627194],[10314.487823898487,4158.782108700078],[10284.606129045227,4154.518136173482],[10284.514702927128,4154.50935079647],[10284.422856379226,4154.508990854161],[10284.33136421146,4154.517059382999],[10284.24099824425,4154.533488317536],[10259.958490361158,4160.111092048141],[10247.939827794526,4162.2287502129775],[10206.55395051612,4164.5544411968895],[10200.325554949835,4164.111867575149],[10200.221937245726,4164.1098885831625],[10200.11867117646,4164.118644378559],[10200.016865875907,4164.1380409193225],[10199.917614788477,4164.167869876034],[10178.97339982362,4171.666136525144],[10141.908365725503,4177.436040309997],[10035.394291467393,4168.311701766189],[10027.788049742094,4166.685264139094],[10027.685652356055,4166.668867169841],[10027.58210745548,4166.663162887825],[10027.478528571059,4166.668212637381],[10027.376029598949,4166.683962113071],[10027.275712821833,4166.710241943692],[10027.178657054887,4166.746769513705],[10027.085906044105,4166.793152002506],[10026.998457241767,4166.848890608856],[10000.727919139003,4185.577124267493],[9938.718722842734,4194.245516068212],[9904.16494624978,4190.589965133332],[9904.067316977797,4190.584444288155],[9903.969615257578,4190.588485122995],[9903.87277530853,4190.602048999571],[9903.777723109832,4190.625006220714],[9903.685367546284,4190.657137270519],[9903.596591717584,4190.698134913353],[9903.512244494157,4190.74760713162],[9903.433132400321,4190.80508087422],[9903.360011902312,4190.870006579844],[9903.293582175007,4190.941763431849],[9903.23447841643,4191.019665294483],[9903.183265774034,4191.102967273682],[9903.140433940773,4191.190872839713],[9903.106392472686,4191.282541443554],[9903.081466872743,4191.377096554189],[9903.065895478394,4191.473634039953],[9903.059827182606,4191.571230813804],[9903.063320010144,4191.66895365983],[9903.076340562751,4191.765868156627],[9903.098764338496,4191.8610476121885],[9903.130376922256,4191.953581924893],[9903.170876035945,4192.042586285855],[9903.21987442888,4192.127209639425],[9903.276903580658,4192.20664282095],[9903.341418181128,4192.280126293962],[9903.412801344613,4192.346957412839],[9903.490370508558,4192.406497141464],[9903.573383960145,4192.458176163662],[9943.841798319187,4214.872550837164],[9991.866407748746,4264.42414796565],[9908.118470127823,4417.721169466515],[9845.836709887675,4403.048867008262],[9845.738738118678,4403.030883609902],[9845.63946330342,4403.022736144515],[9845.539870433724,4403.024505450194],[9827.480697013705,4404.24695850924],[9817.738097763333,4403.585514102371],[9789.21693336862,4397.109894039647],[9789.09891245215,4397.090433739586],[9788.979412267861,4397.085204403273],[9760.601498689282,4397.542435339745],[9719.338687848503,4394.452189738316],[9712.554426641776,4392.289492956367],[9712.479663394406,4392.268817494001],[9671.263020442742,4382.574366026746],[9621.317394058311,4357.2042800018025],[9621.230849214495,4357.165368751525],[9621.141005872632,4357.134835665988],[9621.048672990746,4357.112955668007],[9620.954681942909,4357.09992576717],[9584.89203337564,4353.835008942848],[9526.12378078293,4314.430163094074],[9526.039077048581,4314.37924790705],[9525.949761242124,4314.33694228568],[9525.856705718088,4314.303659432308],[9525.760819357114,4314.279724423186],[9525.663038688857,4314.265371033436],[9525.564318744813,4314.26073945375],[9525.465623730466,4314.26587492113],[9525.367917607804,4314.2807272770615],[9525.272154680235,4314.305151457413],[9525.179270271828,4314.338908909287],[9525.090171591924,4314.381669920991],[9525.005728874361,4314.433016842348],[9524.926766877821,4314.492448163928],[9524.854056830354,4314.559383415324],[9524.788308896726,4314.633168834657],[9524.730165242196,4314.713083753915],[9524.68019376042,4314.7983476377785],[9524.638882526804,4314.888127707168],[9524.606635031425,4314.981547073062],[9524.583766238115,4315.077693301147],[9524.570499508181,4315.175627323627],[9524.566964418824,4315.274392611181],[9524.573195497536,4315.373024515453],[9524.589131884883,4315.470559690854],[9524.61461792891,4315.566045503634],[9524.649404705417,4315.658549336325],[9524.693152449212,4315.747167696698],[9529.667896190402,4324.682704792305],[9529.670569091091,4324.6874750701],[9543.39135387457,4349.018478550463],[9434.366128599399,4485.0268548666045],[9323.27538832269,4375.223267734347],[9323.208807959712,4375.1631187299645],[9323.137104084375,4375.109179859714],[9323.060853973639,4375.061885377213],[9322.980671505513,4375.0216160431255],[9213.587718766608,4326.098245433915],[9196.260561236382,4286.522054662401],[9167.219094908623,4214.428044208454],[9167.176195578619,4214.335477458431],[9167.124087756141,4214.247763545274],[9167.063313829207,4214.165815479222],[9166.994506390933,4214.0904862540665],[9166.918381654896,4214.022559968375],[9166.835732000101,4213.96274366385],[9166.747417723149,4213.9116599657555],[9151.567255935783,4206.136101414138],[9151.467536711776,4206.091716361522],[9151.36357405723,4206.058463948195],[9151.256606578692,4206.036740341963],[9151.147908682055,4206.026804356926],[9109.437821441585,4204.501488412067],[9076.769831611984,4193.380181990683],[9059.99010713265,4186.287183011483],[9059.908551655286,4186.256819522849],[9059.82466513717,4186.23366159961],[9059.739082870816,4186.217884622217],[9059.652452991035,4186.209608073463],[9042.793636595206,4185.336821267739],[9042.667501999636,4185.3382578903465],[8998.613407253146,4188.626479246912],[8933.56552601277,4174.551939185308],[8925.18210915003,4172.274961765798],[8925.079925780235,4172.252870958377],[8924.975994510447,4172.24156892336],[8924.871451251747,4172.241179185718],[8924.767438603913,4172.251706005065],[8924.66509336744,4172.273034329111],[8924.565534118947,4172.304931051115],[8924.469848985746,4172.347047557612],[8882.375815044334,4193.567295325952],[8860.538731643906,4198.482743717766],[8860.438453846939,4198.510875094578],[8860.341645786255,4198.549283561258],[8860.249357539948,4198.597552500747],[8860.162590159633,4198.655158339332],[8835.394831880354,4217.026276754344],[8725.973140757907,4223.1756090141735],[8669.750404616487,4168.8687471883795],[8686.689752999915,4039.228653988535],[8686.697292763558,4039.1412491683973],[8686.697142919147,4039.0535198792986],[8686.689304619953,4038.9661413253807],[8683.82599842407,4017.620545649215],[8700.173143343805,3924.5966078149772],[8700.185240640074,3924.5008620706767],[8700.188052235198,3924.404396081533],[8700.181551943133,3924.308108292423],[8700.165800304942,3924.2128954885416],[8700.14094402496,3924.1196444431093],[8700.107214604444,3924.0292236583296],[8700.064926185456,3923.942475276511],[8700.014472625082,3923.8602072367003],[8699.956323827208,3923.7831857498645],[8699.891021366017,3923.7121281627183],[8699.819173441998,3923.6476962766455],[8699.741449217412,3923.590490183953],[8699.658572583985,3923.541042678856],[8699.571315420877,3923.4998142952477],[8699.480490405715,3923.4671890174777],[8695.626549456696,3922.2894347901397],[8631.13756854408,3771.607033093648],[8631.094137078795,3771.5180189484845],[8631.042113097956,3771.433737778383],[8630.982006946942,3771.355016365627],[8630.914408255689,3771.2826269523352],[8630.839980154526,3771.2172796649143],[8630.759452769002,3771.1596155478514],[8630.673616057484,3771.1102002751913],[8630.583312061826,3771.069518601381],[8630.489426647084,3771.037969605919],[8630.392880811358,3771.015862778464],[8585.853097324145,3763.1153701676267],[8585.751482874784,3763.1026709703856],[8585.649102474548,3763.1004305292327],[8585.547029762727,3763.1086723391495],[8507.432297126148,3773.4637942501818],[8499.765076157586,3772.3140142146162],[8373.082651852343,3713.317276314992],[8365.521111026017,3701.8968616954517],[8358.79696763666,3691.0863274115486],[8358.742804096924,3691.007854505845],[8358.681412866774,3690.9348969645853],[8358.613352095992,3690.8681180948233],[8358.539240571732,3690.8081250290725],[8358.459752092704,3690.755463205446],[8358.375609343191,3690.710611408708],[8358.287577322651,3690.673977417308],[8317.732837868958,3676.0240059488597],[8295.947612370548,3662.0150740860854],[8295.854045419528,3661.9617977154694],[8295.755292809714,3661.9188900466434],[8295.652499394528,3661.8868485144753],[8295.54685687304,3661.8660445811256],[8295.439589974423,3661.856719429631],[8268.392035181318,3660.9677707682304],[8224.285773187155,3652.654227307777],[8169.7626503664,3629.9062268319894],[8159.226214515341,3622.8351717987366],[8159.131082559781,3622.7786982976286],[8159.03029459417,3622.7330788797885],[8111.776583314216,3604.4217989265444],[8111.694804603187,3604.394106259095],[8111.610941944426,3604.373571024172],[8111.525620503979,3604.360346304588],[8089.625631154299,3601.9284698663105],[8042.526638452423,3580.809454563793],[8005.252329920614,3530.5869840726036],[8005.241927299503,3530.573217624659],[7924.760114420925,3425.9553977201267],[8084.915546724389,3354.1163912841794],[8098.531760343561,3349.019564495105],[8138.812285144888,3334.5042256133725],[8206.500171103222,3313.3779986785207],[8242.337117202063,3307.6374119220086],[8242.345307169831,3307.6360651177656],[8294.200495697587,3298.887536536673],[8305.438971019357,3300.0354041539326],[8305.541111333983,3300.0405795688334],[8305.643246088128,3300.035295556421],[8305.744307010604,3300.0196073844463],[8305.843237061887,3299.993679142228],[8305.93900149015,3299.957782024368],[8306.030598654177,3299.912291494216],[8306.117070499953,3299.8576833567317],[8342.321945335263,3274.314073343556],[8342.388479119198,3274.262815241163],[8342.450476919395,3274.2061547590242],[8342.50750139485,3274.144491587969],[8347.028860812925,3268.8234549598133],[8405.785587927558,3237.328736598428],[8405.874274797976,3237.27510498597],[8405.956934312871,3237.21258206668],[8406.032678567639,3237.141839443679],[8406.100693939396,3237.0636370137668],[8406.160249826684,3236.978814804832],[8409.535980820834,3231.598113050446],[8409.586335027843,3231.5077907168775],[8409.627092241906,3231.412751148241],[8409.65781661882,3231.31401066633],[8409.678179602235,3231.2126251692844],[8409.687963437123,3231.109678840163],[8409.687063498382,3231.006272553047],[8409.675489409661,3230.903512100659],[8409.653364940446,3230.8024963693756],[8375.981497328154,3107.8677445963262],[8375.955146022914,3107.7856173909636],[8375.921815755191,3107.7060663859284],[8375.88175447782,3107.629683382558],[8375.835260217422,3107.5570366145735],[8342.66473839923,3060.3445855122313],[8330.656192650426,3017.0622816755626],[8330.64235768791,3017.016665951683],[8317.036332259497,2975.7258422922027],[8317.02541660037,2975.694478021588],[8301.830996432791,2934.2651579004446],[8300.77732979842,2926.479524225266],[8300.756038220847,2926.3692366905425],[8300.72251257749,2926.2620326662313],[8284.174988810762,2882.2040578303763],[8279.88783288321,2858.2063397158327],[8347.384871077853,2736.5629150641025],[8378.430897088909,2705.8244914162337],[8416.822453285178,2672.4383341560233],[8416.897548392508,2672.3658076670017],[8416.96467281045,2672.2858471813197],[8417.023094925356,2672.1993242176363],[8425.021810160226,2658.9060711341463],[8442.289978353268,2637.703326049138],[8468.64447058071,2605.6935852696092],[8468.72642818281,2605.5783024266098],[8479.462919638576,2587.957644844953],[8479.500621999603,2587.890005779882],[8496.888988204293,2553.640192347046],[8496.94361188779,2553.5108177489587],[8513.185375562229,2505.974219292785],[8517.892644246842,2498.5830874706817],[8517.94835285675,2498.483495930103],[8517.99235251017,2498.3782060698513],[8518.02407024695,2498.268588966503],[8519.24904722245,2492.9058333945745],[8519.268220017291,2492.791954710425],[8524.396154670374,2445.943758675273],[8533.809864579416,2411.082921211465],[8533.82491822177,2411.0188720937676],[8541.69127522001,2371.7981154299873],[8543.271527027513,2365.7992511329485],[8543.285970606667,2365.736211481953],[8549.437351853552,2334.241965135347],[8562.821822904914,2282.399652624967],[8576.815275519817,2250.367355238074],[8576.848600521931,2250.280195873354],[8576.87365619617,2250.190309701932],[8576.890224374994,2250.098479390625],[8576.898160794124,2250.0055045344766],[8576.897396348693,2249.9121946944406],[8576.887937694966,2249.819362348278],[8573.10291515357,2224.468850229211],[8571.983357850133,2213.1847806049946],[8583.883223289497,2145.801601173763],[8583.895604997519,2145.7032232421584],[8583.89818326557,2145.6041027263495],[8583.890932745348,2145.5052141320784],[8583.873924720518,2145.407529684945],[8583.847326405883,2145.312009771948],[8583.811399303417,2145.219593499415],[8583.766496631295,2145.1311894601595],[8583.713059851221,2145.047666800625],[8583.651614328188,2144.969846675854],[8583.582764165334,2144.8984941762797],[8583.5071862647,2144.834310805723],[8583.425623672236,2144.7779275845396],[8577.447480295963,2141.069311855525],[8548.39511514046,2116.0998799322606],[8488.248294527595,1997.4741857399326],[8509.34691661182,1956.018375541358],[8604.120072720852,1803.3192844008088],[8611.097806908352,1802.1058008343857],[8611.2133910727,1802.0785426369202],[8758.186920484464,1758.0579544016261],[8758.278490518527,1758.0256051681922],[8758.366490802597,1757.984526048849],[8758.450091358116,1757.9351044831196],[8758.528503702762,1757.8778065926779],[8758.600988287057,1757.8131727851012],[8758.666861469448,1757.741812656992],[8758.725501964116,1757.6643992445415],[8758.776356700653,1757.581662675759],[8758.818946040372,1757.4943832842364],[8758.852868300037,1757.403384249399],[8758.877803540365,1757.3095238326518],[8758.893516583537,1757.213687282649],[8758.899859231295,1757.116778486033],[8758.896771662678,1757.0197114423868],[8758.884282998222,1756.923401643808],[8758.86251102532,1756.8287574404033],[8758.831661087292,1756.7366714731454],[8758.792024146685,1756.6480122548905],[8758.743974041054,1756.5636159789601],[8758.687963957083,1756.4842786325476],[8758.624522156348,1756.41074848933],[8690.278368310193,1684.5671587457402],[8690.210197206261,1684.5019546275],[8690.136185315405,1684.4434643166865],[8690.056991260302,1684.392208311348],[8684.869057249898,1681.3717527050303],[8653.665694150694,1654.8207526741614],[8653.569021987296,1654.748095374084],[8653.464286191396,1654.6876387492389],[8620.47707391518,1638.2205031993667],[8620.467390033871,1638.2157341461054],[8586.086805297866,1621.5141700846511],[8579.169680608584,1456.7167091781969],[8640.78835712942,1430.8274594942943],[8640.808085069988,1430.8189201261582],[8668.070603651528,1418.668582310359],[8765.499272831028,1409.0182393689606],[8820.697451481676,1415.4338660853978],[8820.795006807197,1415.4403928416675],[8820.892733216038,1415.4373614888646],[8820.989696478906,1415.4248010056301],[8821.084969661904,1415.4028314656637],[8907.697758456034,1390.9146677004946],[8945.7890047077,1407.120185299069],[8945.884826487405,1407.1552928270164],[8945.983727388137,1407.180451599215],[8946.084677421108,1407.195399603356],[8946.186625257136,1407.1999811656624],[8946.28850917558,1407.1941485721318],[8946.389268121444,1407.1779625654463],[8946.487852755607,1407.1515917123768],[8946.583236382983,1407.115310648269],[8946.674425644906,1407.0694972168953],[8946.760470864305,1407.0146285354556],[8946.840475935978,1406.9512760257112],[8972.62955334849,1384.2958412431026],[8972.700818060131,1384.2266717193272],[8972.764870364917,1384.150774523092],[8972.821078509702,1384.0688982346778],[8972.868888108938,1383.981850406643],[8972.90782761262,1383.890489598866],[8972.937512957224,1383.7957169104984],[8972.95765135376,1383.6984670923512],[8972.968044175565,1383.599699327369],[8972.968588917387,1383.500387770131],[8972.95928020639,1383.4015119386845],[8972.940209855158,1383.3040470534768],[8972.91156595613,1383.208954418677],[8972.873631026436,1383.1171719407537],[8972.826779221403,1383.029604877826],[8972.771472644232,1382.9471169110284],[8972.708256788239,1382.8705216259539],[8972.637755156602,1382.800574488191],[8936.057034560725,1349.9468459241862],[8935.976299952188,1349.8815800596774],[8935.889233920409,1349.8250361281207],[8935.796774837836,1349.7778235436172],[8935.699919201657,1349.7404511497155],[8935.599710893839,1349.7133217352416],[8935.49722993047,1349.69672769316],[8935.393580821663,1349.6908478692453],[8855.042445105977,1349.310284539853],[8795.516847806577,1342.1310697814504],[8766.747924263977,1328.6779344082663],[8766.638144778879,1328.6343014393972],[8766.523985812812,1328.6039188492061],[8759.71200191402,1327.2158797462732],[8759.694296402093,1327.212438003057],[8691.034749095026,1314.5073326045015],[8669.100453741463,1306.5011110980247],[8623.345133151453,1286.31927814532],[8567.981525306166,1231.207634471582],[8520.211254307156,1091.4440764600588],[8520.174315298198,1091.3513922153327],[8520.128324183113,1091.2628502651824],[8520.073738796993,1091.1793320322913],[8520.011102529692,1091.1016689289415],[8519.941038916459,1091.0306340804239],[8519.864245430726,1090.9669346286819],[8519.781486540862,1090.9112046928146],[8519.693586099998,1090.8639990565093],[8519.60141914468,1090.8257876452478],[8519.505903184008,1090.7969508482645],[8519.407989065961,1090.7777757318247],[8519.30865151184,1090.7684531815203],[8515.09365151184,1090.5842865148536],[8515.024315588866,1090.5836632322375],[8455.422981101841,1092.1149935955107],[8418.234907759916,1073.601572113005],[8418.157920017944,1073.5672149239133],[8418.078311924073,1073.539464604154],[8417.996649295132,1073.518518389926],[8378.863139688223,1065.2217441963776],[8378.751079087355,1065.204555365279],[8378.63779306044,1065.2001610180962],[8349.374537458149,1065.7253643033662],[8320.841863854435,1065.9558325642683],[8282.24710897236,1064.8607702677282],[8267.931318680528,1061.924043109102],[8267.83589925685,1061.9092281233613],[8267.739495788952,1061.9036854169487],[8267.643007176526,1061.9074666720005],[8267.54733311319,1061.9205366307704],[8249.559074003879,1065.2695244850215],[8249.441662220914,1065.2988318209436],[8223.71663935362,1073.4021093350677],[8169.50996188119,1080.8464669684793],[8169.399576015413,1080.8680105194621],[8133.251211893766,1090.0599763192163],[8115.6568100513705,1090.6202450978533],[8097.487870408147,1089.698284487213],[8041.320913715919,1074.9809130523151],[8041.22907637951,1074.961405125017],[8041.135814290485,1074.9505959466571],[8041.041949522038,1074.9485807964193],[8040.948309459774,1074.9553774371593],[8040.8557195085905,1074.9709259588296],[8040.76499581702,1074.9950893065668],[8040.676938083155,1075.0276544887831],[8040.592322505592,1075.0683344546128],[8040.511894941505,1075.116770624168],[8040.43636433217,1075.1725360492962],[7987.503976276436,1118.2350466916687],[7970.90800270178,1127.307001885474],[7918.15623139214,1135.3172575667538],[7899.25532624526,1137.0190288067865],[7899.163543229106,1137.0316010777426],[7899.073317508357,1137.0526130592457],[7898.985423416754,1137.0818844226028],[7898.90061527757,1137.1191639555864],[7898.819620929863,1137.164131718383],[7898.743135482033,1137.216401789376],[7898.67181534627,1137.2755255771958],[7851.145433193671,1180.5414319099139],[7791.667768772687,1206.6691844055633],[7783.657256947352,1207.2949290100012],[7783.554209987496,1207.3083950230855],[7783.453117020941,1207.3324828116413],[7783.355069852684,1207.3669322273245],[7783.261127393068,1207.4113712161204],[7783.172304221542,1207.4653198365306],[7783.0895596291975,1207.5281954429447],[7765.11151274581,1222.7255775029278],[7710.49320979334,1250.2643246423233],[7705.328564714171,1251.4517420159143],[7705.242570753235,1251.475599068069],[7705.159046154001,1251.5070277425211],[7705.078656116422,1251.545777737528],[7705.002040876425,1251.5915404434697],[7704.929810606994,1251.643951400653],[7667.551968742654,1281.399528382572],[7662.773407621037,1284.4238668862229],[7662.748340051963,1284.440262989021],[7659.612451022962,1286.5591028376969],[7659.534296533568,1286.6176651904284],[7659.462227186212,1286.6835718102907],[7659.39693035479,1286.75619410147],[7638.361428864368,1312.5843769503167],[7634.594514969945,1316.708849959013],[7634.539461177129,1316.7745800019031],[7619.00285424762,1337.0283912324885],[7618.959127032712,1337.090093142189],[7541.023898875749,1456.3801669102502],[7450.830913001344,1493.472487541252],[7401.800059699577,1479.1566573833848],[7401.719695623549,1479.1367631796807],[7364.644685646695,1471.5724244455437],[7364.633793979485,1471.570265156339],[7342.941063399432,1467.3946811015833],[7294.067285574099,1436.1432256689318],[7293.968715956298,1436.0877873428556],[7293.864517163956,1436.0438329434653],[7293.756021834767,1436.0119246201698],[7268.585748203039,1430.132842962013],[7258.047921403299,1425.5895289077248],[7196.305153803358,1329.0171215657547],[7188.879143979084,1306.5366183553188],[7188.844140767022,1306.4457653808913],[7188.800468261633,1306.3587469915126],[7188.748540455901,1306.2763880769446],[7188.688849598827,1306.1994693575145],[7176.597797539347,1292.0939412575356],[7171.0724093630515,1284.1410544521127],[7171.067511671138,1284.1340688144141],[7167.392819468677,1278.940087966261],[7167.321176141557,1278.8502538922023],[7145.041955804352,1253.9901565184475],[7115.95815843129,1218.5556848381648],[7115.894936231872,1218.4856708666712],[7115.8253979559695,1218.4219258206815],[7115.750162424889,1218.3650169675327],[7115.669899159825,1218.3154507392624],[7062.677516515758,1188.948868356641],[7025.809939355174,1143.152627286449],[6994.312739356439,1103.2550500094203],[6994.249209535978,1103.182124752207],[6994.1789320059925,1103.1156777808178],[6891.004925096721,1014.6118237701131],[6888.20820447026,1009.8398236847995],[6888.155149792418,1009.7586039622197],[6888.0944747145795,1009.6829073499706],[6888.026750276729,1009.6134462623412],[6887.952613863498,1009.5508744283412],[6887.872763205454,1009.4957807391684],[6887.787949812447,1009.4486837058924],[6887.698971900821,1009.4100265795189],[6887.606666881046,1009.3801731793574],[6887.511903476479,1009.3594044689571],[6853.538678975866,1003.6245974481263],[6830.312020446001,996.8172888997856],[6830.219066692764,996.7948110335155],[6830.124390835957,996.7813152248839],[6830.028858748274,996.7769249018965],[6829.933344133205,996.7816802169314],[6829.838720534438,996.7955376795193],[6829.745853346736,996.818370554092],[6786.7423568432405,1009.6005383862597],[6786.656853302012,1009.6302263068],[6786.57438432124,1009.6675236981185],[6786.495625505635,1009.7121250114749],[6781.639781349791,1012.7601769595268],[6781.5677115638955,1012.8099441524182],[6781.50027278704,1012.8658265190784],[6777.528844215611,1016.4613863646383],[6777.45473782045,1016.5359311648184],[6777.388865592381,1016.6178429137126],[6777.331955332906,1016.7062165942066],[6777.284635825556,1016.8000757933388],[6777.247429888664,1016.8983834903803],[6777.220748598907,1017.0000535145496],[6777.204886749455,1017.1039625457719],[6776.440665736441,1024.8058589173602],[6752.275535010589,1083.170333886384],[6704.659674874224,1127.505014425951],[6615.866923128339,1178.9133650914105],[6538.965528241802,1215.6734842113685],[6533.949738156886,1216.382250559709],[6533.8591059119935,1216.3993531379951],[6445.150114860038,1237.4173566635775],[6434.942018831755,1236.0919968438507],[6434.795986801855,1236.0838227538857],[6420.1736305866425,1236.3365129208614],[6420.071497887623,1236.343518751938],[6419.9706166383,1236.3609297660662],[6419.872044091303,1236.3885634928597],[6419.77681330367,1236.4261303261676],[6419.685922310233,1236.473236559195],[6419.600323664044,1236.529388510617],[6419.520914453455,1236.5939976984446],[6419.4485269004845,1236.666387007413],[6419.383919638984,1236.745797785267],[6415.959141070907,1241.4256487696866],[6322.199453760391,1286.9401663979238],[6314.707170312324,1287.2492368694911],[6314.598963658641,1287.2596137981175],[6314.492522646178,1287.2816744285415],[6314.389105020315,1287.3151580844008],[6306.316348175641,1290.423073508635],[6306.271866565139,1290.4414392060307],[6209.87554976378,1332.9847696073489],[6152.025585897235,1344.3844225885837],[6120.5897556863865,1346.960483667726],[6120.498631400288,1346.9721854277857],[6120.408965620239,1346.99220078673],[6120.321515176236,1347.0203608035865],[6120.2370181995875,1347.0564277918547],[6120.1561878926605,1347.1000973257142],[6120.079706509051,1347.151000809551],[6063.346891799008,1388.7938579524082],[6063.269019467364,1388.8572730330095],[6063.197900378515,1388.9281789402714],[6063.134251809188,1389.0058605475274],[6063.07871569164,1389.0895343912962],[6063.031852139412,1389.1783565729618],[6052.056239679325,1412.781867539396],[6011.25720446281,1451.8226390726163],[6011.193941177269,1451.888992581809],[6011.137020582746,1451.960861054721],[6011.086921098629,1452.0376404345752],[5998.160878584647,1473.9837993132949],[5922.903277431668,1565.301694913323],[5906.803575956137,1571.0789772777114],[5866.7105572492555,1579.614949973251],[5848.675407045677,1582.785134515983],[5789.6089783749,1583.21020393352],[5769.492481832621,1582.6211538291934],[5769.400111580922,1582.6227182332232],[5769.308279876765,1582.6328003396593],[5735.661600687608,1587.9094821044478],[5726.589603840335,1589.0226338067387],[5726.575251948001,1589.0245003277219],[5686.3196276886665,1594.5564241375387],[5654.970395536296,1597.2107080545231],[5654.966592988811,1597.2110373194312],[5627.092063642188,1599.6783130669394],[5627.007145657635,1599.6895120482336],[5626.923497521888,1599.7079367780443],[5614.293702509885,1603.0628998937373],[5592.233169823874,1605.560136997014],[5566.232954414996,1608.0576430275921],[5566.14762870716,1608.069567588736],[5518.7862940461,1616.7830848619399],[5506.073833529058,1617.2137601860104],[5505.980429019546,1617.2213178422587],[5505.888142075216,1617.2375856162732],[5505.797783115768,1617.2624206522617],[5505.710145630262,1617.295604860845],[5505.625999209078,1617.3368468342132],[5505.546082785748,1617.3857844051286],[5505.471098147983,1617.4419878273063],[5478.002115517709,1640.1165429496034],[5477.932938909142,1640.1793016609045],[5477.86991948641,1640.2482408469714],[5477.813607034676,1640.3227590782758],[5477.764492827093,1640.40220625337],[5477.723005338905,1640.4858892704099],[5477.689506509409,1640.573078073816],[5471.020464608031,1660.6906589959603],[5466.565845666485,1670.8095623385884],[5429.757784982818,1723.9956469579179],[5412.635030967658,1738.5037107484266],[5412.551869596064,1738.582805206533],[5412.478318487652,1738.6709073147947],[5373.173385871003,1791.6591940340138],[5334.782199843162,1836.9809722309103],[5299.995800712489,1861.4688428339844],[5299.913165245899,1861.5337668733673],[5281.123615869101,1877.9639907750086],[5281.060767163995,1878.0239600485104],[5281.003360167494,1878.0891575197086],[5280.951828088496,1878.1590911906542],[5280.906559802162,1878.2332333227398],[5195.836156466941,2032.2287101652894],[5186.791580672013,2031.8803867752836],[5186.709352861923,2031.8806032657665],[5155.358636469173,2033.2533382004483],[5155.3538620853315,2033.253558686139],[5133.012235789878,2034.338827239462],[5121.344372468999,2033.8843939529936],[5121.318296974935,2033.8837188310342],[5090.638442429481,2033.4896824673979],[5090.548232302578,2033.4925973724228],[5077.94560527555,2034.4705649399903],[5077.861891063921,2034.480626526483],[5077.779322032673,2034.4977045776088],[5042.985205314225,2043.2387528938286],[4946.913540637476,2045.2675496332658],[4946.826324765865,2045.2732116020827],[4946.739936381025,2045.2864672844987],[4946.655035374984,2045.307215424781],[4946.572270278187,2045.3352975348125],[4932.252546717533,2050.9029633065006],[4885.375543347969,2065.423347928788],[4885.300102044587,2065.4500690804516],[4885.227039239929,2065.4827376705284],[4811.7618606685,2101.926041241957],[4811.675643830263,2101.9742565170513],[4811.594604676716,2102.030742900725],[4811.519533992454,2102.094949195685],[4811.451164321414,2102.166248873314],[4811.390162818671,2102.2439461873682],[4811.337124740302,2102.32728296311],[4811.292567634844,2102.4154459956194],[4811.256926293023,2102.507574985092],[4811.230548505047,2102.602770931695],[4811.213691666849,2102.700104908055],[4811.206520268397,2102.798627123785],[4811.209104288599,2102.8973761935918],[4818.715324571696,2202.0321713941808],[4718.050302458832,2261.2866996081943],[4717.971934367912,2261.3379145784197],[4717.898699149018,2261.396233816783],[4717.8312386744865,2261.46114618252],[4717.770144203727,2261.5320827491996],[4717.715951201112,2261.608421791094],[4717.669134642887,2261.689494232315],[4717.63010485422,2261.774589510952],[4717.599203912897,2261.862961806809],[4717.576702651172,2261.953836578173],[4707.874339153549,2310.8460636096715],[4689.547888259189,2375.9299942284824],[4673.059285394729,2397.3358099791276],[4628.073046562298,2428.102181239985],[4607.257970289432,2435.4240061986493],[4607.161354011706,2435.463777609605],[4607.069414807309,2435.5134128082627],[4606.98315632783,2435.5723699534524],[4606.903520211394,2435.6400054412525],[4606.831375803297,2435.7155809308724],[4606.767510665824,2435.7982714047284],[4580.138825858568,2474.2753689103974],[4580.103660572764,2474.3298780500018],[4548.647311366415,2526.7473383674624],[4548.628980839423,2526.779196231222],[4534.5537362400055,2552.3159993466525],[4522.499965976989,2568.9915556619685],[4522.440233654406,2569.084630265024],[4502.588925549173,2604.1414515650026],[4464.665467472122,2654.724731921256],[4464.5873021717,2654.8464280588314],[4451.1797969450345,2679.4728694383552],[4417.292823548522,2731.0941829601],[4408.778431151485,2743.303483389804],[4408.735788245569,2743.370100218776],[4408.69854375155,2743.439878918911],[4388.967439847661,2784.2109268892737],[4298.244143458408,2825.60515210119],[4267.448440471845,2832.724644921444],[4267.317647543676,2832.764475382828],[4142.643963333149,2880.265528014407],[4142.551507195024,2880.30621355801],[4142.46362723123,2880.3560188077204],[4071.3472635948656,2925.552382444084],[4071.269056825399,2925.607508775707],[4071.196476661422,2925.6698572402092],[4071.1301875896665,2925.7388570242265],[4071.0707965005863,2925.813876420162],[4071.018847132148,2925.8942286096003],[4070.9748150917917,2925.979177951274],[4070.9391035021486,2926.0679467160157],[4070.912039310365,2926.1597222070345],[4070.8938702948326,2926.2536642003242],[4070.8847627967275,2926.3489126370932],[4067.44785149432,2998.6980708131046],[4047.731285037058,3036.8078567311577],[3987.867916198812,3086.363855029549],[3987.7960991182613,3086.42944693812],[3987.7309938160292,3086.5017054403875],[3987.6732161877235,3086.5799469715334],[3987.6233128092176,3086.6634313673358],[3987.5817557660507,3086.7513688661174],[3961.7971880550035,3148.9800180925185],[3944.6278526144074,3168.8010306768783],[3929.781001683062,3180.7105604923336],[3898.3380949485795,3201.8573156849156],[3898.2564747005954,3201.9184771236014],[3898.181508909639,3201.987634311582],[3898.1139774138323,3202.06406783523],[3898.0545827154,3202.1469825882073],[3870.208415468013,3245.5348745742695],[3870.156694770094,3245.625549484122],[3870.11470839235,3245.721122042704],[3870.0829138590743,3245.820550798724],[3870.0616576343377,3245.9227522800243],[3870.051171346581,3246.0266128001417],[3868.977614438972,3268.1874198635537],[3845.4704281571544,3399.78420533228],[3825.8728914300405,3426.618056466176],[3800.4227621465957,3460.784096666722],[3787.6964321496507,3471.8693245187424],[3787.624757799757,3471.938319074959],[3787.5602936410296,3472.014093961695],[3787.50367770498,3472.095899199947],[3787.4554703456397,3472.182925125542],[3787.416148693478,3472.2743104027563],[3781.796479718548,3487.3607913678447],[3768.2889326543836,3509.472933306501],[3768.2416914516334,3509.5596155520166],[3768.2032272431156,3509.6505333587875],[3768.1739148834254,3509.744800683425],[3768.154040037422,3509.8414988396707],[3768.1437963962708,3509.9396854514916],[3767.7947506219375,3516.329347533442],[3706.0110640189546,3621.3942987860605],[3639.7124411391615,3683.516938406531],[3611.8204819390867,3691.8227681005437],[3611.7663712643316,3691.840574418331],[3572.1104889113903,3706.1543979477424],[3572.022507161649,3706.1909812650397],[3571.9384066738467,3706.2357722835964],[3571.858951007025,3706.288364340049],[3571.784861549306,3706.3482799444178],[3571.7168109683134,3706.414975115297],[3571.6554171039425,3706.487844318734],[3571.6012373589083,3706.5662259659484],[3571.554763638018,3706.649408419979],[3571.5164178821037,3706.7366364567297],[3569.1838091864515,3712.8133755871645],[3569.1564138769186,3712.8951123349652],[3569.1361599740067,3712.9789048068365],[3569.123197992549,3713.0641303074926],[3566.5139839808085,3737.170546943236],[3529.220662904868,3795.2519338695247],[3529.1708639223707,3795.338763912538],[3526.408108616457,3800.768828413658],[3504.352578105988,3831.504413810139],[3497.029082421123,3841.455544644434],[3496.9692656666675,3841.5468784310897],[3476.0476661830057,3877.649427718008],[3461.308944459132,3900.03174109035],[3458.460030583109,3903.1658210237238],[3458.4101675322704,3903.2251388885284],[3432.3381645918007,3936.800475497056],[3423.86564599334,3944.6323420362896],[3423.7898830806525,3944.7104371730647],[3423.7230533213506,3944.7963013250633],[3423.6659479042205,3944.888917957809],[3414.4471811374274,3961.840621980463],[3405.08147212338,3976.3361692878548],[3360.832881924681,4029.745090866578],[3346.827624861996,4038.8716227480145],[3346.740980468345,4038.934958897534],[3346.66162286731,4039.0072160075315],[3346.5904661567824,4039.0875617686775],[3346.5283299709004,4039.1750707007423],[3346.475930038915,4039.268734812947],[3335.8514575592903,4060.9095967086714],[3335.822286298204,4060.974805074122],[3332.121786634471,4070.108770331706],[3275.2500027890737,4135.736926566289],[3248.9958724477992,4163.20809849158],[3248.94233508378,4163.268864058277],[3239.933382357522,4174.369854157288],[3239.85385053485,4174.483038451769],[3218.1324131737997,4210.4503897668055],[3204.502973648996,4225.793714440814],[3204.4434927415823,4225.867429780817],[3204.3912531653687,4225.94644216197],[3204.3467236101615,4226.030042690744],[3198.118481080197,4239.189757386456],[3120.2598100817554,4305.973082196173],[3113.877910189802,4309.848587131972],[3076.486506532875,4330.002654658241],[3076.4042806109146,4330.052209862868],[3076.3271856468577,4330.1094216367],[3050.9303828171737,4350.9188989069235],[2985.390519021069,4396.305479238876],[2962.1053523387022,4412.173639046252],[2962.0084669968946,4412.24875737853],[2925.2678929470144,4444.528262141879],[2901.713807973279,4460.0685279406425],[2901.6426977434485,4460.120064331818],[2901.576383390145,4460.177640984621],[2901.5153763737208,4460.240813829649],[2868.7719685373854,4497.271226010552],[2863.976116343857,4501.233040073325],[2863.9023372361376,4501.300467174853],[2863.835657486514,4501.374922408023],[2863.776743210399,4501.455661982125],[2856.653104912527,4512.319747088508],[2856.604899120338,4512.401473981982],[2856.5646562186407,4512.48740181438],[2847.523700552819,4534.448495079528],[2837.3639835567838,4555.983891982742],[2803.467146152007,4600.836336237221],[2790.780805020737,4611.449870488774],[2790.712001914737,4611.513126438684],[2790.6494048938744,4611.582529576749],[2790.593560754556,4611.657473653887],[2790.544957305536,4611.737304019899],[2790.5040191068165,4611.821323341961],[2790.4711037610377,4611.908797695954],[2790.4464987897554,4611.998962977423],[2790.430419121886,4612.091031576171],[2766.019464658693,4804.560447416546],[2687.6990048705247,4782.441611210936],[2679.670875209528,4774.905819397262],[2679.5957834146957,4774.841993277526],[2679.514773718301,4774.78586859221],[2679.428632931169,4774.737990455195],[2679.338197700106,4774.69882388534],[2679.2443463819245,4774.668749289959],[2679.1479905123592,4774.6480587701],[2628.53751163912,4766.365129192635],[2628.432861606894,4766.353617930018],[2628.327581312747,4766.353172872452],[2591.9678920873052,4768.115728546683],[2504.5681033484975,4752.616987884184],[2504.480035070825,4752.605377564334],[2504.3912839283175,4752.601628462749],[2504.302550240358,4752.605770162919],[2504.2145341886007,4752.617769983427],[2487.7056096822125,4755.620715395738],[2487.6110195648707,4755.642714174159],[2487.5190093798647,4755.673784455872],[2487.4304468970136,4755.713633209704],[2487.3461673700435,4755.76188461263],[2487.266965659109,4755.818083594255],[2487.193588734281,4755.88170012869],[2487.1267286306957,4755.95213423335],[2487.0670159218053,4756.028721627532],[2487.0150137722926,4756.110739997405],[2486.9712126267304,4756.197415808323],[2486.9360255840843,4756.287931600207],[2482.9088247774357,4768.35206329772],[2462.673719924686,4819.321093447533],[2399.493198193338,4864.792257330434],[2399.4155371580714,4864.854231630661],[2399.3444095107616,4864.923606756429],[2399.2805174271184,4864.999697833008],[2399.224491653005,4865.081753685464],[2399.1768852776804,4865.168964254293],[2399.1381682736765,4865.260468592377],[2399.1087228572032,4865.355363364288],[2399.088839714893,4865.452711764065],[2399.078715134126,4865.55155276341],[2399.0784490652736,4865.650910599007],[2399.08804413498,4865.749804405307],[2399.1074056202356,4865.847257897681],[2399.136342383483,4865.942309010352],[2416.283335753462,4913.623197239528],[2416.8400234354026,4994.934846185545],[2416.8452461475254,4995.030297473023],[2416.8595591441745,4995.124813943175],[2416.882831629966,4995.21753188323],[2416.914850935689,4995.307604015791],[2416.955324461723,4995.394207241444],[2417.0038823518807,4995.476550160425],[2417.0600808732356,4995.5538803046165],[2417.123406471055,4995.625491013759],[2417.193280461778,4995.690727893079],[2417.269064321155,4995.748994793297],[2417.3500655192274,4995.799759258367],[2417.4355438488224,4995.842557391186],[2417.5247181897303,4995.8769980927855],[2417.6167736467596,4995.902766636286],[2417.7108689964316,4995.919627542939],[2417.806144374269,4995.927426733985],[2424.728644374269,4996.161926733987],[2424.7306815146517,4996.161993663807],[2508.4707474735847,4998.827825532716],[2602.581716520434,5035.138848816935],[2699.836499468081,5159.009453928608],[2702.5095809478066,5178.4314913482485],[2615.6690758733093,5286.227933740624],[2535.602211287143,5274.067502142084],[2535.502725038709,5274.057448945435],[2535.4027321597655,5274.05738148538],[2535.30323243715,5274.067300436425],[2535.2052207268393,5274.087106623141],[2535.109677006797,5274.116602011777],[2535.0175565785894,5274.155491690319],[2534.929780515723,5274.20338681719],[2534.847226454219,5274.259808509113],[2534.7707198174976,5274.324192629267],[2534.701025563317,5274.395895427849],[2534.63884053528,5274.474199978664],[2515.8283796695855,5300.75920103734],[2464.6743872558623,5369.86835793211],[2388.3203019900166,5417.501964017367],[2388.242264781933,5417.5559543235095],[2388.169696481122,5417.61709842151],[2388.1032505476437,5417.6848457235],[2388.043525311116,5417.758586181354],[2387.9910585828998,5417.837655780019],[2387.9463228132386,5417.921342516816],[2387.909720836957,5418.008892812847],[2372.71966282169,5459.814218003686],[2372.6933852108536,5459.8977695972035],[2372.674519357326,5459.983300066518],[2372.663209988268,5460.070153274761],[2369.3241091368327,5498.948013794731],[2346.834154563837,5550.95186202586],[2346.8001525902373,5551.042227808618],[2346.7750238418503,5551.135451490732],[2346.759002571604,5551.2306640308825],[2339.81847798144,5609.569568948915],[2339.8117542851955,5609.66408981804],[2339.8140074876083,5609.75882273666],[2339.825217356266,5609.852917059741],[2339.8452832332428,5609.945527876455],[2339.8740249389475,5610.035823596977],[2339.911184390027,5610.122993419662],[2401.6741008450213,5737.864936598659],[2389.6172551578957,5770.752493993938],[2305.942910354993,5849.081284630581],[2254.5664806927525,5887.281729176608],[2254.4965692916535,5887.338784718983],[2227.871663164333,5911.147806097112],[2118.054161352502,5922.571468679557],[2117.957587941574,5922.586313795602],[2117.862924264895,5922.6105127519095],[2117.7710740577645,5922.643834525854],[2117.682914195848,5922.685961001086],[2117.599286323817,5922.736490004523],[2117.520988820339,5922.794939145816],[2117.448769176111,5922.860750422633],[2117.3833168577175,5922.9332955478],[2117.3252567254276,5923.011881947434],[2117.275143067781,5923.095759372799],[2117.2334543099023,5923.184127062795],[2117.200588446074,5923.276141388656],[2117.1768592401636,5923.370923907906],[2117.162493230182,5923.467569750683],[2117.1576275655693,5923.565156258339],[2117.162308697859,5923.6627517918905],[2129.904681579215,6054.885780531759],[2129.92098884904,6054.9929371233275],[2129.948797994332,6055.097699299727],[2129.9877823003994,6055.19883626649],[2138.8605305691967,6074.954176289757],[2076.871358463988,6203.950115449471],[1975.9434990468064,6270.718364152529],[1975.8649426304812,6270.7760256039055],[1975.792371470594,6270.841059289586],[1975.7264746995052,6270.912847652715],[1975.667878069891,6270.990708994333],[1975.6171380126223,6271.073903946758],[1968.9929899647777,6283.230527733714],[1968.9686501738654,6283.2781889148055],[1946.4200707585808,6330.51121982285],[1926.7229136957953,6362.652234496145],[1916.938152369285,6376.309829805987],[1913.3146357410042,6381.2459823559775],[1913.2536637004919,6381.339586103373],[1913.2035123066312,6381.439406386606],[1913.1648074112672,6381.544197523281],[1903.6882986953785,6412.405731364237],[1891.3020664467426,6440.6376567010475],[1869.907199398407,6471.702015879419],[1869.8875040903417,6471.731732952547],[1849.68334036416,6503.42947466038],[1815.9086909425212,6556.509418846179],[1799.0260613187604,6573.859647677822],[1765.2660291180864,6599.164994338875],[1765.1987813326032,6599.220126062557],[1765.1365774387273,6599.280891572472],[1765.0798878080968,6599.346831373712],[1743.3791299001732,6626.929242898925],[1743.3236443644562,6627.007166480293],[1743.2758582905367,6627.090035173696],[1743.2362089558374,6627.177090670162],[1722.4818175872535,6679.210638212816],[1719.339978631178,6683.431846877605],[1719.31598800873,6683.465510384738],[1716.2689649650993,6687.933682100728],[1716.213506121589,6688.025163894031],[1716.168137291222,6688.12204666371],[1713.136991660154,6695.614292618404],[1713.101977562189,6695.716362951887],[1713.0781655806095,6695.821611845311],[1713.0658329902265,6695.928813744772],[1711.3450762334696,6724.309750681707],[1711.344672751555,6724.316819609566],[1707.3942087534374,6798.119775926175],[1636.8626456712627,6890.09484500583],[1636.809173016904,6890.171788665613],[1636.7631367867402,6890.253399431186],[1636.7249411628304,6890.338960788463],[1636.6949214894432,6890.427721538543],[1612.490170285591,6974.835779323453],[1612.4686923882957,6974.92641615465],[1612.4557410395794,6975.018658215599],[1612.4514286096855,6975.111705183257],[1612.4557925147017,6975.204749750937],[1612.4687948919245,6975.296984632778],[1612.4903229283705,6975.387609568025],[1621.3930093939657,7006.3688222721785],[1612.0077199641898,7064.140697434215],[1569.5192282727428,7116.331820854129],[1569.464137395974,7116.406287568642],[1569.4161733085373,7116.48553239554],[1569.375747556514,7116.568875390729],[1569.3432070048332,7116.655601446617],[1569.3188308610695,7116.744966427951],[1569.3028282797584,7116.836203556714],[1569.2953365677886,7116.928529991312],[1568.437742582826,7141.679657810859],[1568.438908710661,7141.7736876401605],[1568.4489021917611,7141.867192180094],[1568.4676346540866,7141.959344572943],[1568.4949404470601,7142.049329917987],[1568.5305781064105,7142.136352477641],[1568.5742324894331,7142.219642714137],[1600.2156610608617,7196.248214142707],[1600.2666584782091,7196.326635663387],[1600.3247606790571,7196.399948730015],[1600.3894592301338,7196.467511804031],[1617.2643712858367,7212.514752466605],[1588.1071808233517,7390.6877791448915],[1535.120637693796,7455.80588433573],[1535.0635611480516,7455.883365498236],[1535.0141966146589,7455.965974247533],[1534.9730012616526,7456.0529455388205],[1534.9403566017766,7456.143473925523],[1534.91656495927,7456.236721018584],[1534.901846670024,7456.3318232508345],[1534.8963380410366,7456.427899874548],[1534.9000900880662,7456.524061118095],[1534.91306806317,7456.619416426178],[1534.935151776508,7456.713082707318],[1534.9661367094282,7456.804192512221],[1535.0057359085265,7456.891902067277],[1535.0535826431405,7456.975399088806],[1535.1092338016651,7457.053910305663],[1535.172173995236,7457.126708620552],[1535.2418203307802,7457.1931198437205],[1535.3175278092224,7457.252528936672],[1535.3985952988644,7457.3043857080775],[1535.4842720286083,7457.348209909129],[1535.5737645408951,7457.383595681157],[1535.666244039965,7457.41021531431],[1603.8029004551804,7473.517224598473],[1645.7591210599235,7530.871328401695],[1641.243976518752,7653.4642254895625],[1641.244719414679,7653.554312008417],[1641.2535669148542,7653.643966090946],[1649.5201232135385,7710.884434907228],[1644.5299837148366,7734.431212036569],[1644.514315159001,7734.528623721882],[1644.5083221686903,7734.627105314804],[1644.5120630829088,7734.725698142323],[1650.520337939531,7803.392425243767],[1649.6044335859801,7808.226901917324],[1649.5900208912917,7808.334817211927],[1649.5874253098705,7808.443659756617],[1649.5966776082762,7808.552139393193],[1651.1834979567357,7819.8493419839],[1651.310256693697,7839.658367412468],[1651.3108065443478,7839.685737165177],[1654.2228235577622,7925.870860107981],[1642.759401992722,7971.314845768135],[1638.1469120743595,7983.1092084784195],[1616.771883945864,8026.440043768114],[1616.7255649508004,8026.550039439949],[1616.6926804728557,8026.664770029751],[1615.0872645797742,8033.8632878289045],[1600.406461724284,8071.542842390183],[1600.3801318052904,8071.619460103985],[1597.7418965111729,8080.44455120237],[1597.7198963759377,8080.53248696287],[1597.7059494619973,8080.622053628078],[1597.7001703670142,8080.712515255225],[1597.7026065761008,8080.8031285479055],[1598.3475167557415,8089.717664078615],[1598.3600082556707,8089.818622240223],[1607.58017942056,8142.275403510103],[1602.716547404005,8170.985474617346],[1602.7045113073489,8171.089107813253],[1602.7033380251185,8171.193431014533],[1603.6975445266153,8195.462883965522],[1604.5663088148613,8220.76916484676],[1593.9689285037641,8285.169389618202],[1587.6799584280154,8296.97582290916],[1587.637691680003,8297.065653378238],[1587.6045403588737,8297.159232120854],[1587.5808312037561,8297.255636825748],[1581.708809190096,8327.54501425192],[1581.7068465971533,8327.555433106061],[1570.0226825958146,8391.44325457152],[1545.4216567559931,8422.96038415049],[1545.3912174392688,8423.001508068466],[1537.3496937405193,8434.467922723888],[1537.2877921024633,8434.568298707562],[1537.2381375040977,8434.675263990497],[1535.5785772109596,8438.883791638733],[1535.5512760814017,8438.962480895463],[1535.5306180526768,8439.043169163813],[1535.5167464367764,8439.125296680957],[1531.7680960696655,8468.798198978575],[1527.5160964689117,8486.729422972838],[1517.4374568805144,8524.91892456744],[1438.6720130817625,8595.90104562118],[1438.6032780853195,8595.969304470167],[1438.541470050373,8596.043893591474],[1438.4871689693193,8596.124113057924],[1438.4408843910244,8596.20921010848],[1438.4030506393349,8596.298386211973],[1436.1934074034434,8602.29971778217],[1423.8292824066882,8627.623353282695],[1387.700563038638,8674.79192737809],[1387.6797775669186,8674.820070798578],[1382.9617772586935,8681.44777392137],[1259.985219219827,8726.116889536412],[1259.8975699719094,8726.15352743479],[1259.8137928481954,8726.198317163527],[1259.734643914293,8726.250854507582],[1259.6608374676641,8726.310665331805],[1259.5930395912862,8726.37720985988],[1259.531862142434,8726.449887545657],[1247.6893462020553,8741.957799122953],[1216.052890722233,8774.199625884867],[1215.9738528834393,8774.290536051005],[1215.9063200714818,8774.390290537507],[1209.0103300965445,8786.030140161567],[1208.9659056429832,8786.113951338843],[1208.9296221795405,8786.201594683092],[1208.9018061792156,8786.292281593043],[1208.882707926082,8786.38519608189],[1208.872499263271,8786.479502119419],[1208.8712720467536,8786.57435115447],[1208.879037318834,8786.66888975008],[1208.8957252087937,8786.762267262573],[1216.1946516340474,8818.756578458948],[1218.269478428458,8843.567335211354],[1218.270525032373,8843.579024148656],[1223.503510338239,8898.399887597992],[1219.8591184196423,8917.283504453471],[1219.846369594092,8917.369509151884],[1219.8411395186529,8917.456296172877],[1219.8434677292466,8917.54320946379],[1219.8533366261363,8917.629592017447],[1224.914771317779,8949.553273349533],[1225.0287690058296,8968.043040330344],[1225.0368137050193,8968.163612471571],[1230.4489877937692,9010.523080802614],[1210.2468555603948,9065.130895395801],[1210.2168880983359,9065.22631421801],[1210.196601793904,9065.324249228433],[1210.1861995669533,9065.423720802706],[1210.1857854688838,9065.523733946526],[1210.1953636418357,9065.623288248376],[1210.214838277257,9065.721387886444],[1210.2440145742562,9065.81705158963],[1210.2826006881578,9065.909322453006],[1240.3139055995796,9128.70127117834],[1240.3477981271349,9128.765956442527],[1242.963829855706,9133.343936280926],[1253.6906551303643,9225.01765070887],[1247.5244993268714,9246.147566050617],[1247.5003000283143,9246.250417017753],[1247.4870878142865,9246.355247189422],[1247.4850101849875,9246.460886249186],[1247.49409033492,9246.566154850243],[1247.5142268939487,9246.669877781565],[1252.8306890934753,9267.969364018954],[1252.8653224322993,9268.080252796954],[1252.9125824211042,9268.186376699552],[1268.3701790554792,9298.090461315735],[1273.0445274638898,9331.816757095958],[1274.9688287643005,9361.032389726557],[1274.979396061883,9361.12571619647],[1274.9986725648992,9361.21763961131],[1275.02648822572,9361.307349068882],[1275.0625976685558,9361.394053197404],[1275.1066823540375,9361.476987136599],[1275.1583533892106,9361.55541928491],[1275.2171549581542,9361.628657753316],[1278.370379004705,9365.198763741322],[1284.3907422819132,9490.558006773663],[1284.4002000021007,9490.655313803258],[1284.4191144275424,9490.751232263367],[1284.4473047721456,9490.844845355137],[1284.4845015895946,9490.935258314668],[1288.1740972914972,9498.788617361977],[1303.4213283518538,9532.958586361383],[1304.2919724617043,9589.011086336555],[1304.2977165726531,9589.10369919454],[1304.3120203238313,9589.195380921055],[1304.334760557992,9589.285342123943],[1304.36574147862,9589.372808224985],[1304.404696335767,9589.457026129136],[1304.4512897228042,9589.537270708759],[1304.5051204643198,9589.612851047092],[1304.565725070294,9589.683116387121],[1338.841613953872,9625.88157292711],[1367.335318025468,9687.029331687285],[1367.3768437449999,9687.108908683058],[1367.425337826012,9687.184441508674],[1367.4804095573363,9687.25532160495],[1382.7408163496482,9705.17454534072],[1382.7864008203112,9705.224541874682],[1396.4318989343021,9719.209701146363],[1407.2346117911427,9733.21569441488],[1407.2409082713475,9733.223772086883],[1416.758301566204,9745.305401338454],[1440.1033704740394,9805.424204229783],[1440.1403567771881,9805.507889303359],[1440.1848369487993,9805.587843618398],[1440.2364386374318,9805.663397863085],[1440.2947298761565,9805.733919559378],[1440.3592226986289,9805.79881835759],[1440.4293772239425,9805.857550978322],[1475.191181308414,9832.352069430102],[1516.6790809313957,9869.30395905013],[1516.7632582586848,9869.371164402643],[1516.8541757319874,9869.428925937282],[1516.9507784920625,9869.47657348267],[1517.0519457168014,9869.513554213794],[1526.9343850572866,9872.533434694751],[1527.0241896242496,9872.556385327009],[1527.115733436645,9872.570922251734],[1546.260035380403,9874.707045842035],[1574.3235979524518,9881.746112669363],[1574.420845914179,9881.765437404427],[1574.519529553177,9881.775036927298],[1574.6186787489357,9881.7748168688],[1574.717318804236,9881.76477939224],[1574.8144800270213,9881.745023172152],[1574.9092072630629,9881.715742424258],[1575.0005692857098,9881.677224996212],[1575.0876679504167,9881.629849537887],[1596.0344647437244,9868.85158533433],[1596.0539534016082,9868.839386983489],[1631.7668649276675,9845.910374983388],[1715.3609499245656,9844.265995423862],[1813.7024418940546,9907.807272342216],[1822.2996626600607,9946.804090175945],[1819.3920689514005,9972.339748010088],[1817.5264125336794,9983.500581153008],[1817.5147921977225,9983.601223820135],[1817.5134146460737,9983.702525750055],[1817.5222940178935,9983.803447181923],[1817.5413391754994,9983.902952260316],[1817.5703546398001,9984.000019667232],[1834.6768407550612,10032.184015281953],[1836.2339756427828,10039.245328184259],[1838.381571149067,10067.641372166676],[1838.3944243144656,10067.742466092033],[1838.4174996086026,10067.841726942348],[1838.450557390265,10067.938123875267],[1838.4932543482546,10068.030655790752],[1842.8708223347278,10076.371983112158],[1842.9189874895044,10076.454022527101],[1842.9747305357503,10076.531113775205],[1843.0375469795129,10076.602559153867],[1843.1068683101325,10076.667712057564],[1843.1820671454611,10076.725982829861],[1843.2624629098898,10076.776844100008],[1843.3473279937932,10076.819835555823],[1843.4358943386517,10076.85456810968],[1843.5273603882458,10076.88072741989],[1843.6208983430206,10076.898076735597],[1843.7156616519587,10076.906459039463],[1843.8107926741611,10076.905798468722],[1843.9054304407964,10076.89610100177],[1843.998718447167,10076.877454404053],[1849.8758404003918,10075.40887270162],[1855.6857055794699,10074.213700058448],[1855.778069806619,10074.19005921904],[1855.8677628412308,10074.157729677023],[1855.953969370056,10074.117005309223],[1856.0359057723215,10074.06825630176],[1874.205519082565,10062.04962425521],[2017.010470824073,10121.087329464333],[2160.077488633301,10222.540523274862],[2164.5661218619857,10239.279996954627],[2164.596565574,10239.37450026118],[2170.5976170384038,10255.25451719032],[2177.3080401299153,10282.2318182835],[2177.3351407292294,10282.322291983322],[2177.370655752471,10282.40980550209],[2177.414268408018,10282.493578225089],[2177.465589673912,10282.57286290526],[2177.524161767907,10282.646952328578],[2177.589462230859,10282.715185622363],[2177.660908587024,10282.776954150218],[2177.7378635397026,10282.831706941024],[2177.8196406558855,10282.87895560356],[2177.9055104891872,10282.918278682933],[2177.9947070864628,10282.949325419913],[2178.086434820054,10282.971818879694],[2178.1798754847373,10282.985558422124],[2205.401698130405,10285.682591356966],[2205.4822469685805,10285.687300740225],[2232.048131893979,10286.166748948079],[2232.150373917295,10286.16336085521],[2232.251734821866,10286.149545011787],[2232.351153874898,10286.125445999374],[2327.8845659781127,10257.72284667493],[2348.738129454688,10256.69333928542],[2468.670188058093,10279.59517032357],[2525.4843219720115,10327.410585237105],[2546.111718556859,10385.941500144241],[2548.701031796448,10407.938265422226],[2548.722559814181,10408.057927188893],[2548.7584506267704,10408.174091914698],[2552.214959636734,10417.3434685187],[2561.625313349501,10443.054028527475],[2569.5413484639153,10466.588206245924],[2571.2156967365004,10476.772030349835],[2571.234575290582,10476.861232833164],[2571.2615002459656,10476.948345000017],[2571.2962477624956,10477.032642644892],[2571.33852896729,10477.113424960762],[2576.789846739883,10486.464558673686],[2588.018098363197,10527.631707319448],[2588.046808294056,10527.7204415078],[2588.0836599930703,10527.806115124462],[2588.128332923562,10527.88798297892],[2588.1804385196524,10527.96533298324],[2588.239523566016,10528.037492345824],[2610.635203008524,10552.924530673352],[2610.714970145689,10553.003727113291],[2610.8031213600625,10553.07347118598],[2610.8985428723095,10553.132881683967],[2628.9918557722176,10563.032521880381],[2662.640503372616,10583.968096406958],[2662.7362584599714,10584.020647756977],[2662.8371738297287,10584.062442126095],[2673.3970192035827,10587.774175434846],[2715.4988146662627,10613.725074910422],[2715.5730835134013,10613.766600517158],[2715.6506136959024,10613.801662012726],[2715.730843876817,10613.830005543565],[2739.451405502267,10621.090622279406],[2829.696759594545,10701.284367675147],[2854.277547055705,10741.18924063434],[2854.360824503726,10741.305039330711],[2866.5581042142394,10755.938590625783],[2866.6279843327925,10756.014155919956],[2866.705261494192,10756.082138160124],[2866.7891170748353,10756.141817186988],[2866.8786627636546,10756.192560800047],[2866.9729499723067,10756.233831454718],[2880.707356496175,10761.420815489244],[2880.8022593370824,10761.45134495312],[2880.8997303529964,10761.472273365232],[2880.9988008187333,10761.48339272652],[2881.098486112823,10761.484592526129],[2881.1977955032626,10761.475860839731],[2881.295741994005,10761.457284448046],[2881.3913521343143,10761.429047974347],[2881.4836756935006,10761.391432049582],[2881.5717951048805,10761.344810523278],[2930.6218458834855,10732.175573894978],[2930.741902134258,10732.091701430474],[2940.149915763738,10724.43570067328],[2940.227595147098,10724.365412607982],[2940.2974950679272,10724.287383768502],[2940.358848409634,10724.20247048224],[2940.4109818500474,10724.111604629945],[2940.4533232507924,10724.0157834188],[2940.485407936221,10723.91605843856],[2960.6318820206284,10647.837446535403],[3143.3763840789893,10612.744862759517],[3190.5786212953585,10701.47289773858],[3202.448694142915,10757.470984306467],[3215.444100044651,10834.507105152357],[3217.5207621116456,10859.001664984826],[3217.533591004585,10859.09757024365],[3217.555628720531,10859.191786687226],[3217.5866689335903,10859.283432223601],[3217.626421032898,10859.371648830684],[3217.674512843433,10859.455610589383],[3217.730494110469,10859.534531416199],[3217.793840715037,10859.60767242285],[3217.86395958094,10859.674348834036],[3250.2107771671604,10887.581009396596],[3279.3012811048284,10919.205480496868],[3296.8990845035437,10951.08328886111],[3296.9452282011657,10951.15877803576],[3296.9978636750902,10951.22989317623],[3297.05657890239,10951.296077603423],[3297.1209142687026,10951.356813235148],[3297.190366166024,10951.411624641607],[3351.0620687897417,10990.186609156424],[3357.603838721977,11000.987446645535],[3357.6536109464287,11001.06188465927],[3357.709842488407,11001.13157185969],[3357.772082466977,11001.19594947446],[3357.8398318238014,11001.254501305051],[3357.9125473247414,11001.306757865776],[3369.423391195813,11008.82105242233],[3417.3301507568563,11093.29331764027],[3417.3797711309035,11093.37203549042],[3417.4364936054467,11093.445800267478],[3417.4998270386477,11093.513973265739],[3417.569223046483,11093.575964196925],[3417.644080751026,11093.631236301313],[3417.72375198326,11093.679310995354],[3417.8075468953634,11093.71977201559],[3460.4543736797755,11111.916541748478],[3506.862508280986,11176.03331567935],[3509.102080788065,11184.690866090496],[3509.1280935588125,11184.77570362474],[3528.899179944212,11240.331734956413],[3533.6902441999155,11256.765913046767],[3533.7189025327725,11256.850090194144],[3533.754925347819,11256.931388711833],[3533.7980278093355,11257.009165764965],[3533.847869102185,11257.082806363209],[3542.719064156234,11269.01085858024],[3555.43436316632,11289.94568584662],[3555.4984794474967,11290.038917329402],[3555.5727175689444,11290.124308829083],[3555.656127059483,11290.200767077702],[3578.340020150416,11308.745529379072],[3586.9867520435914,11315.86876859851],[3632.639030729257,11386.21787254812],[3637.4638137487623,11403.842592419764],[3639.0803473634464,11415.03154077238],[3640.877842247829,11431.049787142352],[3640.893725558267,11431.147441196807],[3640.9191809328404,11431.243047775068],[3640.953959199225,11431.33567102317],[3640.997719927095,11431.42440428975],[3641.050034760456,11431.508379000896],[3641.11039161065,11431.586773162262],[3654.7597211678744,11447.608402493335],[3672.7797156627644,11493.962332142133],[3672.7937410976874,11493.99652573031],[3701.6950911459035,11560.908001140144],[3701.7270642312187,11560.97506409704],[3701.7639280658836,11561.039568174941],[3726.576739237711,11600.939203876218],[3726.635328809664,11601.023447783014],[3726.702243190397,11601.101243923182],[3726.7767777866197,11601.171773120965],[3726.858147765895,11601.234292719866],[3726.945496320749,11601.288144402652],[3735.7417926170456,11606.106662921171],[3735.796823206,11606.134635528504],[3755.028039330448,11615.174289778572],[3779.2790363786517,11628.950579005628],[3779.411516276024,11629.013469978609],[3829.465036809545,11648.417583702721],[3829.5615227527173,11648.4494512543],[3829.6607445396094,11648.47136278276],[3829.761677697525,11648.483092049859],[3829.863280083729,11648.484517949995],[3829.964502645651,11648.475625760642],[3868.1810860622345,11643.150430955446],[3868.2824310784504,11643.130932329414],[3868.3812267314684,11643.101092294666],[3868.4764207479257,11643.061228677674],[3868.5669992155213,11643.011766066169],[3868.6519973822074,11642.953231286858],[3868.7305099317673,11642.886247794186],[3886.3319204835757,11626.22555607208],[3892.1442854171946,11621.596718509056],[3906.042850417999,11610.74034893341],[3906.050116203752,11610.734619200268],[3922.966546340051,11597.267071867456],[3938.3014842406496,11585.649467414845],[3938.3920496955925,11585.571940592128],[3938.472745548097,11585.48418702093],[3970.264167596368,11546.481121137042],[3970.324986511873,11546.39812766082],[3970.3769553923153,11546.309323983845],[3970.419524050559,11546.215650257998],[3986.361252185438,11505.724027497197],[3986.3988644096617,11505.608275077659],[3991.164182523817,11487.198064779492],[4040.3976929303285,11420.498679465523],[4061.536794548569,11399.325962256808],[4061.597119538766,11399.259875321717],[4079.6149669376246,11377.65410279042],[4140.8671618623175,11341.627732498411],[4157.792522399622,11332.184733647606],[4191.051725881308,11322.155738735533],[4191.148876620535,11322.120880268296],[4196.978159731789,11319.682843915896],[4215.047884378901,11313.549081962119],[4224.496820295757,11311.005088172744],[4224.589403251061,11310.97526246298],[4224.678650563113,11310.936583090343],[4224.763717847331,11310.889416007207],[4224.843800267119,11310.834207469672],[4224.918140148565,11310.771479815463],[4224.986034148921,11310.701826521992],[4234.877397171864,11299.513634889065],[4234.936156860031,11299.440530724629],[4234.987809061601,11299.362242986863],[4258.268114571694,11260.197131148347],[4258.314417268974,11260.109647693276],[4261.420354712283,11253.465399878798],[4264.514112217588,11247.24533863443],[4264.555415356202,11247.15022565653],[4266.370627620353,11242.295508675397],[4266.401336862102,11242.19863334399],[4266.422055167965,11242.099141438963],[4266.432568561611,11241.9980605015],[4266.432768461897,11241.896434484144],[4263.792992244257,11187.920918907137],[4263.783202442949,11187.821961597665],[4263.763632862481,11187.724465849922],[4263.734477014484,11187.62939573982],[4263.6960232033325,11187.537691357615],[4253.837389389992,11166.960569381828],[4291.750787392903,11045.213884071432],[4297.9087437884,11041.389520686134],[4305.80216519091,11037.130043832987],[4305.891932009804,11037.075324114909],[4305.9754580283525,11037.011482642916],[4332.104729803697,11014.769859606266],[4332.196607499783,11014.680914516373],[4354.85470734863,10989.747851360557],[4354.859906295747,10989.742085608379],[4377.991030561949,10963.888042857598],[4382.68422477209,10960.103565826254],[4406.92677600469,10945.384654207024],[4407.002698178594,10945.333665430866],[4407.073644540051,10945.27595368526],[4407.139021691436,10945.212001673084],[4407.198282816178,10945.142344291],[4423.814974636338,10923.695988412053],[4447.642007840545,10895.290942693862],[4447.695621066749,10895.22098450139],[4447.742865973274,10895.14657720655],[4447.783375536806,10895.068298844402],[4456.691791912935,10875.8205238928],[4465.651572854703,10863.100341845335],[4465.683893302499,10863.051476291805],[4476.070476346391,10846.301356498172],[4495.068788500651,10819.255965214312],[4505.017284845988,10808.497386222012],[4505.036370031887,10808.476146487521],[4516.05329310881,10795.857684949058],[4516.086596072373,10795.817467909228],[4539.215058600943,10766.35398079828],[4565.528094891899,10734.781668540321],[4573.611215055191,10725.48913743322],[4573.669681640963,10725.41514806197],[4588.057151269564,10705.328823868982],[4588.099445614183,10705.264711465717],[4588.136694601435,10705.197542091833],[4601.65614491856,10678.444969857865],[4601.685919540146,10678.38045426083],[4611.893062238461,10654.0246615158],[4617.820690682757,10644.307780393423],[4617.86652545418,10644.223868352346],[4623.847098269715,10631.909683886326],[4623.855464319993,10631.89202075357],[4633.502682395304,10610.998608832842],[4640.912462772305,10599.142571192326],[4645.299609183921,10593.23491096745],[4645.3394144544,10593.177186595025],[4668.846461916902,10556.391980102318],[4668.891554039734,10556.313863060202],[4668.92942395201,10556.23200072726],[4668.95976356034,10556.14705910038],[4668.982326034661,10556.059729228267],[4675.823655264157,10523.598889214],[4685.462425761507,10498.701286046646],[4685.474887140257,10498.667280917063],[4692.741452637023,10477.668555181132],[4701.152418212585,10461.160360704298],[4711.923321083607,10440.475487515825],[4711.955267360794,10440.408118232963],[4711.982105282272,10440.33855595245],[4717.177387241139,10425.21667755582],[4738.7822477893715,10391.429075377926],[4738.833742930037,10391.338460539133],[4738.875526903781,10391.24297809017],[4748.492289606767,10365.722181861776],[4748.516453861886,10365.64979783452],[4748.535028087994,10365.575781912235],[4754.305173015529,10338.196216694845],[4754.305488380086,10338.194714565523],[4775.566270012779,10236.538466250948],[4824.119567423602,10154.859273230502],[4849.962538821934,10134.557539691994],[4862.599703269303,10126.001496633031],[4862.679500314532,10125.941447529698],[4862.75290997676,10125.873738706568],[4862.819200107422,10125.7990454551],[4862.877709564335,10125.71811272569],[4867.328120748546,10118.865727857268],[4867.361569032696,10118.810388613445],[4877.991660565876,10099.865422938388],[4878.032638471021,10099.783882534606],[4878.066012278224,10099.698945998583],[4891.807277976306,10059.417030866221],[4922.2657631154725,9981.645024220044],[4934.0545011731965,9968.121437435006],[4934.069695514009,9968.103588997461],[4940.925755095841,9959.856023308805],[4951.589970576988,9949.048052351744],[4951.655084090495,9948.975271991956],[4951.712788136441,9948.896487517006],[4951.762532405744,9948.812450274765],[4951.803842499723,9948.72396170744],[4951.836324454321,9948.631865708408],[4951.8596684972445,9948.53704057423],[4956.015046107048,9927.221650058034],[4965.936028566556,9900.355075068372],[4996.966263912294,9825.056582011295],[4996.992594078635,9824.985067498077],[4997.0134017934815,9824.911755546369],[5011.737853883056,9764.332817397382],[5035.299124274646,9735.685211105209],[5035.355775046853,9735.60926441554],[5035.404983808013,9735.528297091956],[5035.44630880076,9735.443035995097],[5035.479379041969,9735.354246531704],[5039.380065441207,9723.14123027648],[5042.492839433052,9715.832850608744],[5070.225627250708,9662.164923153594],[5070.26730793679,9662.073210363296],[5100.335332165032,9585.94825837064],[5140.07847061122,9518.882534287755],[5140.118028332198,9518.808933933855],[5140.151303481944,9518.732288061216],[5150.627877977041,9491.543787711966],[5160.666945803569,9471.637114721827],[5168.140620768033,9459.612666704277],[5168.156066436757,9459.586964378126],[5181.3029033872535,9436.94797810618],[5233.79183117627,9348.353920394906],[5233.838128449989,9348.266111002713],[5233.875491791565,9348.174144119448],[5233.9035530253595,9348.078925980057],[5233.922035638026,9347.981394857125],[5241.80346110057,9291.07813039209],[5266.875817197677,9249.465478083617],[5266.90408924173,9249.41534553678],[5274.357591762572,9235.261512438687],[5306.4630387717525,9187.062063493046],[5306.522208092855,9186.960833295867],[5306.569121412044,9186.853373061997],[5323.583557660728,9140.667598562524],[5323.595472698533,9140.633353535684],[5327.736421177001,9127.998298376688],[5342.328537333966,9100.126430264592],[5342.358252055026,9100.064600285656],[5347.5913296863655,9088.144866387294],[5352.435876385234,9079.753648193637],[5369.765300660994,9050.430292147588],[5388.100545698704,9024.108723784342],[5388.155107615432,9024.02107122107],[5399.42994632511,9003.632315460702],[5399.465789438467,9003.560902271913],[5413.512228353031,8972.542373652053],[5422.091761000947,8957.08085028393],[5431.902913029839,8944.734131166],[5431.96404916118,8944.648265804906],[5432.015807495465,8944.55644226967],[5432.057612972404,8944.459680764467],[5433.973128540236,8939.293987321555],[5455.435780329856,8904.16427950873],[5455.486576534765,8904.070168403006],[5460.410490544791,8893.650079095294],[5478.0466618125865,8864.454848957908],[5483.505546123611,8858.387783842396],[5483.558004933671,8858.324422249241],[5483.605078579519,8858.256963446209],[5493.295343601822,8843.07590860496],[5493.304356770786,8843.061520434405],[5519.153217263558,8801.008351895145],[5538.870785138039,8778.732795432534],[5542.53237332166,8775.287070766724],[5542.595330172198,8775.222216315595],[5542.652173896595,8775.151942167733],[5542.702440100668,8775.076822439372],[5542.74571812638,8774.99747083352],[5548.847498689395,8762.496717231397],[5566.29544804027,8736.247371231237],[5586.737323362805,8709.319988316518],[5590.934111886362,8703.882212745615],[5590.994772080913,8703.79427582344],[5591.045705277098,8703.700369641478],[5591.086330196684,8703.601565909803],[5591.116183205278,8703.498992232076],[5599.290540622333,8668.551955865774],[5609.48593675987,8630.23318004663],[5612.54446753149,8619.712861191045],[5628.996007968041,8565.29753175468],[5694.542774846394,8513.078554818461],[5730.371062421472,8508.369642040954],[5748.828235932454,8506.498254767681],[5758.027279846731,8506.787007714907],[5758.128587797893,8506.785051623925],[5769.881261239545,8505.961123440604],[5820.959367483709,8505.717626857042],[5838.7381896536335,8511.082074464814],[5838.763640904007,8511.08938769501],[5858.015481163046,8516.346333188803],[5858.117492277243,8516.36853933493],[5858.221262625602,8516.379989013834],[5858.325661175628,8516.380557431114],[5858.429550047815,8516.37023839137],[5858.531796917833,8516.349144365742],[5858.6312873581555,8516.317505266039],[5858.726936984633,8516.275665938841],[5872.019299134818,8509.61416141784],[5973.324009164395,8521.574268769988],[5973.415356005738,8521.580830468389],[5973.5069200748285,8521.579007679009],[5973.597933396229,8521.568815690132],[5973.687632613787,8521.550339985037],[6001.333054666052,8514.522503993501],[6150.632704048786,8515.986271272333],[6176.63647664793,8525.058631328926],[6231.024291361833,8550.66439417974],[6238.246329230062,8557.699130439109],[6238.308532257,8557.754852311113],[6238.375167727143,8557.80518974945],[6238.445770915157,8557.849791693601],[6247.988781667844,8563.33473793016],[6248.1129983053415,8563.395130923775],[6255.607200186229,8566.418209140467],[6270.152351441692,8574.294587912285],[6301.374951899664,8603.1490436377],[6301.43967636859,8603.20395404181],[6301.508824216391,8603.253177862278],[6301.581897273668,8603.296360470553],[6342.022916924033,8624.934034179525],[6382.613389653242,8656.493060954132],[6387.342657916521,8662.07765325868],[6387.362649904835,8662.100546233813],[6424.249525331327,8703.066388981555],[6424.332515151784,8703.148385545042],[6424.424490044063,8703.220158561655],[6452.881733378764,8722.8681754195],[6461.040654255296,8729.582759891955],[6461.1508052544405,8729.661535987947],[6479.556106094927,8741.023780840529],[6479.608143922169,8741.05378544513],[6514.084305148757,8759.575642795324],[6531.305921727747,8775.28247105248],[6531.365732460072,8775.332880433936],[6531.429300243204,8775.378460762893],[6563.594404650029,8796.587784918085],[6563.658199268315,8796.626514604692],[6563.724704637523,8796.660378712731],[6580.858837010136,8804.594301929288],[6649.008922644812,8849.374438823235],[6649.0613875710105,8849.406645165915],[6679.12330175004,8866.609605405214],[6704.517960765636,8898.38533783532],[6704.581247894867,8898.457185488252],[6704.651116193065,8898.522651256906],[6728.736181869395,8919.015431087859],[6743.036035816373,8936.295606279824],[6743.096286949141,8936.362100133369],[6743.162256066128,8936.422925351519],[6743.23341200831,8936.477592192028],[6743.309181854255,8936.525660497638],[6743.388955533084,8936.566743240064],[6811.171731602501,8967.709811238536],[6849.127264237393,9005.327138018163],[6904.736002331622,9086.451989829577],[6904.785904767721,9086.518657296945],[6904.841181122218,9086.580941556334],[6904.901448064409,9086.63841067859],[6911.348026788769,9092.292376665258],[6941.500227502632,9144.08534156938],[6941.565985987664,9144.184272131115],[6941.6430118668295,9144.274706905055],[6950.997904438959,9154.020661478413],[6951.054571805633,9154.075229146038],[6965.915157818913,9167.298935074794],[6987.268552651592,9187.160788429495],[6987.350246208701,9187.229037662208],[6987.438731792775,9187.288216175624],[6987.533006703309,9187.33765337096],[6994.625936645813,9190.58822692997],[7036.3434594886685,9224.224526226471],[7044.098436710142,9230.674142986529],[7058.990246221826,9243.875950415275],[7059.032027327442,9243.91100257247],[7068.078008184374,9251.089069505655],[7095.992409013824,9287.73363060625],[7118.652172863836,9342.973854518388],[7118.691282423905,9343.057879485104],[7118.738003158279,9343.137922759026],[7118.791933747401,9343.213296788223],[7150.581241923502,9383.552290498916],[7150.641929788728,9383.622359064173],[7150.708845043143,9383.686507092587],[7150.781412709339,9383.744183384473],[7164.660677447428,9393.761935250352],[7216.85537130335,9441.98498488973],[7216.918725986169,9442.038813910096],[7216.986332926851,9442.087194493397],[7217.057724868187,9442.12979226307],[7314.756645461544,9495.04609841701],[7346.892696552087,9564.573548423394],[7354.484371352843,9592.428525556388],[7354.521694759933,9592.53943272955],[7354.571718632936,9592.645220488319],[7371.952637079208,9624.441727359968],[7377.710493145728,9640.812559981205],[7377.741102607262,9640.889302238213],[7377.777942742068,9640.96325572098],[7377.8207620678495,9641.033915599879],[7384.485834832922,9651.07206528803],[7384.48979772261,9651.07798782508],[7407.848383170206,9685.719689584981],[7418.19897175392,9713.127435482802],[7418.22780292448,9713.195957416552],[7418.261644853709,9713.262148168375],[7439.124752456535,9750.577166863846],[7439.198687118754,9750.690616191909],[7445.670449243963,9759.286891062504],[7455.296670817287,9775.546426298526],[7470.3848232126265,9806.539267684633],[7470.4274743472315,9806.61776203025],[7470.4769604728335,9806.692136790416],[7470.532886666318,9806.761798418729],[7512.061212993428,9854.001753448545],[7512.081713384745,9854.024367821921],[7524.793443106607,9867.623913572212],[7545.578319002936,9909.05012375978],[7551.1834840414485,9928.070143732843],[7566.93756267089,9990.64269363911],[7566.95060339236,9990.689629475173],[7582.4930878417335,10041.771649017288],[7592.846754788209,10079.768777524532],[7595.781442711366,10123.718573327269],[7595.793519862233,10123.820452361248],[7595.815971707598,10123.92055785449],[7595.848561937408,10124.017836177143],[7597.510019124291,10128.24385422689],[7597.51615868218,10128.259101371194],[7622.653022924188,10189.233557394771],[7623.160368612165,10202.740057611496],[7623.169615779381,10202.843250490327],[7626.414657796188,10225.672494187806],[7626.431087673426,10225.759947928856],[7626.455226754755,10225.845594892857],[7644.644069259501,10280.875120509556],[7644.699902641324,10281.010063406733],[7658.948710792967,10309.383839011183],[7667.40565880494,10338.25444683275],[7667.409993582832,10338.268842996651],[7677.903806767058,10372.19381437208],[7681.004288970264,10385.892094474852],[7681.027881497142,10385.978254415833],[7681.059069015855,10386.061965116647],[7699.695092258946,10429.979424979436],[7572.034856144033,10561.387637400456],[7560.77401451213,10558.344200355376],[7537.96218273081,10550.687970596546],[7537.9093229356395,10550.67184039712],[7504.030540326944,10541.348883875382],[7503.92983341314,10541.326685751392],[7503.827375867338,10541.314977185946],[7477.2631569883615,10539.660586715825],[7455.020532491968,10532.551046646282],[7454.928259737439,10532.526342631625],[7454.834050860167,10532.510555389566],[7454.738765473723,10532.503828971578],[7430.6028672412785,10531.955946704062],[7400.754556039531,10527.326284812976],[7379.721393412756,10522.010484446395],[7379.648478217321,10521.9949230634],[7379.574606274389,10521.984837508708],[7344.981452063484,10518.56979448102],[7324.768140036446,10514.150350338039],[7324.681403723297,10514.13535187375],[7324.593684494275,10514.128038953038],[7324.505662010417,10514.128468237448],[7286.836599694519,10515.972065780177],[7254.027907336355,10508.540680137608],[7219.623129999747,10498.074140979208],[7210.211530503741,10493.862605522481],[7210.1179564677095,10493.82625297416],[7210.021209199063,10493.799465402451],[7209.922263678851,10493.782512761998],[7209.822117041235,10493.775565894866],[7209.721778524773,10493.778694808858],[7187.294842956813,10495.608024183553],[7170.028455385557,10496.275666906662],[7159.5872288040455,10496.381142851671],[7128.883862907077,10495.08540869209],[7082.993189816014,10487.607136644356],[7082.91315502505,10487.597387481344],[7082.832594970934,10487.594117676346],[7053.246360852996,10487.586956927047],[7031.616333590585,10487.514512526244],[6971.195243521382,10479.292389907474],[6965.081607066442,10477.640870580193],[6952.138891300922,10473.836334154552],[6926.986301327287,10461.982691510158],[6926.902648618093,10461.94780909066],[6926.816181201629,10461.920643950578],[6926.727609369583,10461.901419239563],[6915.143503209263,10459.931953436659],[6892.733261240818,10452.893733046189],[6892.669111402614,10452.875909162905],[6874.487741149777,10448.470620467484],[6849.52840366239,10436.03086708225],[6842.033722388089,10430.52075093821],[6841.955905984758,10430.468962408044],[6841.873593933174,10430.424666012614],[6841.787505430317,10430.388248789213],[6841.698392669702,10430.360028931313],[6841.607034269129,10430.340253008353],[6801.315959598043,10423.572329528979],[6776.461244405403,10414.048958893936],[6776.435820226602,10414.039610230266],[6743.108847245012,10402.294956754064],[6728.092242537772,10393.150952216873],[6728.013177683527,10393.107568858204],[6727.930525804724,10393.071485151127],[6716.368524065394,10388.633163872171],[6683.166974935726,10366.939993874174],[6683.074429955667,10366.886360383476],[6682.976685785837,10366.842918446104],[6662.335996130664,10358.962327313098],[6662.215363448541,10358.924811567394],[6634.858998131998,10352.279460035421],[6595.3382238919785,10334.106951054306],[6562.971453336806,10317.19636165515],[6562.966405459865,10317.193742547348],[6540.764971101997,10305.754459909565],[6534.5311203015635,10302.029338334138],[6489.137496994347,10274.757100859448],[6453.788795751387,10252.29613636698],[6413.877991600432,10225.819554353682],[6397.277933188156,10206.854413925541],[6397.215221354071,10206.78900112539],[6384.6591822671535,10194.827550003816],[6384.591693916338,10194.76878741567],[6384.519222624762,10194.716293309732],[6384.442348722182,10194.67048804406],[6384.361687793229,10194.63173841436],[6355.466243840889,10182.309611583389],[6320.978087721944,10164.536547554255],[6320.901528525522,10164.501097480132],[6290.217594099292,10151.83629867238],[6290.205726369646,10151.83148896258],[6275.618262222033,10146.02799517548],[6253.7473043142745,10133.68493093881],[6253.651097427525,10133.637254769139],[6243.858841161797,10129.423354193861],[6235.269641940679,10125.586939332954],[6235.181038034879,10125.552319312526],[6235.089545445441,10125.52627504222],[6234.99599210692,10125.509042201795],[6234.901224601992,10125.500776734634],[6234.806100500568,10125.501553436585],[6234.711480599471,10125.511365279117],[6234.618221132913,10125.530123472929],[6226.441545501857,10127.58380143912],[6214.492003773582,10129.77749845217],[6214.399682551849,10129.799014951754],[6214.309813509893,10129.829176567006],[6214.223204226,10129.867712260591],[6214.140632985723,10129.914275744308],[6214.062841788076,10129.968448590877],[6201.150546132421,10139.901342141266],[6193.323985151843,10145.149204932648],[6193.289039717382,10145.173733882533],[6178.430058649196,10156.084369560538],[6178.350678685977,10156.149170079856],[6178.2783469254255,10156.2217538403],[6178.213822871704,10156.301358691633],[6178.157784045821,10156.387148760163],[6178.110818871469,10156.478223225644],[6178.073420496403,10156.573625780153],[6178.045981614253,10156.672354669598],[6178.028790341125,10156.773373212414],[6172.685185785917,10202.116697193269],[6172.678436130719,10202.249411046272],[6173.440830842309,10250.877623009168],[6173.450067295401,10250.998442560185],[6173.473849004445,10251.117257989692],[6183.965907832124,10290.850515192784],[6185.945023170748,10302.721132592298],[6185.954898409862,10302.772152905729],[6189.663729972331,10319.580240762667],[6198.814084293524,10408.434792448134],[6198.829902046294,10408.536589886638],[6198.856109014244,10408.636219774822],[6210.927469816631,10446.48359033595],[6210.958913808857,10446.568645889882],[6210.9979335669595,10446.650503268163],[6211.044208224763,10446.728489343503],[6211.0973572578,10446.801962822996],[6211.1569436124355,10446.870319521591],[6211.2224772998325,10446.932997330407],[6233.664661993461,10466.527973466595],[6249.053963526497,10482.684670031882],[6262.911151439688,10503.746193757412],[6262.977280785543,10503.835474429192],[6263.052906110898,10503.916868156848],[6263.1370938869995,10503.989370205727],[6304.9759053219,10536.151828722644],[6316.410973447661,10551.63561141433],[6316.47153863382,10551.709889533737],[6316.538936328577,10551.778028594039],[6316.612547458436,10551.839402712021],[6316.691695877142,10551.893448143039],[6316.775654576327,10551.939668459227],[6316.863652363369,10551.97763910938],[6333.815754251902,10558.347254371807],[6365.520404474759,10570.345787978049],[6383.473949629594,10580.05757014362],[6383.49749093512,10580.069903061942],[6389.060945081831,10582.890934493244],[6437.81159366553,10616.220757158722],[6484.931817056744,10663.09529408079],[6485.001588280475,10663.158454767441],[6541.358232818082,10709.542574665995],[6557.2367145972485,10723.305824851916],[6557.286916884474,10723.346573193205],[6568.795220133572,10732.085967977364],[6568.838392558972,10732.116979110078],[6585.331353994015,10743.311718168523],[6588.944636450846,10746.291496872434],[6589.03265956029,10746.356340714361],[6589.127235309694,10746.411187949994],[6589.227233254831,10746.455383001758],[6599.679506738157,10750.407029998602],[6700.373881861772,10797.41793897759],[6712.32323622026,10812.3062084081],[6712.376053603975,10812.366850125753],[6712.433572956783,10812.423051709318],[6734.534128494702,10832.344609755095],[6734.569630907431,10832.375136083196],[6739.463771401639,10836.387899760955],[6739.53620467521,10836.442003648426],[6739.613228405655,10836.489344483947],[6747.368049765624,10840.78527225959],[6747.477062465072,10840.837320705448],[6754.778277039972,10843.79602515889],[6754.789706231964,10843.800575022067],[6774.398004595222,10851.467089538577],[6823.247176093887,10900.902873901712],[6823.32434260434,10900.973211719178],[6823.408422645906,10901.035119921473],[6966.095694696797,10995.018856805336],[6994.442494221119,11013.789183060186],[6994.448884099325,11013.79337918504],[7000.394612085377,11017.665393134472],[7000.483968909794,11017.717217830394],[7000.578195229213,11017.759547984022],[7000.676285607233,11017.791931913944],[7000.777193376247,11017.814024069337],[7000.87984180584,11017.825588717162],[7000.983135591984,11017.826502457518],[7001.085972544409,11017.816755540398],[7001.187255347462,11017.796451969703],[7013.591952580211,11014.635437405059],[7057.952515655813,11013.622024427632],[7215.715902128524,11039.9484780268],[7250.193133113207,11214.094727254143],[7250.217318641195,11214.191348533193],[7250.250995958347,11214.285084596899],[7250.29383096509,11214.375005526203],[7250.345398711972,11214.460219250514],[7250.405187615416,11214.539880397593],[7250.472604532953,11214.613198680167],[7250.546980647544,11214.679446736045],[7250.627578102666,11214.737967344015],[7250.713597322291,11214.788179943875],[7250.804184943175,11214.829586395932],[7250.89844228074,11214.861775922871],[7250.995434244575,11214.884429184907],[7251.094198615105,11214.89732144783],[7322.778984908669,11220.642919174608],[7368.060839996617,11252.445375572193],[7377.602943319256,11265.546483982396]],[[4812.197937827548,4974.1198635217015],[4812.230864960711,4974.026697198022],[4812.27282621299,4973.937235400176],[4818.287510525204,4962.60640351751],[4832.350335451667,4847.369514772505],[4800.765056943877,4801.803335895561],[4800.712526235089,4801.718869277112],[4800.668646811846,4801.629601807035],[4800.633852820893,4801.536416705268],[4800.608488516931,4801.440235953125],[4800.592804856543,4801.342011171147],[4800.586957015195,4801.242714203672],[4800.591002851928,4801.143327503339],[4800.60490233689,4801.044834410607],[4800.628517947393,4800.948209424509],[4800.661616028581,4800.854408560868],[4803.5306111921755,4793.854338081048],[4825.922324059656,4704.144508799383],[4806.353419289645,4662.043204719689],[4712.340555362126,4617.650413736221],[4656.330767331292,4627.846534737667],[4643.595510023767,4634.1947695985755],[4599.164707529377,4676.600187301615],[4529.759760484413,4791.476733029575],[4529.6976049706755,4791.567848021609],[4526.586258816829,4795.628232636994],[4526.523888047012,4795.7019615272775],[4526.454696306784,4795.769330401946],[4526.379328882616,4795.829710974685],[4526.298488655795,4795.882540132518],[4526.212929547302,4795.927325187435],[4526.123449486705,4795.963648471227],[4526.030882970612,4795.99117123069],[4525.936093280111,4796.00963678686],[4525.839964429749,4796.018872928809],[4525.743392923164,4796.018793519705],[4525.647279392231,4796.00939930012],[4525.552520197718,4795.990777881132],[4525.459999069764,4795.963102927252],[4525.3705788661655,4795.926632536822],[4525.285093525299,4795.881706834968],[4513.214531634816,4788.7740908709775],[4347.326640442297,4702.77899737791],[4280.516399179406,4726.445108793716],[4280.420976097646,4726.473648367064],[4280.323187315881,4726.492554078902],[4280.224002905843,4726.501638383211],[4280.124406784001,4726.500811163042],[4280.025386951031,4726.490080624477],[4213.934436995647,4715.975748529052],[4168.265750448521,4725.657523066863],[4055.200557458501,4823.226735266291],[4048.91348105696,4863.591358084221],[4042.1874827660113,4909.957301147269],[4146.747268123434,5050.295916466872],[4292.115065413178,5047.151635838371],[4299.51429944931,5044.273743535596],[4299.604115519804,5044.243624544975],[4299.696378600385,5044.2221395717015],[4299.790260714778,5044.209481423645],[4299.884919357366,5044.205763696043],[4299.979505053906,5044.211019752078],[4300.07316898478,5044.225202423483],[4339.8020313518655,5052.182016274192],[4356.715411850431,5051.581452045947],[4365.811863845072,5050.093122295134],[4365.874986774708,5050.08484777325],[4401.573554267821,5046.556913888951],[4401.687708184932,5046.552191059798],[4450.758845333169,5047.327973045361],[4450.886729433513,5047.33822556481],[4513.50515325285,5056.430311018363],[4513.592737617394,5056.447045332295],[4596.556577028193,5076.169280452176],[4596.638607065801,5076.192516402825],[4596.71835973434,5076.222655220545],[4596.795255324703,5076.259477831196],[4601.662343416618,5078.850767995207],[4638.047382145427,5089.059955270772],[4638.144828471589,5089.0927885733545],[4690.58196800482,5109.819960111814],[4743.397954836585,5108.489011746169],[4816.334273863938,5014.058193206153],[4812.1616177849,4974.511025988852],[4812.156117204332,4974.412365441622],[4812.16038059193,4974.313643693801],[4812.174366319296,4974.215824680329],[4812.197937827548,4974.1198635217015]],[[4026.074370558452,5692.797744085853],[4063.4930710815215,5659.784137429874],[4101.478366099442,5618.857458351772],[4101.512789461579,5618.822156340891],[4106.274255839717,5614.174128793424],[4133.2760492873695,5557.306670827292],[4135.967259561318,5544.251438008985],[4135.993680376608,5544.150319916205],[4136.030510592688,5544.052511617215],[4136.077347915755,5543.959081465037],[4146.242126100792,5526.080682126705],[4154.4109336382735,5476.2219162999845],[4155.479093394858,5449.4587674834975],[4155.049390958305,5440.004704372319],[4153.633213593993,5430.134330893704],[4153.623086568637,5429.996699847333],[4153.5636460091955,5416.463483064115],[4153.567632573543,5416.3697799348465],[4153.580380155682,5416.276862400013],[4158.051323908458,5392.152847872379],[4157.966981572916,5374.568053923668],[4155.4332025327885,5355.358461406612],[4155.424846604532,5355.24919547889],[4154.415727803938,5308.331210083002],[4153.130763058304,5303.271129540796],[4153.111321985454,5303.175052602624],[4153.1013808560265,5303.077533849005],[4153.101035191684,5302.979510311637],[4153.975606720052,5283.773703223555],[4154.976198450153,5218.542892073044],[4153.5659395747725,5209.734965279648],[4139.0252463127545,5171.909785972291],[4137.4467327608345,5168.110710483462],[4121.145994130166,5134.32644428977],[3951.455612609905,5050.408487117792],[3923.7408638895035,5065.689722729941],[3886.798732592715,5094.481213011665],[3882.184974336081,5099.0532143284745],[3846.1078388577475,5156.264963896138],[3848.068291146738,5253.785380519567],[3848.0646470116767,5253.893100712203],[3848.0494306372816,5253.999803015702],[3848.0228187909506,5254.104247877982],[3847.9851206203634,5254.2052219714715],[3838.972825816786,5274.870816899582],[3838.9337618088484,5274.950534879702],[3838.8877817644525,5275.026474254126],[3838.8352480501703,5275.098036548208],[3838.776574681738,5275.164657782867],[3833.89056603045,5280.239411575246],[3807.0715682795076,5334.529265080042],[3801.3443864644382,5368.109503779909],[3801.3236642117745,5368.203468990446],[3792.5057827102137,5400.671895135938],[3792.0723696695804,5412.82540881099],[3792.8157660326924,5432.556987781261],[3797.455337408214,5463.116566150984],[3797.466212513899,5463.236531981005],[3799.0057308227874,5514.301246899409],[3803.258974830221,5550.3755917727985],[3803.2657898682714,5550.481387951786],[3803.2613663470465,5550.587311078119],[3803.2457539837224,5550.692170652518],[3803.2191282499643,5550.794788129265],[3803.181788399748,5550.89401016219],[3790.4943901936326,5579.850003597594],[3869.3773558957873,5724.859493664968],[3894.6046859429757,5726.043286663909],[3894.681046746724,5726.049809890199],[3941.6134796523975,5731.877917012884],[3983.9041355051,5717.079067105284],[4016.8118137777765,5700.141769850734],[4026.074370558452,5692.797744085853]],[[3599.100237327396,5770.915961847117],[3598.9998584929253,5770.939686616633],[3598.897569790764,5770.9529454441945],[3598.7944594474084,5770.955597272118],[3598.6916244306126,5770.947613888202],[3598.5901587789917,5770.929080225871],[3539.770657906742,5757.016412881694],[3476.2244434974364,5745.684469356764],[3476.1368317377587,5745.664749949857],[3476.051342344703,5745.637250147721],[3475.968664762477,5745.602191727335],[3475.8894657589494,5745.55985742348],[3475.814384048378,5745.510588648579],[3468.3617524694305,5740.1263781222615],[3468.277806964677,5740.058546133791],[3468.201660529463,5739.982062496115],[3468.134200119478,5739.897818092664],[3465.5406778522715,5736.2743363112895],[3465.4880108106186,5736.192636742452],[3465.443524732674,5736.106209746127],[3465.4076399512323,5736.015871939614],[3465.38069552856,5735.922476892039],[3465.3629460527213,5735.826907059288],[3459.416502735387,5692.050094309859],[3433.657331555365,5653.639245353836],[3250.3117032305113,5660.410855306484],[3230.0684416417516,5686.800313001688],[3230.001137316794,5686.8792163718945],[3127.51364059425,5795.118657548569],[3126.300358135607,5799.02625179546],[3118.789768990111,5833.400461876132],[3132.6788252559945,5892.733839080977],[3299.609446162072,5939.046924300553],[3299.6835041708587,5939.070607816227],[3299.755498253375,5939.099973606914],[3343.219310149981,5958.832642557398],[3343.319444138216,5958.8851218181335],[3343.4130147954456,5958.948568019856],[3343.4988262055413,5959.022170263739],[3377.792909045778,5991.978974997466],[3377.8613926747275,5992.051709019906],[3377.9222774178966,5992.130913145454],[3377.974955630603,5992.215796897494],[3378.0189015713545,5992.305513115348],[3378.053676648875,5992.399166409139],[3378.0789337993556,5992.495822096007],[3378.0944209502354,5992.594515528474],[3378.099983535946,5992.694261721858],[3378.0955660405184,5992.7940651846675],[3378.0812125516395,5992.892929853844],[3376.730364819172,5999.763140575707],[3377.508918018642,6036.328299109475],[3398.962951325493,6084.989974148514],[3398.9959555107857,6085.075182528314],[3399.021043957968,6085.163047846006],[3399.038007184922,6085.252836448658],[3399.046703553043,6085.343798624404],[3399.047060449879,6085.435174862342],[3398.722655977346,6093.191786432589],[3398.713998205001,6093.287737321102],[3398.6961473668093,6093.3824097965335],[3398.669269145861,6093.4749251534595],[3398.633613013172,6093.564424707816],[3398.589509912208,6093.650077766803],[3398.5373691872255,6093.731089338981],[3398.4776747839283,6093.806707513009],[3398.410980757714,6093.876230436536],[3398.3379061311884,6093.939012830459],[3398.2591291486933,6093.994471978103],[3398.175380981156,6094.042093133724],[3398.08743893971,6094.081434300136],[3397.996119261052,6094.112130331122],[3397.9022695315225,6094.133896320548],[3391.935567516644,6095.21784084847],[3345.0414047136755,6109.02198155157],[3307.160076196108,6141.429869027697],[3307.0939840106594,6141.481765160188],[3307.023768088959,6141.52792910591],[3299.0739533672313,6146.2886501130715],[3258.9582356577325,6193.942311738048],[3237.894312068914,6245.904235612607],[3287.1294757065707,6392.7130145902265],[3363.1634093052694,6404.457667192017],[3444.7154285931388,6403.230064572846],[3475.7041633375748,6382.530901910457],[3575.0778994733437,6246.051642417012],[3576.9420902591773,6202.474906654409],[3576.9502408578446,6202.383309382064],[3576.966771296148,6202.292848135284],[3576.991541784559,6202.204287898927],[3577.0243428516274,6202.1183775819745],[3611.496499714373,6122.96073052315],[3611.5387362636143,6122.875149543014],[3611.588938669426,6122.793984595509],[3611.646649686777,6122.717974933431],[3611.711343681975,6122.6478128550825],[3709.2923163732535,6026.464059065642],[3715.1498631923264,5958.646640386757],[3715.172994384492,5958.5025604711755],[3718.86780439376,5942.878301898422],[3718.8937633897717,5942.787791757224],[3718.9281202226143,5942.700124413414],[3718.9705702861497,5942.616077121731],[3719.0207372200184,5942.536395041689],[3719.078176246436,5942.461784631036],[3719.142378113572,5942.392907382326],[3719.2127736105517,5942.330373958154],[3719.288738614058,5942.274738777056],[3719.3695996217775,5942.226495098048],[3719.454639723644,5942.186070647426],[3719.54310495793,5942.153823826555],[3783.0955837254596,5922.324078616206],[3783.11951946157,5922.316936944577],[3815.6462022010046,5913.052786044937],[3895.2965052486047,5753.054293525351],[3868.382964904006,5726.852783059383],[3701.429782077851,5735.308632991349],[3636.2086039239566,5745.951649030717],[3599.3794049698304,5770.785055368201],[3599.2910254417684,5770.838232751718],[3599.1976383863303,5770.882023538133],[3599.100237327396,5770.915961847117]],[[1661.8565296174422,7441.03395622376],[1661.8963922613336,7441.13011216755],[1670.5162153847157,7465.426294221044],[1670.5232577555853,7465.446850167461],[1672.0364437855214,7470.0253248645395],[1672.0464430293202,7470.057375857588],[1674.909782365569,7479.808186251208],[1674.9326747100213,7479.903040343978],[1674.946213473388,7479.999673983237],[1674.9502697482515,7480.097167085475],[1674.9448049133655,7480.19459138393],[1668.2416529579748,7543.895594073964],[1679.7184925182728,7574.135581055958],[1679.754540937339,7574.251247392306],[1679.7763371379644,7574.370424216898],[1683.890566367297,7608.411734847017],[1684.9305132501236,7614.97703885647],[1691.71385850717,7639.419880738001],[1691.7411455529023,7639.552466963778],[1697.8320606775717,7684.316143150923],[1712.8079693989935,7713.363021006724],[1712.8455946360225,7713.4448481626705],[1712.8757051552177,7713.529728658344],[1712.898056719862,7713.616973999021],[1712.912468028773,7713.705876507913],[1715.4562140756884,7735.601453561944],[1716.7695280823116,7741.645714937723],[1723.0208010537278,7767.042058530421],[1777.3450231652257,7826.951282809185],[1808.7841919063785,7843.295301775546],[1808.8598447415013,7843.338928619309],[1808.9314027583027,7843.388989614007],[1895.453910282364,7909.729046367582],[1918.501198477545,7912.708353680257],[1927.6474664055615,7912.391091587119],[2044.6984234299027,7803.99951367442],[2051.931622649348,7775.061646384043],[2051.959451778323,7774.9694381619975],[2051.996022668406,7774.880334926193],[2052.040996058156,7774.79516327101],[2052.093954737683,7774.714713318275],[2052.1544074190274,7774.639731387448],[2052.2217932937497,7774.570913072152],[2052.295487235446,7774.508896787278],[2052.3748055989336,7774.454257846531],[2109.690769614202,7738.9155704106815],[2166.181707783554,7695.1605833179765],[2201.058551693686,7601.349658429975],[2195.743790829945,7561.913336009569],[2195.7351634245706,7561.805539938713],[2195.738226562085,7561.697442564998],[2195.752944420856,7561.5903080250555],[2195.779144883988,7561.485389195729],[2202.484313423314,7539.717921607139],[2202.5119378512663,7539.639882333226],[2202.545922509347,7539.564395378671],[2213.490922509347,7517.717087686362],[2213.5355971932963,7517.637254917631],[2213.5873806128734,7517.561838899397],[2213.645839387546,7517.491470795735],[2233.9200393875453,7495.241670795735],[2233.9933561988755,7495.169108830969],[2234.0737579834645,7495.104485719087],[2264.3825627037745,7473.2175544388565],[2336.964845796716,7375.5633962603615],[2334.9989346467974,7354.317670379658],[2334.994902227062,7354.246572423197],[2334.046503384631,7309.181649276047],[2330.279030198563,7297.335065269452],[2330.2553174381896,7297.246688549892],[2330.239782186544,7297.156514299017],[2330.2325545162416,7297.065297522343],[2330.233694942684,7296.973801954187],[2331.52952625349,7274.745650940442],[2327.757311251294,7239.882901746504],[2301.8163953301246,7177.51004130016],[2282.442675272933,7147.978260188177],[2269.364942209883,7136.114326182458],[2269.2971016161305,7136.046576481797],[2269.2360609892526,7135.972641307688],[2269.182381437161,7135.893200299464],[2269.136556402058,7135.808983708118],[2269.0990071245337,7135.720765683555],[2269.07007877137,7135.629357158332],[2265.550415709565,7122.320631205883],[2257.436609425737,7102.733605575224],[2257.401224053072,7102.6334399993975],[2257.376664129248,7102.530085863916],[2252.8925895817138,7077.910648334481],[2252.879451884366,7077.809470246534],[2252.876692069465,7077.707480109786],[2254.2308758103773,7021.253666494454],[2254.238787546388,7021.149852718242],[2254.257450298419,7021.047424219353],[2254.2866617641853,7020.947491312032],[2254.3261052944454,7020.85113725857],[2254.375353325445,7020.759406526856],[2254.4338720136643,7020.673293468456],[2264.341810964218,7007.611708643592],[2283.2566631632067,6982.595822608067],[2328.00796578897,6921.887285470396],[2328.073399251565,6921.807492970414],[2328.1467072342884,6921.734868451487],[2328.227109125804,6921.670185247166],[2328.3137487761305,6921.6141321288615],[2361.4231312646098,6902.5414869675715],[2361.5070270346146,6902.49829662584],[2361.5946202288037,6902.463206197299],[2361.685130925624,6902.436528123817],[2361.7777532263526,6902.4184999444515],[2361.8716624307453,6902.4092821804115],[2361.966022380088,6902.408956905802],[2391.368981795593,6903.696176062835],[2407.245334499756,6903.7485458862475],[2413.8670831516984,6903.645991469587],[2413.9848933585054,6903.651120492164],[2414.101280685976,6903.67008207714],[2441.55810581803,6909.824210517508],[2441.599317879226,6909.834370906869],[2472.0986904596557,6918.044062536825],[2472.200385768463,6918.077365764707],[2472.2979401604925,6918.1213450941295],[2478.7940573615992,6921.47909532464],[2478.8733403114015,6921.524788610204],[2478.9481144074575,6921.5775379896695],[2479.0177535167254,6921.636901757536],[2496.147642523068,6937.649005351615],[2496.157770078374,6937.658605324323],[2507.908216081885,6948.953618062994],[2621.185840473854,6969.520430685288],[2691.4872894412492,6921.736121804449],[2694.8595785361836,6916.043008099199],[2698.8193868543294,6809.924390728458],[2629.7864651575323,6652.243252780736],[2550.4004364926845,6578.893602026264],[2516.449870812261,6569.320492615713],[2455.762341302073,6575.153507898322],[2455.6640450338455,6575.15809180161],[2455.5657741513305,6575.152992569657],[2455.4684802280435,6575.138259579186],[2455.37310537744,6575.114035492225],[2455.2805731302697,6575.080554874692],[2455.1917794918754,6575.038141925049],[2455.107584266021,6574.9872073350325],[2455.028802729267,6574.928244312862],[2454.9561977365097,6574.861823807418],[2454.890472334129,6574.788588979647],[2454.832262952272,6574.709248974727],[2454.7821332421922,6574.624572055298],[2454.7405686183206,6574.535378162238],[2454.7079715579143,6574.442530975045],[2454.6846577038023,6574.346929548679],[2454.670852807962,6574.249499607862],[2454.6666905455277,6574.151184583129],[2454.6722112203865,6574.052936475433],[2454.6873613749144,6573.9557066377565],[2454.711994307612,6573.8604365630035],[2454.74587149364,6573.7680487673615],[2454.788664894498,6573.679437857425],[2454.8399601344745,6573.595461867567],[2454.8992605131157,6573.51693395145],[2454.9659918148623,6573.444614508117],[2455.039507869275,6573.379203818926],[2455.119096808009,6573.3213352666035],[2455.2039879579593,6573.271569202093],[2455.293359303815,6573.2303875185835],[2455.386345447774,6573.198188985256],[2455.4820459893344,6573.175285385935],[2520.2132631085146,6561.015536353287],[2524.7229390506463,6558.3725450820675],[2524.7317073025974,6558.3674657703805],[2546.978607572139,6545.630284860278],[2547.0564681912983,6545.590128592435],[2547.137544579352,6545.556940926607],[2547.2212144910864,6545.530976571555],[2547.306835776507,6545.5124347985575],[2564.5496161794003,6542.562433928319],[2584.2348652129267,6538.136223134274],[2584.368076229745,6538.115583185429],[2608.920235227634,6535.992246962986],[2643.17059864101,6530.230027656464],[2701.645704407153,6495.373039504958],[2708.7302687804117,6489.847333053915],[2708.809221553749,6489.7916701030545],[2708.893176801493,6489.743884949584],[2708.981351061289,6489.704423519992],[2709.0729214993635,6489.673654064571],[2709.1670335891317,6489.651863720941],[2709.2628090855583,6489.639255834509],[2764.2306167209495,6485.090347412727],[2878.1793086317603,6360.213607100323],[2898.0044939604286,6328.959548520874],[2879.77438766082,6200.957651118259],[2851.1065850462996,6170.904708886712],[2818.266395512569,6151.7389664940565],[2727.9390809890806,6144.871077783484],[2717.5809415000167,6147.442539640391],[2717.4831254288424,6147.461704557744],[2717.3838873667673,6147.47103648534],[2717.2842132715095,6147.470442707883],[2717.185093432899,6147.4599291247205],[2717.0875126340716,6147.439600191219],[2716.992440367375,6147.409657880984],[2716.9008212021936,6147.370399679185],[2716.813565400388,6147.322215626964],[2716.731539872593,6147.265584446267],[2716.6555595652167,6147.20106878361],[2716.5863793637227,6147.129309620026],[2716.524686592634,6147.051019902741],[2716.471094186772,6146.96697746185],[2716.4261346015783,6146.878017282352],[2716.3902545230235,6146.785023208347],[2716.3638104296574,6146.688919161798],[2716.347065050894,6146.590659963108],[2716.3401847567243,6146.491221844717],[2716.343237904782,6146.391592751957],[2717.3658386798784,6133.715003066846],[2714.7290834020137,6081.762178236408],[2635.1272750531753,6001.642352384648],[2526.3861053306264,6042.618184520502],[2516.9502809771957,6059.134528132904],[2492.0817449882393,6103.853543308511],[2492.0376701826935,6103.9254773992],[2491.9876890510463,6103.993440538962],[2491.9321573141046,6104.056949027209],[2471.601592829085,6125.418129430964],[2471.538794173962,6125.478599416479],[2451.5807153873598,6143.0860567579575],[2451.4921202239366,6143.155745653708],[2451.396245090636,6143.215021314713],[2451.294308142884,6143.263130605457],[2406.208354910574,6161.510307703792],[2374.138887078139,6176.7850792787085],[2374.04901016747,6176.8226331624455],[2373.9559059639464,6176.851264623136],[2373.860457854519,6176.8707020010925],[2373.763571465479,6176.880760871495],[2373.6661660697328,6176.881345794239],[2373.5691658646037,6176.8724512194885],[2359.540900925979,6174.893221015507],[2265.2325483813183,6200.599583031318],[2265.139040834524,6200.6203169892215],[2265.0439785932726,6200.632010090011],[2264.9482337165778,6200.634555066345],[2264.85268452565,6200.627928571734],[2264.758207546564,6200.612191394713],[2264.665669469397,6200.587487901193],[2221.586325663427,6186.845956798004],[2190.038336711898,6183.847694407443],[2063.4067711106395,6278.206306214066],[2049.7190374387087,6315.47694272519],[2049.685942886944,6315.556327863603],[2049.646149357102,6315.632575647439],[2049.5999512118537,6315.705122052814],[2007.8205664913069,6375.4743298675085],[2005.585078343283,6379.904357601822],[2005.5353120443378,6379.991752895259],[2005.477019075175,6380.07370746543],[2005.410789047132,6380.149392372849],[2000.760175815727,6384.9533562274055],[1982.050106256059,6406.03049030913],[1977.0492811777506,6413.548297381543],[1976.9894476698473,6413.629117211407],[1976.921799882689,6413.703519297802],[1976.847021863499,6413.770751294747],[1932.2348824276291,6449.99546568994],[1873.6841962850956,6503.292845149291],[1863.5137542440116,6540.541042625049],[1863.4795185596722,6540.644058033086],[1863.434318211199,6540.7427554741535],[1831.4293061940239,6601.657559970946],[1831.6141948975726,6643.900618419807],[1837.0470540101314,6662.688438163744],[1852.5103249787874,6692.792806435003],[1852.5516470866444,6692.884278472911],[1852.5835913287813,6692.97943211557],[1862.041010862514,6726.668466520364],[1862.0549375623127,6726.724176146829],[1864.0430245694988,6735.773851812008],[1864.0585905196176,6735.864361148078],[1864.0657873877876,6735.955916834999],[1864.7347657159607,6756.526319311779],[1864.732645487835,6756.631557595595],[1864.7194750136987,6756.735990015652],[1864.695400217028,6756.838459503369],[1857.5955693088167,6781.215119140808],[1850.9681671822564,6818.085324332863],[1853.2631058407294,6852.623495675538],[1853.264975606312,6852.715504341752],[1853.2583790803837,6852.807295280998],[1853.243372129521,6852.898091107486],[1853.2200818489514,6852.987122863129],[1853.1887054861734,6853.073636529914],[1853.1495087704484,6853.156899415753],[1853.1028236623163,6853.236206359716],[1828.9940906527058,6890.189172905705],[1798.9103914739942,6939.243616704153],[1788.466698059774,6962.5409021762225],[1788.4615325064865,6962.55223562404],[1772.9638311119706,6996.001308079155],[1772.9149242952592,6996.093837145918],[1772.8566145983868,6996.180747928721],[1749.534913847452,7027.2899642422],[1728.5595703834817,7059.219661524894],[1721.392209904903,7071.119343176972],[1721.3738141698097,7071.148720592403],[1703.8260997373493,7098.121088337652],[1703.76480356407,7098.20535100976],[1703.6950719931435,7098.282777922301],[1703.6176621294362,7098.352528418861],[1703.5334144442556,7098.413845189089],[1646.1284051663376,7135.780438988551],[1620.5940659837647,7206.25289114865],[1619.3271455348588,7212.303341294321],[1602.9054747589319,7390.335460547707],[1661.605156764572,7440.705575586725],[1661.6805164572868,7440.777380270542],[1661.7480037768998,7440.856629608682],[1661.8068874985795,7440.94246493489],[1661.8565296174422,7441.03395622376]],[[4792.428482011328,6938.576975110031],[4792.414716516858,6938.566911255049],[4768.6447826891335,6920.875935317817],[4763.6767764003835,6918.390904932269],[4763.615687269158,6918.35764284849],[4708.942023687699,6886.074345937153],[4663.712894716252,6876.564116072883],[4663.606663691904,6876.535597664815],[4636.798019704796,6867.7318585425655],[4619.354437598359,6865.484956547419],[4619.259564105638,6865.468054227977],[4619.166758082229,6865.442098302976],[4619.076881386862,6865.407329816475],[4618.990768674571,6865.364071651864],[4618.909219645526,6865.312725533359],[4613.289192720929,6861.383714249542],[4470.978181756903,6897.699030512603],[4456.614568502385,6902.0546851971685],[4434.447828889961,6910.151637188825],[4402.3122097800315,6933.779107875462],[4370.095302792921,7032.504720047203],[4373.4889825991095,7047.243198014497],[4373.498027705056,7047.286922601428],[4377.830260274902,7070.871891139057],[4377.843316703846,7070.970179392679],[4377.846575493705,7071.0692774825975],[4377.84000460715,7071.168211170423],[4377.823668642903,7071.266007834011],[4377.797728200663,7071.361706029362],[4377.762438302245,7071.454364942622],[4377.718145884444,7071.543073639277],[4297.671160460232,7213.750417247907],[4304.027119988644,7235.22360962304],[4304.051154727595,7235.323353607111],[4304.0648428183,7235.42503530265],[4304.06804017265,7235.527584354133],[4303.798523044056,7248.8991328134225],[4303.79364739373,7248.979636981617],[4303.782300085861,7249.059486412841],[4296.408316239253,7289.240299335554],[4296.385877218098,7289.335872802729],[4296.3541749731185,7289.428785476577],[4296.313515044126,7289.51814188358],[4296.264289303272,7289.603080824801],[4296.206972178271,7289.682783675941],[4296.142116079954,7289.756482277077],[4296.070346078251,7289.82346633603],[4295.992353877889,7289.883090274017],[4295.908891151888,7289.934779447612],[4295.820762297083,7289.978035687033],[4262.66618112358,7304.276967799573],[4238.349117747421,7324.782785245244],[4204.593892373113,7372.506324992053],[4200.087017063516,7394.037052031701],[4352.371968803553,7498.151958733641],[4409.911551435644,7461.215500956643],[4440.223262017606,7429.689267692821],[4440.297455955979,7429.619576003081],[4440.37835039076,7429.557787957867],[4440.465107120342,7429.504543784643],[4440.556827199937,7429.460395182074],[4440.652560256159,7429.425799603504],[4470.413497782322,7420.354667912082],[4583.829904977215,7345.705480639432],[4589.738760267947,7335.691801098058],[4589.78356038325,7335.622569179726],[4589.833912376813,7335.557263853824],[4607.18076951967,7314.881263853825],[4607.257371021846,7314.799701105253],[4607.342605077858,7314.727206775918],[4607.435404534135,7314.664688514547],[4631.786560636311,7300.1711520907065],[4631.8967152434525,7300.11451602639],[4637.497861662285,7297.660523038467],[4637.596448965252,7297.623388098131],[4637.69839589083,7297.596830905783],[4637.802570985133,7297.581146205363],[4637.907818065032,7297.576508072893],[4638.0129690500125,7297.582967984503],[4638.116856926061,7297.600454245118],[4689.971720250701,7309.166512392514],[4690.048565490702,7309.186890355071],[4706.467053157266,7314.247321725309],[4823.240346462728,7268.705983157372],[4823.276181901265,7268.6927900176],[4839.838257321958,7262.951959340524],[4895.984762124002,7103.375151227682],[4893.191114089745,7097.304887994705],[4879.389153516136,7071.48073624564],[4879.367358294593,7071.4374663765075],[4867.069090631549,7045.474830506942],[4867.048995605557,7045.429540961202],[4835.626877202055,6969.597325861587],[4792.428482011328,6938.576975110031]],[[5498.768899791149,3532.371962048931],[5498.693291210566,3532.451999879652],[5478.591247113348,3551.510940873546],[5458.003556910971,3577.7324385108204],[5457.944558030096,3577.800962325174],[5457.879610531476,3577.8638765803244],[5457.8092454506095,3577.920666865345],[5457.7340381192125,3577.970868841225],[5446.813313138912,3584.567086335315],[5446.730498606014,3584.611926494103],[5446.643833182026,3584.648777423323],[5446.554085492776,3584.6773122962577],[5440.311840384581,3586.3427867082237],[5369.405143919494,3639.846136760702],[5369.324879188223,3639.9007946862553],[5369.239691453488,3639.947409767102],[5369.150384028463,3639.9855424269485],[5369.057799074656,3640.01483307802],[5368.9628096603965,3640.035005511939],[5368.866311527873,3640.045869504351],[5368.769214646361,3640.0473226087197],[5368.672434631289,3640.0393511223942],[5368.576884110061,3640.02203021582],[5368.483464116056,3639.9955232236894],[5357.391970104358,3636.2576957444753],[5267.102709089196,3642.772304490309],[5207.6899418516905,3674.138174790966],[5207.606341621212,3674.1774847039096],[5207.5194705071635,3674.208912038124],[5207.430069890897,3674.2321885843235],[5207.338902741213,3674.247115694229],[5207.2467471029795,3674.2535659758873],[5207.154389457091,3674.251484380868],[5135.878064907615,3669.344104144757],[5051.57142235744,3780.788813879975],[5051.503676011685,3780.8692074805685],[5051.427863815953,3780.9420443486915],[5051.344823699578,3781.0065194404096],[5051.25547347992,3781.061920131981],[5051.160800718006,3781.1076340962722],[5051.061851803319,3781.1431560706233],[4995.123753104748,3797.9622095266973],[4966.294233544458,3816.02362648947],[4943.242846434115,3834.089647439722],[4940.717179973054,3996.6293315151374],[4965.395919420434,4020.855612533117],[4965.456905322737,4020.92109018859],[4965.511794030568,4020.9917572487984],[4965.560146071798,4021.067047910601],[4965.601574310799,4021.146359351511],[5041.22756392972,4183.208607180342],[5137.944002042776,4134.991089602068],[5146.9282538253265,4117.346259634589],[5146.975120666988,4117.264077352663],[5155.819002213338,4103.331846352812],[5165.955318488168,4080.2918689997027],[5165.992917737287,4080.2154177883453],[5166.036888014136,4080.1424444644103],[5166.086910160846,4080.0734787041315],[5175.704347144118,4067.9373442672945],[5186.115369653169,4048.1860147055863],[5186.164798193506,4048.102364111175],[5186.222111542143,4048.023905315536],[5186.2867686265345,4047.951379016974],[5186.358159044847,4047.8854699074486],[5186.435608828513,4047.8268002086666],[5186.518386804909,4047.775923797936],[5186.60571150006,4047.7333209792287],[5186.696758516228,4047.699393948824],[5186.790668314719,4047.6744629983314],[5186.886554330452,4047.658763490945],[5213.103476100516,4044.665241673184],[5322.292812792661,3969.406214043225],[5326.413297886763,3951.285515541801],[5326.424520804438,3951.240927543612],[5341.381115007335,3897.1086811668006],[5341.411554882071,3897.0162797582866],[5341.450829447321,3896.927273495621],[5341.49856698479,3896.842504788036],[5341.554315677482,3896.7627759379725],[5341.6175478859695,3896.6888415476046],[5341.687665142296,3896.6214013768267],[5341.764003814238,3896.561093720303],[5341.845841386322,3896.508489366256],[5341.93240329815,3896.4640861941857],[5342.022870275309,3896.4283044626327],[5342.116386083481,3896.4014828315976],[5342.21206563236,3896.383875157258],[5418.310359831447,3886.1772107880615],[5432.5205104408005,3880.043913172271],[5432.611004397806,3880.0099428596827],[5469.910110116374,3868.0306805210157],[5469.919408521581,3868.0277441081944],[5477.39795892629,3865.706073762352],[5477.4327717274455,3865.6959544454976],[5505.13556866231,3858.1851881619727],[5505.162005221296,3858.1784065836823],[5510.973497041892,3856.7718635067126],[5609.696816008474,3776.9702365705243],[5613.925229153395,3770.6259683228686],[5613.981138667496,3770.5500909482794],[5614.043943424932,3770.479814265196],[5614.113085515356,3770.415762558946],[5614.18795073231,3770.358504816832],[5614.267874029378,3770.3085496736767],[5614.352145427948,3770.2663408934927],[5631.498048553856,3762.666999609937],[5631.539240237885,3762.649831951467],[5687.5301087874695,3740.7674280440556],[5687.62153909313,3740.7366899981703],[5687.715505559132,3740.714903958488],[5687.811133890631,3740.7022726294726],[5695.898955885525,3740.028791876519],[5737.061128766741,3734.0303010347884],[5767.773765940836,3720.378295338367],[5822.165354102387,3694.1279699941465],[5822.288864110898,3694.078205994376],[5841.562938184972,3687.768153084323],[5841.612326249004,3687.7533822192986],[5868.42371844492,3680.4820531851924],[5924.883121346254,3637.540342206307],[5924.9184465112085,3637.514672533148],[5929.543587299624,3634.30566207458],[5929.630350689696,3634.251810016837],[5929.722153748936,3634.2070872229388],[5929.818039161471,3634.171960059048],[5929.917007040931,3634.1467948287705],[5930.018025357202,3634.131853953365],[5930.120040698352,3634.127293235235],[5930.221989255549,3634.133160233229],[5930.322807916375,3634.1493937667033],[5930.421445350896,3634.175824553507],[5930.51687297485,3634.212176975246],[5985.744167136957,3658.5480641160875],[6053.980826908698,3644.056102595201],[6054.0781308136075,3644.040402989531],[6054.176507596911,3644.0343584849506],[6105.551791590025,3643.4145306019905],[6105.627681522175,3643.416496795565],[6105.70320361508,3643.424214386006],[6118.838395812442,3645.272613697538],[6118.928852760627,3645.2896204825815],[6119.017362870843,3645.314872227115],[6175.592221174919,3664.311630474221],[6193.378243204846,3663.280294411669],[6238.835460890722,3652.9407775751715],[6268.125169463376,3643.9645525021474],[6268.239959013892,3643.9366709985215],[6274.764150077065,3642.754992892344],[6274.864507502377,3642.742019168474],[6274.965662263745,3642.7392542852976],[6275.066578543459,3642.7467265550254],[6283.680771617936,3643.825007784547],[6395.201211555125,3564.4238941693307],[6354.614214959766,3439.2612231212897],[6329.475484233189,3407.0257261649926],[6255.110201438074,3374.546258341626],[6255.021301865526,3374.5020527256006],[6254.937223936379,3374.449249578746],[6254.858796429524,3374.388369395962],[6254.786792426108,3374.3200122896783],[6254.72192168907,3374.2448520743815],[6254.664823666811,3374.163629624643],[6254.616061189981,3374.07714557212],[6254.576114923504,3373.9862524135115],[6254.5453786285325,3373.8918461072662],[6254.524155281041,3373.794857241882],[6254.512654085307,3373.6962418628373],[6254.5109884117255,3373.596972048599],[6254.519174679293,3373.498026328577],[6254.537132193756,3373.400380037497],[6254.564683943037,3373.3049957012627],[6254.601558342091,3373.2128135490757],[6254.647391909992,3373.124742245345],[6254.701732852864,3373.041649932733],[6254.764045517334,3372.964355674641],[6254.833715670614,3372.893621381478],[6254.910056555159,3372.8301443003047],[6254.99231565822,3372.77455014188],[6260.663957449264,3369.3387292463576],[6260.751782889397,3369.291347084812],[6307.952241380638,3346.7910041687396],[6347.405170431173,3305.009816035769],[6407.984253484722,3237.634671042095],[6408.051889680148,3237.5663579610377],[6408.125772823513,3237.5048550090623],[6408.205220136163,3237.450730555366],[6408.289497419106,3237.404484781993],[6408.377825837993,3237.366545061487],[6408.4693891205825,3237.3372620074015],[6408.563341100184,3237.3169062341594],[6408.658813535362,3237.305665856219],[6408.754924133651,3237.303644749645],[6413.988500777798,3237.4452924891166],[6534.834695103643,3084.947635183161],[6526.195367325861,3070.175785932248],[6503.462108252023,3038.9389510817373],[6484.103261255149,3021.6233181884263],[6428.4020931091,2983.7424539626377],[6428.32391052593,2983.6834854346666],[6428.251870537239,2983.6171526099743],[6428.18666399237,2983.544091607322],[6428.128916209283,2983.4650030673765],[6428.079180977874,2983.3806454337027],[6417.1857042753645,2962.6457350394376],[6417.156072799927,2962.584337178906],[6415.2584383913245,2958.283692017616],[6415.224808590094,2958.1967030335554],[6403.4094370402,2922.8096966641283],[6403.387155403365,2922.7328581181614],[6403.371087542354,2922.6544842875232],[6402.063125758915,2914.6614906569503],[6402.052690659952,2914.5733081185967],[6402.050119396357,2914.4845475419634],[6402.35972304056,2894.4510565644523],[6395.0036887187935,2837.3186442583747],[6245.9580074198,2785.4913638261874],[6166.052111744282,2832.260586419294],[6144.524506169707,2855.4867069638985],[6126.404929567244,2878.1914789548273],[6126.349896531453,2878.2547968407375],[6126.289749991469,2878.3132791750995],[6126.22491324599,2878.3665143715416],[6105.271928938856,2894.1466672203137],[6011.5498785544905,2971.975141720531],[5989.0846223442495,3014.659859051265],[5985.817659438829,3069.6825713943986],[5985.8067693714665,3069.7818450782434],[5985.786031596627,3069.8795374656947],[5985.75565294993,3069.9746741867657],[5985.715936423679,3070.0663063613215],[5985.6672781448615,3070.1535200630765],[5918.232476053748,3177.986899254146],[5918.178155573703,3178.06519800745],[5918.11662863039,3178.1379705611193],[5918.0484539751615,3178.2045560378074],[5848.022281968423,3240.3620300606794],[5773.219409236199,3335.6226563138553],[5773.137999158754,3335.7141833386245],[5773.046009382527,3335.795070161051],[5748.782563638977,3354.6251213737287],[5748.714302880665,3354.673656327958],[5748.642219994966,3354.7163087663007],[5704.266667746449,3378.523471476814],[5664.960002664319,3431.6706256938332],[5664.899809565012,3431.7443916000343],[5664.832874116042,3431.8120987916254],[5664.759803061694,3431.87313352877],[5664.68125876317,3431.926942554884],[5649.570345468372,3441.2544107629765],[5649.551655337205,3441.265667849167],[5596.5549332011615,3472.4028613183173],[5575.79452313939,3492.309740489574],[5575.717230309155,3492.3766187477067],[5575.633514519297,3492.4352578945736],[5575.544250338881,3492.4850453338663],[5501.772511407679,3528.8194472951423],[5498.768899791149,3532.371962048931]],[[6339.102267991995,5405.019013002583],[6339.130768469711,5404.929332964538],[6339.167572299229,5404.842728896581],[6339.212353590498,5404.759967659241],[6339.264715814747,5404.6817820856],[6339.324195315663,5404.608864492218],[6339.390265414966,5404.541860548812],[6339.462341076036,5404.4813635609935],[6339.539784084285,5404.427909216669],[6389.759459510575,5373.146688246697],[6392.930144515866,5369.530474134236],[6392.997988366114,5369.46032058007],[6393.072347443072,5369.397114276171],[6445.777109347834,5328.855722334779],[6445.861533324383,5328.7974221177055],[6445.951486566496,5328.748079059829],[6446.046022197281,5328.708212562124],[6446.144145104139,5328.6782422722235],[6446.24482241365,5328.6584836670845],[6446.346994363919,5328.6491447321705],[6446.4495854599845,5328.650323772127],[6527.280604901531,5333.734296829361],[6532.455686053809,5331.666914944293],[6532.540986661478,5331.637230488244],[6564.746027311887,5322.036796883909],[6564.8563644439255,5322.010614522687],[6564.968956450025,5321.99709272886],[6608.762274231563,5319.243597540333],[6672.824468648144,5300.451548372243],[6671.764799282583,5137.431573709929],[6584.428550457791,5110.748261892779],[6494.39853276997,5097.059920082748],[6369.424809700586,5097.56362824122],[6369.324423399642,5097.5589833160775],[6369.225010194666,5097.544286397244],[6369.127574058098,5097.51968590868],[6369.033098995997,5097.485430290342],[6368.9425391105715,5097.4418654892],[6368.856808964724,5097.3894314655145],[6368.776774345891,5097.32865774969],[6368.703243522466,5097.260158094558],[6368.636959081114,5097.184624277089],[6368.578590427396,5097.102819112154],[6368.528727025457,5097.015568748867],[6366.076147127947,5092.174514305148],[6299.185149979934,5038.74924519522],[6202.391509152724,5057.9286119912695],[6151.684334616201,5089.704037681979],[6151.595369818997,5089.7536637376425],[6151.501817796765,5089.793981223656],[6151.404649383793,5089.824571746093],[6151.304872943399,5089.845117852401],[6151.203523903649,5089.855406325762],[6151.101654012232,5089.855330397743],[6072.571109322909,5085.791494785281],[6012.4665620983,5112.974874756064],[6004.671285685166,5117.285339286226],[5915.540114041739,5228.059401923572],[5956.2486786609325,5309.552607488347],[6043.522695471248,5391.353882378836],[6043.609431243794,5391.446171558874],[6147.266632498261,5516.779450634932],[6240.6394627981035,5540.4362439157085],[6329.57242130495,5461.696744496018],[6339.082323231726,5405.110974913131],[6339.102267991995,5405.019013002583]],[[5320.429451210839,7847.560251775482],[5320.4656534662445,7847.648084564354],[5320.493357565785,7847.738956369476],[5347.298833456096,7953.422651934434],[5351.929084694442,7964.680256086676],[5351.964742549075,7964.782314240075],[5358.973118808873,7988.9679917316735],[5358.995831374679,7989.063783157672],[5359.009014907739,7989.161343664008],[5359.012541635121,7989.259727708113],[5359.006377376286,7989.357981765807],[5358.990581874354,7989.455153572722],[5358.965308217086,7989.55030135351],[5358.930801353178,7989.642502949394],[5358.887395718253,7989.730864755607],[5358.8355119935595,7989.814530382075],[5358.775653028784,7989.892688953431],[5358.7083989685125,7989.964582967907],[5358.634401629541,7990.029515638925],[5281.1585931335585,8051.5363422835135],[5333.845191256928,8185.898447963285],[5354.087956808075,8197.047851752528],[5493.763475103526,8168.262477447219],[5564.188175666195,8146.384414757069],[5564.287501871715,8146.359060162415],[5564.3889019041,8146.344007454834],[5564.491310195981,8146.339414816467],[5564.593650584644,8146.345330509284],[5564.694847620938,8146.361692367931],[5564.79383787069,8146.388328452986],[5564.889581089856,8146.424958857803],[5598.404732605009,8161.258898251743],[5598.461663211798,8161.2862780482055],[5608.120070291444,8166.312944714872],[5608.19486084417,8166.356070288159],[5608.265652969957,8166.405486042476],[5608.3319190238135,8166.4608236603735],[5612.796330983864,8170.528641605275],[5725.878157665645,8182.1501739783735],[5808.863990458151,8029.1057438994885],[5782.97723987985,7961.167729692478],[5773.721779171508,7948.9334421545045],[5773.667626079341,7948.854229749779],[5773.621314276553,7948.770191785879],[5773.5832701634345,7948.682102013994],[5773.553844018317,7948.590771490962],[5761.4032625620575,7903.585868400338],[5761.3838643267545,7903.498731830108],[5761.372314284574,7903.410212510579],[5761.36870447865,7903.321015858706],[5761.373063675792,7903.231852689154],[5761.385357137237,7903.143433549767],[5766.343720308593,7876.321745570227],[5766.344686941732,7876.316592294385],[5775.6179216110195,7827.58488443371],[5764.869708914704,7760.2393197187985],[5760.669783103702,7745.355176188866],[5733.725062681216,7694.237220749236],[5733.682728942016,7694.14607864559],[5733.649756625451,7694.051147855685],[5733.626478719885,7693.953387088277],[5733.613130309551,7693.853783632146],[5733.609846200424,7693.753343385437],[5733.616659558818,7693.653080697067],[5733.633501576432,7693.554008122787],[5733.660202165248,7693.4571261993515],[5733.696491675259,7693.363413340069],[5733.742003617667,7693.273815953779],[5733.796278366068,7693.189238887044],[5733.85876779823,7693.110536286071],[5733.928840831592,7693.038502970674],[5781.398809663197,7648.910991749799],[5814.104086132952,7587.14360235416],[5814.878716230726,7470.415177257761],[5745.854192170613,7365.2681718423755],[5699.229199753342,7342.903709526954],[5670.426943106818,7339.909486283074],[5650.920700596883,7341.425792305629],[5650.820503218987,7341.428542394886],[5626.454614143357,7340.875372646986],[5626.361135378393,7340.86885894808],[5626.268676716949,7340.853623998243],[5626.178050011511,7340.829801571118],[5595.168596229997,7331.104171319017],[5595.1549906781465,7331.099797123211],[5587.281886236416,7328.506369229214],[5557.79860929393,7319.006404369629],[5494.612526735011,7311.389490562509],[5404.5801809424975,7327.345581685656],[5359.108797189266,7340.323234090526],[5342.95386626893,7347.068750591004],[5276.463283993589,7415.865336052702],[5286.933058676341,7496.645903557106],[5286.9408842428065,7496.743808305272],[5286.939067755671,7496.842008506791],[5286.927626737821,7496.939556865642],[5286.906671555942,7497.035512373854],[5286.876404355855,7497.128949389018],[5286.837117112503,7497.218966563567],[5286.78918881339,7497.304695539696],[5286.733081802651,7497.385309326047],[5286.669337321008,7497.460030275344],[5286.598570284653,7497.528137586034],[5286.521463353406,7497.588974255548],[5286.438760345387,7497.641953418136],[5286.351259061713,7497.686564006109],[5286.2598035904475,7497.722375679898],[5286.165276164038,7497.749042979354],[5286.06858864879,7497.766308656262],[5285.970673748478,7497.774006155901],[5285.872476006942,7497.772061223732],[5285.774942696474,7497.760492621696],[5285.6790146798785,7497.739411947231],[5285.5856173343645,7497.709022556731],[5285.495651624825,7497.669617603859],[5198.852622263713,7454.530935703577],[5198.768214270546,7454.483683226506],[5198.68876678251,7454.4284962484735],[5198.6150232299415,7454.365891181951],[5193.372812344171,7449.459953130032],[5104.324678790937,7406.513468924965],[5052.044721162719,7418.306462487397],[5017.039641535991,7430.749704604434],[4976.6772383608595,7468.822420033578],[4986.396983470195,7603.2910059977985],[5016.321623521422,7630.644320252319],[5021.330719231965,7633.712171132354],[5062.950554109941,7654.621179516607],[5063.032928160154,7654.667563332295],[5063.110554112766,7654.721518443151],[5063.182738229101,7654.78256265545],[5063.2488354038605,7654.85015042058],[5088.61919063206,7683.373359200322],[5140.198292310164,7703.960904944957],[5140.289339068512,7704.002646550815],[5140.375753580395,7704.053286515282],[5140.45666894633,7704.112316824622],[5140.531273433572,7704.179145294218],[5140.598818619316,7704.253101509292],[5152.954900000171,7719.223419022922],[5285.106529128869,7809.500220725308],[5285.1997159021175,7809.572282163126],[5285.283782081844,7809.65480187565],[5313.582467759707,7840.912953596654],[5317.162282458139,7844.405501613356],[5320.207279195634,7847.25460554492],[5320.273487965439,7847.32273484037],[5320.332932911859,7847.39683949908],[5320.385077532238,7847.476250711532],[5320.429451210839,7847.560251775482]],[[7931.07064580305,4223.251204003917],[7930.971455277542,4223.223744292951],[7930.875612549331,4223.18623472716],[7927.030217812488,4221.448076832424],[7927.0078545889955,4221.4376341744955],[7909.6293856894745,4213.059882978323],[7909.540431807108,4213.011298038859],[7889.952612436935,4200.985540463102],[7889.86023689079,4200.921407655458],[7889.775646129776,4200.847308656187],[7872.8269759883515,4184.226335029483],[7857.683946342012,4171.663683279796],[7822.480035259629,4152.6617683590775],[7790.240248321915,4144.34630991903],[7790.202676517371,4144.335833605764],[7786.67114963329,4143.276473611267],[7637.017810647373,4250.163639681274],[7683.447204271639,4314.377228582188],[7683.491433804244,4314.443857376702],[7683.530197669317,4314.5138074344395],[7726.6527772703985,4400.245991613365],[7772.289754384025,4444.960126490767],[7786.689377910889,4453.919502091489],[7796.4365738136585,4459.659843607377],[7796.518164417352,4459.7134227577135],[7796.594142694053,4459.774701248487],[7796.663784741584,4459.843095233437],[7796.726427027794,4459.91795307164],[7796.781472712514,4459.99856153618],[7796.828397334116,4460.084152609601],[7796.866753806443,4460.173910801386],[7796.896176678541,4460.266980917747],[7796.916385616583,4460.362476209699],[7796.927188074819,4460.459486821788],[7796.928481130108,4460.557088460964],[7794.566580320812,4526.917536686737],[7794.558154620972,4527.0162614097535],[7794.540000290118,4527.113667690986],[7794.51229555954,4527.208799238099],[7792.996159947419,4531.592926378016],[7811.54329507147,4632.31985750325],[7921.986595917555,4700.135840270765],[7927.909143417502,4701.980534513414],[7928.00710507472,4702.016759418919],[7928.100754070571,4702.063004371507],[7980.807356711628,4731.6093429069215],[7980.855128698421,4731.637898541178],[8084.338502970238,4797.471287339736],[8109.193365444955,4794.805701718965],[8109.3373377242815,4794.800697295938],[8124.125776462679,4795.353249236346],[8142.552537634458,4794.764303479168],[8142.653598925816,4794.7661844850845],[8142.753954062631,4794.778257981234],[8142.852577724217,4794.800400613638],[8156.839625994934,4798.692746946609],[8214.676091308513,4795.629562213518],[8228.377004490518,4790.953599502929],[8228.476463892237,4790.9253043508215],[8228.578313518217,4790.907431412873],[8228.681464311976,4790.900171800623],[8265.56524809576,4790.216388016839],[8265.652225235195,4790.218561081549],[8265.738684290298,4790.2282861412195],[8265.823970788064,4790.245489579632],[8265.907439131459,4790.270041171142],[8280.46552726886,4795.2498840682265],[8280.563965765765,4795.2895069711885],[8280.657651438658,4795.339337752907],[8280.74552937636,4795.398815313192],[8297.853657455176,4808.352756199892],[8297.934370177902,4808.4208652664975],[8298.00744985411,4808.4971066561],[8322.632882380061,4837.066137797969],[8322.693637673769,4837.144104767379],[8322.746399058973,4837.227688798533],[8322.790651054089,4837.316073270928],[8322.825961314733,4837.408394663497],[8343.997611045752,4902.192359125384],[8367.140489363808,4933.58285548204],[8367.19534387879,4933.665557171268],[8367.241731064429,4933.753288672017],[8367.279194072482,4933.84518595295],[8369.815339982577,4941.073262592596],[8369.84249018234,4941.164259518948],[8369.860886562703,4941.257421459366],[8369.870363232943,4941.35190831916],[8369.87083473649,4941.446868056088],[8369.862296821524,4941.5414443636955],[8367.801326921859,4956.432481152993],[8367.785280712245,4956.519722245917],[8367.761566569625,4956.605198140632],[8367.730371088472,4956.6882362709885],[8367.69193973009,4956.76818325236],[8367.646574891207,4956.8444100227935],[8355.597344121976,4975.187486945872],[8355.533661492867,4975.273934609876],[8355.461077307604,4975.353056110822],[8355.380428365732,4975.423939282569],[8355.29264444276,4975.485766936009],[8341.153328203443,4984.348539965448],[8341.075314299094,4984.392698291154],[8340.993659289274,4984.429692701164],[8317.566415688827,4993.8019479922805],[8299.719273910941,5004.093144097716],[8254.685193053636,5078.295602542906],[8253.308663144166,5104.640427811458],[8255.527159110674,5131.3186976247225],[8278.620653253967,5189.7000260630475],[8432.038483499307,5215.816510053717],[8450.478048238001,5209.145020286519],[8480.898081666213,5195.129557176299],[8480.991342561758,5195.092147562937],[8481.087886953726,5195.064286224326],[8481.186740026267,5195.0462544784605],[8481.28690365261,5195.03823439321],[8481.387366473227,5195.040306947964],[8505.646431149476,5196.762928392974],[8603.866872319424,5176.346644501741],[8665.454387339105,5027.005888474451],[8646.961216683478,4994.185494909095],[8646.958450925647,4994.180553775801],[8643.467194963805,4987.901645455716],[8643.429159138987,4987.825837937748],[8630.995387368524,4960.177978942556],[8586.205339660988,4910.870151596333],[8582.527338874863,4907.440688579134],[8582.460592898306,4907.3722006537655],[8582.400694334638,4907.297650111321],[8582.348190993443,4907.217718762831],[8582.303563050396,4907.13313763014],[8582.267218655765,4907.0446802602555],[8582.239490201617,4906.953155650785],[8582.220631281894,4906.859400851149],[8568.573342227406,4816.96724860046],[8561.452046399407,4800.503853927798],[8561.421942765488,4800.425357090535],[8561.398539015045,4800.344609045971],[8561.382000565369,4800.262180519854],[8550.46418798273,4730.827986127494],[8486.698798679285,4672.636960989104],[8486.637650617278,4672.576121992869],[8486.581973016753,4672.510239720976],[8486.53218014517,4672.439804368422],[8486.48864248484,4672.365340007262],[8464.81955859726,4631.33918682125],[8464.780553820965,4631.256366900465],[8464.749286298997,4631.170327061304],[8464.726018069197,4631.081788361659],[8464.710944131244,4630.991492801151],[8461.830976450125,4607.031681327949],[8461.824016650457,4606.93167152809],[8461.827105371618,4606.831467442939],[8461.840211570729,4606.732076163523],[8461.86320352526,4606.634496611843],[8461.895850156898,4606.539709501317],[8471.330020369664,4583.045369075785],[8471.375048934642,4582.948534968094],[8471.430147040943,4582.85705483496],[8471.49468632684,4582.7719719538845],[8471.567930759462,4582.694256645444],[8471.649045028826,4582.62479520735],[8482.20595229874,4574.520102419553],[8512.042745295465,4549.777958763629],[8560.514869345896,4505.991201851284],[8570.744088037522,4490.240196449773],[8451.170847837579,4335.627386304369],[8431.211455609762,4334.29603074769],[8394.779293513266,4339.478495050456],[8394.669258562952,4339.487987197604],[8394.558847956963,4339.485287339513],[8354.81562451374,4336.311111515337],[8354.800678594127,4336.309804925929],[8336.39324537694,4334.56137287018],[8336.285768730851,4334.545231724059],[8336.180678488468,4334.517522393351],[8316.37902745657,4328.127053350198],[8316.306510320732,4328.100517467252],[8316.236256956741,4328.068465120657],[8254.454343590443,4296.9453015522095],[8234.103408941122,4288.182205617137],[8234.039701207603,4288.152069230496],[8196.034133442035,4268.506110744537],[8195.960387822497,4268.46392734049],[8195.8904889269,4268.415636550764],[8063.869810214933,4168.663737606172],[8023.315728129377,4172.8139868253165],[7976.2722480307,4185.771301728949],[7939.8711945485475,4219.6731607522925],[7939.804509006834,4219.730020396199],[7939.7331013996145,4219.780823291882],[7939.657520135925,4219.825179274091],[7939.578345678401,4219.862747689743],[7931.676190505987,4223.196368379398],[7931.579429051173,4223.2314399767265],[7931.479575251451,4223.2563802004215],[7931.377686837759,4223.270924863779],[7931.274843093286,4223.274919898147],[7931.172133420844,4223.268322984942],[7931.07064580305,4223.251204003917]],[[11513.982418452253,6313.689660002591],[11514.081937011715,6313.694935228287],[11514.180436306551,6313.710089785356],[11514.276938064257,6313.734973162199],[11514.370483851406,6313.769338222797],[11568.08703629612,6336.601137843347],[11623.58918819831,6356.3087747712825],[11678.465743340195,6347.359706649158],[11702.80310822062,6336.589439505403],[11702.82217044305,6336.581239150007],[11725.919641393151,6326.927718985961],[11726.014334890397,6326.893631071469],[11726.111975154778,6326.869233876817],[11756.64754014622,6320.853633686382],[11833.112084646513,6261.6675027065685],[11849.637170793816,6244.376711781186],[11871.562785888771,6207.243794213677],[11871.613766313834,6207.165966814329],[11871.671759175484,6207.093214248558],[11871.736262481261,6207.026166270417],[11871.806717883592,6206.965403254436],[11871.882515512918,6206.911451171833],[11871.962999256788,6206.864777037651],[11872.047472439233,6206.82578486823],[11876.426089259261,6205.046800480857],[11914.533685071689,6034.221523074178],[11911.940344585413,6024.209526758684],[11911.917812128762,6024.0956790231],[11902.61849292515,5956.8076023557805],[11887.263031336368,5933.046143466195],[11852.320730004416,5899.640468642183],[11852.306020643613,5899.626113937777],[11836.24451022068,5883.626331945449],[11801.525372459482,5855.1337482134795],[11623.200151020374,5924.659946423566],[11584.658778195582,5940.832352112703],[11505.96158481972,6063.730925035471],[11505.90944756519,6063.8047694877405],[11505.85085496097,6063.873604095111],[11505.7862857846,6063.936866389598],[11505.71626764996,6063.994039436242],[11505.641372695984,6064.044656057129],[11505.562212911544,6064.088302648846],[11429.619039653542,6101.589856321163],[11429.569232578044,6101.612775309993],[11399.761926908843,6114.350589453768],[11399.736254296065,6114.361141440559],[11395.826379687916,6115.9051069578],[11395.698204696577,6115.945991553327],[11329.811841060213,6132.170991553327],[11329.796100809452,6132.17473291885],[11308.71254787114,6137.006320829224],[11275.138890295586,6313.118813059701],[11410.316074601737,6361.308910060942],[11459.74858470738,6331.623195470546],[11459.842392882518,6331.573437263478],[11459.940948436993,6331.533906824881],[11499.426438912336,6318.082616100293],[11499.457217620251,6318.072683406253],[11513.68762285559,6313.733141498376],[11513.784277428487,6313.708858475058],[11513.882869023779,6313.694316500607],[11513.982418452253,6313.689660002591]],[[8198.818654767392,7533.729760252859],[8168.289315208507,7503.712011950217],[8160.203496596152,7498.869742471655],[8160.124980593007,7498.817541476559],[8160.051729893182,7498.758177837742],[8159.984395673906,7498.692179280536],[8159.923576516637,7498.620132512755],[8159.86981308584,7498.542678009027],[8159.8235833226445,7498.46050431717],[8142.060156749219,7463.079455366121],[8142.055637481795,7463.070338544362],[8090.807873016826,7358.3515034432585],[7941.336931916539,7320.873712361693],[7918.47542238051,7340.526938440812],[7918.396090096154,7340.588489794725],[7918.310985151624,7340.641775148496],[7918.220965590334,7340.686257269569],[7918.126939005751,7340.7214876811695],[7918.029853390873,7340.747111183929],[7917.930687580394,7340.762869437066],[7917.830441381916,7340.768603563029],[7917.7301254957065,7340.764255749325],[7917.63075132464,7340.749869831396],[7917.533320777045,7340.725590850663],[7917.438816165292,7340.691663592194],[7917.348190301935,7340.648430116733],[7917.262356893285,7340.596326311981],[7917.1821813272645,7340.535877497906],[7878.687796693179,7308.358232220459],[7862.584313448275,7295.660913235499],[7862.503180391417,7295.5895029244475],[7862.430228218079,7295.509753405046],[7862.366309155947,7295.422596311612],[7849.473077031066,7275.687828437698],[7819.325856543067,7232.811619126689],[7730.463718371651,7212.030871805524],[7730.373472190381,7212.00524819926],[7730.286024324917,7211.97128037246],[7730.202144398887,7211.929267274012],[7730.122570634693,7211.879578658779],[7730.048003356453,7211.822651833401],[7729.979098826488,7211.758987807592],[7704.548428076971,7185.9484107035605],[7639.110797381153,7160.955735147346],[7465.7413210724535,7187.627192522669],[7445.613490523548,7201.087570119567],[7430.3607077411225,7358.242755478487],[7430.349638855805,7358.322912410903],[7410.067502971387,7471.261022524139],[7410.065060528412,7471.27412073276],[7402.301992287586,7511.419498998347],[7405.256384259877,7565.616351816188],[7528.93559825573,7676.356144305437],[7566.72491983853,7689.488887868748],[7566.807067031755,7689.521573635439],[7587.05747543851,7698.635582868372],[7633.398563068162,7713.839576912529],[7725.842482934665,7713.594953704599],[7779.395315582183,7691.176159539364],[7792.882071445423,7678.290522900031],[7792.956501650993,7678.2261102383845],[7793.036903864899,7678.169327050023],[7793.122499082432,7678.120723498539],[7793.212457984671,7678.080770496281],[7793.305908973623,7678.049855141756],[7793.401946617002,7678.0282769690875],[7793.499640420825,7678.016245045877],[7793.598043844824,7678.013875947583],[7858.651538673917,7679.651302239605],[7874.997038477141,7677.827748395597],[7875.090769787558,7677.821729700949],[7880.493382434283,7677.7290943355565],[7880.590060963036,7677.732115266265],[7880.68599537645,7677.744462422496],[7909.6846674907,7682.91302267052],[7948.147092244313,7679.036619305786],[7948.224965521291,7679.031829923822],[7948.302975168874,7679.033126199565],[7953.631363465809,7679.329879696384],[7961.223520466297,7679.633741138661],[7961.31477009504,7679.641590641567],[7961.4049188625695,7679.657755701254],[7995.299617891985,7687.3526291320095],[8018.686206159207,7685.818700406563],[8018.7879793149805,7685.817216214216],[8018.889376158165,7685.826085163148],[8018.989346219691,7685.845215371238],[8027.055166318707,7687.818950341983],[8027.142102030697,7687.844448905452],[8027.226373211697,7687.877710938025],[8027.307288164296,7687.918463425117],[8027.384182738987,7687.966371870523],[8027.456425785503,7688.021043041978],[8036.607858855003,7695.6223718866195],[8097.833207781465,7725.902888453082],[8104.717276284021,7728.085472273592],[8104.817689738211,7728.123349490486],[8120.748708219526,7735.1308654900895],[8241.185132944402,7700.4770791640385],[8211.43926929139,7550.8073294151955],[8198.818654767392,7533.729760252859]],[[10346.025227111397,7845.144713026245],[10314.949077567273,7877.852340729972],[10291.048206714984,7909.453854286864],[10290.987565852358,7909.526598768903],[10290.920315383735,7909.593280463221],[10290.847058483538,7909.653301296774],[10290.768452198316,7909.7061229382725],[10286.528710208166,7912.272395022219],[10246.251638747854,7960.137933143078],[10242.589005124202,7965.325599395411],[10242.54302013133,7965.385760590907],[10227.649408120733,7983.412872748693],[10227.630086068064,7983.435562769536],[10221.895267027237,7989.970176782728],[10221.83582177499,7990.032307661097],[10221.771258704191,7990.089101973756],[10191.548823464598,8014.452058106277],[10174.594075245688,8033.726687206594],[10167.436269039266,8045.4220159782435],[10167.380960353634,8045.503151006369],[10167.317960993341,8045.578470486572],[10167.24787839466,8045.64724819268],[10167.171388290048,8045.708820973938],[10167.089228192772,8045.762595149066],[10167.002190285844,8045.808052230508],[10166.91111378385,8045.844753923653],[10143.355053177791,8054.01748119638],[10143.293862521352,8054.036537358632],[10137.59428210177,8055.613040855135],[10137.56595750683,8055.620430900449],[10085.662404039731,8068.353965529634],[10061.26198322803,8247.702905276108],[10112.982143830475,8391.129742831561],[10154.086090251698,8490.352949879658],[10280.381842403705,8544.533937376582],[10314.80169276173,8525.011361053288],[10314.886616833532,8524.968399669653],[10314.975240397986,8524.933706121567],[10315.066760722479,8524.907594655504],[10315.160348836187,8524.890301783335],[10315.255157038711,8524.88198414007],[10315.350326578357,8524.882717065073],[10315.444995430516,8524.892493919675],[10315.538306105702,8524.911226147295],[10315.629413416491,8524.938744075562],[10315.717492133046,8524.974798453179],[10315.801744457865,8525.01906270758],[10315.881407252058,8525.07113590296],[10315.955758947679,8525.130546371847],[10316.024126083546,8525.196755987377],[10316.085889405287,8525.26916503752],[10316.140489474417,8525.347117657146],[10339.753745749662,8562.727186575506],[10510.37597635501,8519.045725460672],[10512.922373802272,8515.896273114453],[10512.993825047399,8515.81696261658],[10540.16309020782,8488.719163847516],[10561.869801770457,8406.73951856519],[10595.78457355295,8246.910169568035],[10582.253653611035,8217.374636542912],[10582.242479252885,8217.34932599246],[10577.300096718476,8205.721798702474],[10577.269873052759,8205.641229378518],[10577.246687991112,8205.558359970117],[10564.938627544938,8152.93607043925],[10563.28118330295,8149.321756384675],[10563.242122677219,8149.223065443012],[10563.213742337732,8149.1207903689265],[10563.196362007022,8149.016083356014],[10563.19017748554,8148.910123995207],[10563.195258445845,8148.80410598593],[10571.76151796755,8064.264421687878],[10571.08696911783,8039.513841682362],[10571.086878534878,8039.510285798583],[10569.72347735348,7981.969478387166],[10566.49207458235,7969.314838712089],[10462.538070373219,7849.654075305409],[10346.025227111397,7845.144713026245]],[[7426.670386675704,11197.286594261455],[7568.835222645126,11197.47686039972],[7568.85976847922,11197.477194555695],[7577.653156908973,11197.704880506108],[7577.749101551719,11197.711994328642],[7577.843918549188,11197.728295185483],[7577.936730277058,11197.753632196209],[7578.026677671744,11197.787770841933],[7578.112928181877,11197.830395136],[7652.481580613324,11239.147707431845],[7754.143250234301,11225.535695441737],[7780.701946130396,11203.813841670091],[7801.3006150494475,11171.33176256561],[7815.833005877505,11143.737385805107],[7821.893765651645,11130.896268560533],[7831.802516210193,11098.009290703101],[7831.830576287936,11097.928763464433],[7831.865395138753,11097.850919699376],[7831.906719559816,11097.77632548811],[7835.368736650841,11092.111240635888],[7850.542106729376,11061.793355089354],[7850.585788955557,11061.715054821769],[7850.636309010106,11061.640981932826],[7850.69326075883,11061.571731899574],[7850.756186362702,11061.507861427795],[7850.824579958466,11061.449883976606],[7864.213637297741,11051.094704862184],[7891.251153557959,11010.15628671483],[7781.132307216056,10872.932398510777],[7745.240484230973,10875.065976290483],[7694.508442082907,10905.637276843603],[7694.435034110395,10905.677425975979],[7694.358526750459,10905.71129789849],[7674.192306014672,10913.647919972067],[7674.065383321157,10913.688337878508],[7649.918748419431,10919.639439724844],[7649.820878890481,10919.658441861551],[7649.721603645836,10919.667604377411],[7649.62190943436,10919.66683620136],[7649.5227871692505,10919.656144968703],[7649.425222078827,10919.635636945226],[7649.330183913834,10919.605515970956],[7649.238617308572,10919.566081434099],[7649.151432391686,10919.517725295254],[7649.069495739916,10919.460928191518],[7648.993621764734,10919.39625465917],[7648.924564617479,10919.324347522464],[7648.863010693456,10919.245921504245],[7646.369219801957,10915.71988400056],[7598.782973261464,10880.325119026362],[7469.54632668648,10887.767751202317],[7418.606102703506,10973.677597323976],[7418.554143364413,10973.75647921865],[7418.494973185938,10973.830106769235],[7418.429120091881,10973.897823061436],[7418.357171631858,10973.959023921941],[7418.279769739114,10974.013163308951],[7410.521578895209,10978.910901863834],[7410.4292658540535,10978.962562081391],[7410.3320120975795,10979.004181577993],[7410.230905941582,10979.035294611675],[7410.1270788120355,10979.055553012791],[7410.021692583906,10979.0647300802],[7409.915926579212,10979.062723118148],[7367.226756600875,10975.988482320658],[7250.386764580707,11017.110262952589],[7275.236417609182,11193.473760542127],[7279.632194011116,11196.926928117546],[7323.252697050704,11218.61182895883],[7418.88215472422,11196.100799035577],[7418.978471422696,11196.083053225408],[7419.076062096176,11196.074814254991],[7419.173990672471,11196.076161151053],[7419.271317838283,11196.08708099441],[7426.670386675704,11197.286594261455]]]]}'
[ ]: